seek() Function in Python
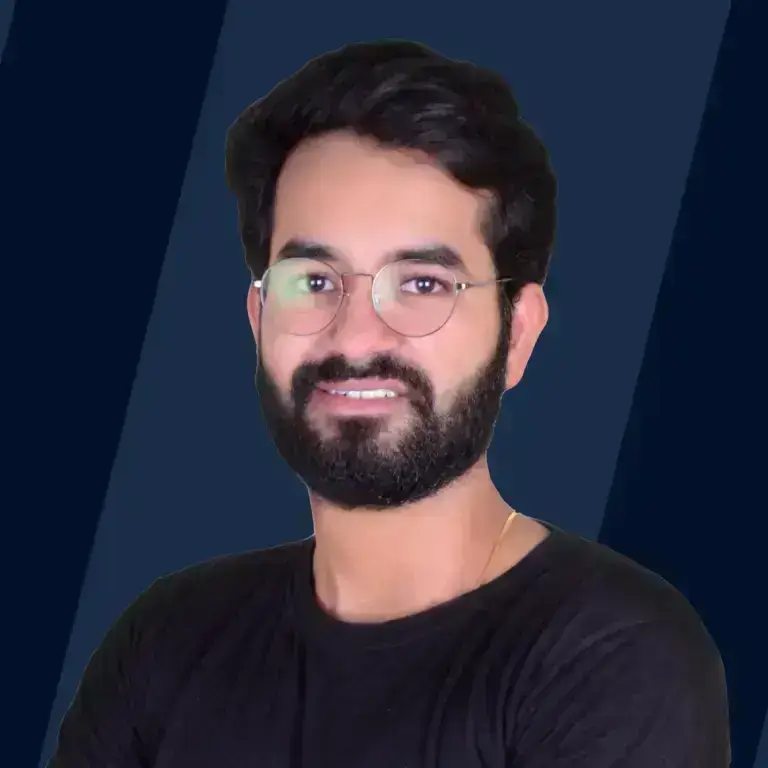
Overview
File handling is an important concept of programming. It allows us to save information for permanent memory. File handling in python provides great features along with ease of programming.
The seek() function in python is one of these great features. seek() in python allows us to manipulate the file handle such that various parts of the file can be accessed at random.
The seek() function in python is complemented by the tell() function that gives the current position of the file handle.
Syntax of seek() in Python
The seek() function is a class method available inside a file pointer object. To call seek() in python, we need to first declare a file pointer by opening a file.
The syntax of "seek()" in python is :
Parameters of seek() in Python
As seen in the above syntax the seek() function in python takes two parameters :
- offset : required, the number of characters to move the file handle.
- from_where : optional, the reference point from which the file handle is moved relatively.
The offset parameter tells the number of characters to move the file handle. It is an integer number that makes the file cursor move by the given number of characters (or bytes, if the file is opened in binary mode)
Example usage of offset :
The from_where parameter is the reference point from where the file cursor will be moved.
This argument is optional and takes either of three values :
- os.SEEK_SET (or 0) :
default, offset gives absolute position of file cursor. - os.SEEK_CUR (or 1) :
offset gives the new position that is relative to the current position of the file pointer. - os.SEEK_END (or 2) :
offset gives the position that is relative to the file's end position.
Example usage of from_where :
Note :
That the relative referencing (os.SEEK_CUR and os.SEEK_END) requires the file to be opened in binary mode.
Return Value of seek() in Python
The seek() function in python returns an integer value representing the new position of the file cursor.
Return Type : integer
Example : The Following Program Shows the Return Value Provided by seek()
Contents of the sample.txt file are :
Output :
Exceptions of seek() in Python
The seek method can cause the exception io.UnsupportedOperation. This exception occurs when we try to seek relative to the current or end when the file is opened in non-binary mode.
Binary Mode :
In this mode, the file is read bit by bit, this mode is used to read special binary files which are , not human-understandable.
Non-Binary Mode :
It is also known as character mode, where a file is read one character at a time. These files are human-readable.
Example : Demonstrating Exceptions of seek() in Python
Note :
- That the seek(765) will not throw an exception even if the file length is less than 765.
- All further read-write operations will be done from this new file cursor position itself.
- For example, readline() after the seek(765) will return an empty string ('').
Example of seek() in Python
Example : The Following Code Snippet Demonstrates the Basic Usage of seek() Function in Python
Contents of the "sample.txt" file are :
Output :
What is seek() in Python ?
Up until now, we have seen various bits that make up the seek() function. Now, let us have a look at seek() as a whole.
The seek() function in python allows us to access various parts of an opened file according to our requirements. This enables us to perform read-write operations at random.
seek() takes a mandatory argument offset and an optional argument from_where, and returns the new position of the file cursor. It is useful for file-handling operations as it gives us the ability to jump to a specific portion of the file.
A file handle needs to be opened on which the seek() function can operate. If the file handle is opened in append write mode ('aw') then any operations done using seek() are useless.
In the big picture, the seek function manipulates the file cursor. This cursor then affects the read and write positions of that particular file operation.
More Examples
For the rest of the examples we are going to use the "sample.txt" file with the contents :
Example - 1 : Seeking Forward Through the Line and then Reading the Rest of the File
Output :
Explanation :
When we open the file, we have not provided any other arguments after the file name. Hence the file is opened in non-binary (or character) mode. In the second line of code, we seek ahead 5 characters of the file and read the rest of the file. The initial 5 characters of file "This " are not seen in the output.
Example - 2 : The Use of Negative Offset Values
Output :
Note :
That the read() function returns a byte array (b'') instead of a string since we have opened the file in binary mode ('rb')
Explanation :
For using the negative offset value we need to open the file in binary mode. Use os.SEEK_END as the reference point for the offset as the default reference point is the start of the file, and negative values from the start of the file are not allowed. We can also see that the return type from the read() function is a byte array when the file is opened in binary mode.
Example - 3 : Reading the Width of a Png File
Output :
Explanation :
In this, we use seek() to read the height and width of a .png image file. The urllib library is used to download the google.png file.
The real magic happens when we call seek(16). For any .png image file, it is defined in the structure that the width of an image is a 4-byte integer located at the 16th-byte offset of the .png file. Also, the height of the image is given as a 4-byte integer on the 20th-byte offset of the .png file.
We have used the struct library to convert the binary value of a 4-byte integer to the integer value of a python variable.
Conclusion
In this article, we have understood the working of seek() in python. Along with the following concepts :
- seek() allows us to jump to any portion of the file at random
- seek() takes two arguments, offset and from_where
- offset is a required argument, an integer specifying where to make the jump.
- from_where is an optional argument, an enum specifying the reference point for the offset.
- seek() returns the new position of the file cursor.
- seek() may throw an io.UnsupportedOperation exception if the file is not opened in binary mode and os.SEEK_CUR or os.SEEK_END reference points are used.
- seek() allows the offset values to be negative for os.SEEK_CUR and os.SEEK_END reference points.
- tell() function is complementary to the seek() function in python.
See Also (Related Functions)
Visit the following topics to learn more about file handling in python :