Program to Find Square and Square Root in Java
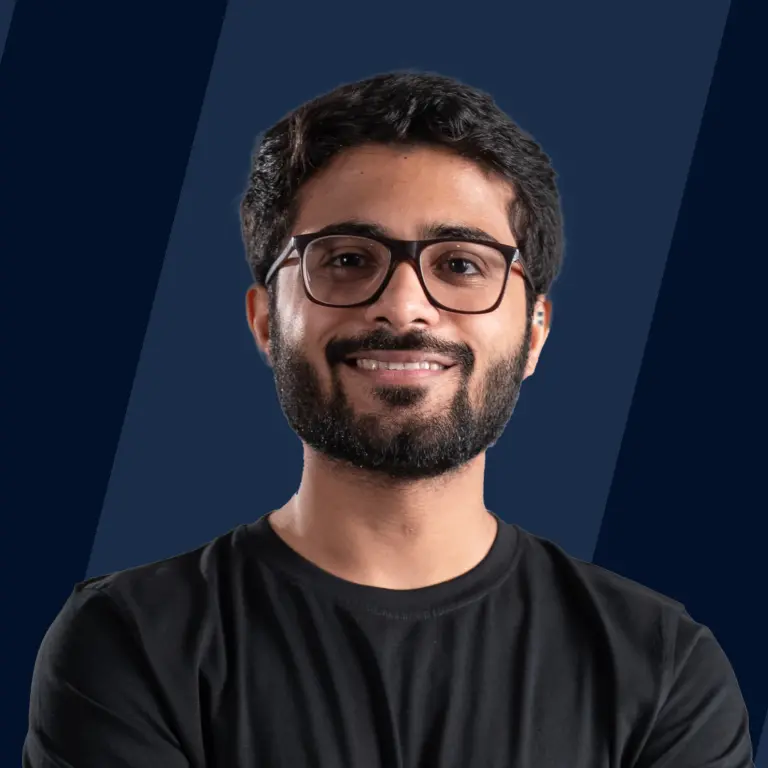
Overview
Squares are the numbers generated when a value is multiplied by itself.
In contrast, a number's square root is a value that, when multiplied by itself, returns the original value. Both are therefore vice-versa methods.
For example, the square of is and the square root of is .
In Java Math. sqrt() returns the square root of a value of type double passed to it as argument.(There are other ways as well)
As a programmer, I always find it fascinating to write programs for mathematical operations that I used to do by hand back in school.
Square and square root of a number is one of the principles which are used in many real-life applications as well as programming concepts. For example prime factors of a number, binary exponentiation etc.
Let’s start by defining what a square and square root actually means.
What is Square?
Simply put, the square of a number is the number multiplied by itself.
Square of
For example, if
Square of
What is Square Root?
Square root is exactly the opposite of the square of a number. The square root of a number X is the number that when multiplied by itself equals X.
Square root of X = √X
where √ is the symbol for square root
For example, if X = 9
Square root of 9 = √9 = 3
The square root of X can also be represented by .
In a nutshell, if the square of a number X is S, then X is the square root of S.
Now that we know what we mean by the square and square root of a number, let’s see how we can write a program for square root in java.
How to square a number in Java?
There are a couple of ways to find the square of a number in Java. Let’s look at them one by one.
1. Multiplying the number by itself
It’s as simple as it sounds. Just multiply the number by itself and you’re good to go.
2. Using Math.pow Method
Math is a utility class in Java that has many useful mathematical functions, one of which is pow which allows you to calculate the exponentiation or power of a number.
Syntax:
Parameters : base, exponent Returns: base to the power of exponent
Both parameters accept double as the input. The return type is also a double value.
If you pass an integer or any primitive numerical value to either base or exponent, it will automatically be cast to double in Java.
So, if base = 10, exponent = 2 Math.pow(10, 2) = 100.0
So, in order to find the square of a number, we can pass the base as the number and the exponent as 2. Here’s a snippet that shows the usage of Math.pow.
How to find Square Root in Java?
Calculating the square of a number was pretty straightforward right. But calculating the Square Root in Java is a bit more interesting. Let’s see how we can do that.
1. Square Root in Java Using Math.sqrt()
This is the friendly sibling of the Math.pow function we saw in the previous segment. Like the way it reads, it finds the square root of a number.
Syntax
Parameters : number Returns: The square root of number
The number parameter accepts double as input and the return type is also a double value.
So, if number = 9 Math.sqrt(9) = 3.0
Here’s a snippet that demonstrates the use of Math.sqrt()
2. Square Root in Java Using Math.pow()
Just like we used Math.pow to find the square of a number, we can use it to find the square root of a number as well.
But how?
Remember the way the square root of a number is represented: ; it means the square root of a number is X to the power of ½.
So if we pass the value of the exponent in Math.pow as which is KaTeX parse error: Expected 'EOF', got '½' at position 1: ½̲, the resulting number will be the square root. Let’s try that in the below snippet.
Neat right? The same function is used to calculate two different operations.
3. Square Root in Java Without using any inbuilt function
Now that we have looked at a few ways to find the square root of a number using inbuilt functions in Java, let’s look at a way without using any such inbuilt function.
Let me propose an algorithm first, and then we’ll break it down one step at a time.
-
Start from , if , then i is the square root of n as n is a perfect square.
-
if , it means the square root must lie between , let’s call them .
-
Apply binary search in the range . Find of :
-
if , it means we assume that is the square root of .
-
if , it means that the assumption we made is incorrect. This implies, the square root of n must be less than mid since any value higher than mid can never satisfy the condition . Hence, we will try to find the square root on the left side of mid by repeating step 3 for .
-
Otherwise, means that our assumption is incorrect. It implies the square root of must be greater than mid since any value less than mid can never satisfy the condition . Hence, we will try to find the square root on the right side of by repeating step 3 for .
What is Perfect Square?
A perfect square is an integer that is the square of an integer. For example,
Here, 9 is a perfect square because is the square of . On the other hand, is not a perfect square because it cannot be represented as the square of an integer.
So, if we want to calculate the square root of , which is a perfect square, we need to find a number that, when multiplied by itself, equals .
Numbers that are not a perfect square will have a real number (decimal) as their square root. So, we need a way to find the nearest approximate value for the square root (up to 6 decimal places for now). It’ll be something like: R.abcdef
Let’s say we need to find the square root of , which is not a perfect square. Let’s find R first.
Observe that the maximum perfect square less than is whose square root is . Since the square of is , which is greater than , the square root of has to be less than . So we can be sure that the square root of as well will be something like .
Now that we have the value of let’s find abcdef.
In order to find the decimal places, we can use Binary Search. We know that the square root will lie between (, ). We apply binary search in this range to find the value whose square is equal to (up to decimal places). Here is a sample walkthrough of how we are applying binary search.
Hence, the square root of
Here is a snippet of this algorithm
Conclusion
- Squares are the numbers generated after multiplying a value by itself. In contrast, the square root of a number is a value which, on getting multiplied by itself, gives the original value.
- We can square a number in Java in two different ways, multiplying the number by itself and calling the Math.pow function.
- We can find sqaure root in Java by using Math.sqrt function, using Math.pow function, or calculating it using a binary search for the given range.
- A perfect square is an integer that is the square of an integer.
Exercise
- What will happen if a negative number is passed as the exponent parameter to the Math.pow function?
- What will happen if a negative number is passed to the Math.sqrt function?
- Can you think of improving the time complexity of the binary search algorithm we used above to find the square root of a number?