Calculate Square Root in Python
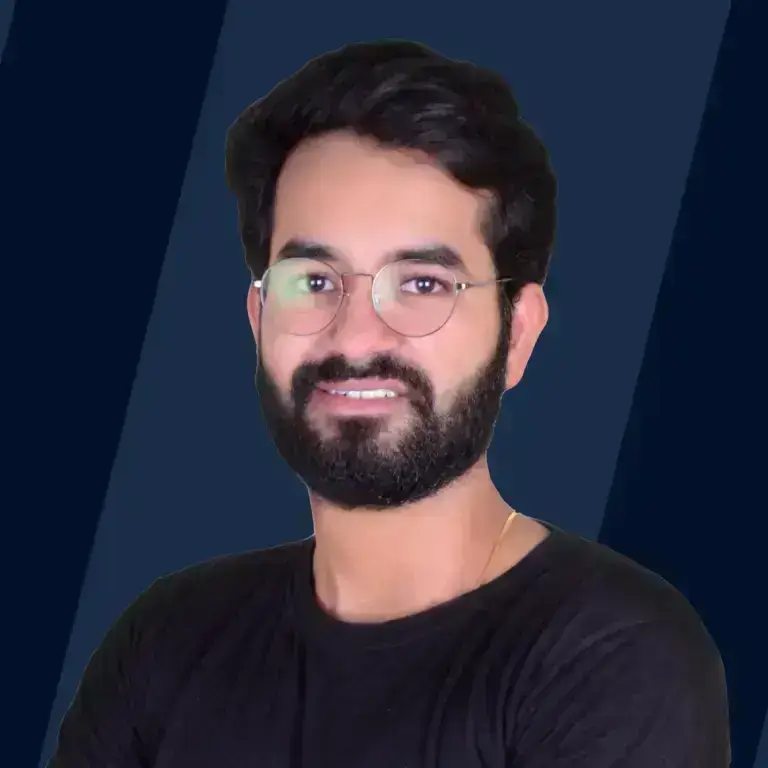
In Python, calculating the square root of any number is a common operation in various mathematical and scientific computations. In this article, we will explore three different methods to compute how to find a square root in Python, namely using math.sqrt(), numpy.sqrt(), and the exponentiation operator **.
Square Root in Python Using math.sqrt()
Let's learn how to find square root in python.
For using the square function in python (sqrt()) we need to import the inbuilt math library into our project.
The sqrt() in Python has the following syntax:
Where a is the number whose square root has to be calculated.
We need to import the math library into our project to find square roots in Python using sqrt(). For importing the math library, we use the following line of code:
We pass a number whose square root is to be calculated as a parameter into the sqrt().
Example
Now calculate the square root of number 81 using the sqrt() function of the math library in python.
Code:
Output
Explanation:
- We imported the math library, which has sqrt().
- We stored the number whose square root will be calculated into a variable.
- We stored the result of sqrt() into a variable.
- Finally, we print the variable to get the square root of the number entered.
The square root function in Python takes a number as its parameter, which should always be non-negative(number 0 & all positive numbers, including floating numbers). Passing a negative number as a parameter will cause a math domain error because a complex number is not supported in Python.
The square root function in Python returns a float type value for every kind of non-negative number passed as its parameter.
For example, if a perfect square number like 25 is passed in it, it will return 5.0, while on the other hand, if 50 is passed in it, it will return 7.07.
Square Root in Python Using numpy.sqrt()
Let's learn how to take square root in python using numpy.sqrt().
The NumPy library stands for Numerical Python. The numpy.sqrt() function is a part of the NumPy library, which is widely used for numerical computing in Python. It is useful when working with arrays or matrices of numbers.
Explanation
The numpy.sqrt() function takes a single argument, a scalar value, an array, or a matrix containing numbers and returns the square root of each element in the input. It operates element-wise on the input array or matrix, meaning it calculates the square root of each element separately.
Example:
Let's explore how to use numpy.sqrt() with some examples:
Output:
In Example 1, we compute the square root of a single number, 25, using np.sqrt(). The result is 5.0.
In Example 2, we compute the square root of each element in an array [4, 9, 16, 25] using np.sqrt(). The result is an array [2., 3., 4., 5.], where each element is the square root of the elements given in the input array.
In Example 3, we compute the square root of each element in a 2x3 matrix using np.sqrt(). The result is a matrix where each element is the square root of the elements given in the input matrix.
Square Root in Python Using The Exponentiation Operator **
Let's learn how to find square root in python using the Exponentiation operator. This method is simple and does not require importing any external libraries. Here's how to use it:
Explanation To find the square root of a number in python using the exponentiation operator, raise the number to the power of 0.5.
Example:
Output:
Conclusion
- The math.sqrt() function from the standard math module is used for simple and efficient square root calculation.
- The numpy.sqrt() function from the NumPy library for working with arrays or matrices of numbers.
- Exponentiation Operator ** is used for simple and quick calculations of square roots without the need for additional libraries.