JavaScript String startsWith()
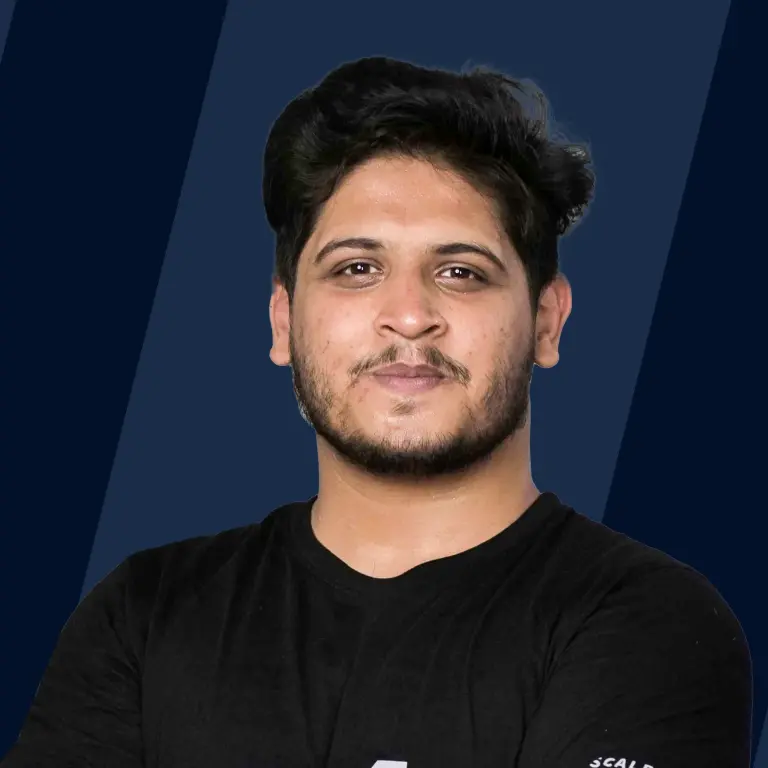
The startsWith() method in JavaScript is designed to determine if a string begins with specified characters.
Syntax of String startsWith() in Javascript
Here, the string is the string to be checked. It returns true when the string matches the searchString from the given position, and false otherwise.
Parameters of Javascript String startsWith()
The startsWith() method takes two parameters: searchString and position.
- searchString is a character or the set of characters that must be searched in the string.
- position is an optional parameter. The position determines the index from where the string should be searched
Return Value of String startsWith() in Javascript
Return Type: boolean
The startsWith() method in JavaScript returns either true or false.
- true is returned by the startsWith() method if the string to be checked is found in the search string.
- false is returned by the startsWith() method if the string to be checked doesn't exist in the search string.
Exceptions of Javascript String startsWith()
The startswith javascript method works only with strings i.e, if we try to use the startsWith() method to find an integer, we will get an error.
Example:
Output:
Explanation:
In the above example, we get an error because startsWith() works with string. But here myString has an integer value. Therefore, we get an error.
Example of String startsWith() in Javascript
Code:
Output:
Explanation:
In the above example, we used the startsWith() method to check whether string starts with searchString.
The JavaScript startsWith() method, introduced in ES6, checks if a string begins with a specified character or substring. It accepts two parameters:
- searchString to define the starting sequence
- An optional position to specify the starting index for the search.
This method returns true or false.
Note : The startsWith() method in JavaScript is case sensitive, i.e, uppercase and lowercase are considered distinct. For example, scaler and Scaler both are different because the first letter i.e, "S" is in lower case in the first instance and the second instance it is in the upper case.
Polyfill
The startsWith() may not be available in all the JavaScript implementations. You can use the startsWith() method using polyfill String.prototype.startsWith() with the following code snippet.
More Examples of Javascript String startsWith()
Example 1
Passing a searchString as parameter to the startsWith() method.
Code:
Output:
Explanation:
In the above example, We declared the myString variable. With the help of the startsWith() method, we will try to find out whether the word passed as a parameter to the startsWith() method exists in the myString variable.
Example 2
Passing a searchString and position as parameter to the startsWith() method.
Code:
Output:
Explanation:
As we discussed earlier, the startsWith() method takes position also as a parameter. In the above example, the startsWith() method checks whether Hello is present in myString from the second character in the string.
Example 3
Code:
Output:
Explanation:
In the above example, we passed javascript as a parameter and the variable myString has Hello world this is JavaScript stored in it. Even though javascript is present in myString, The startsWith() returns false because startsWith() method is case sensitive.
Browser Compatibility
The startsWith() method is widely supported across modern browsers, but it's important to note its compatibility for older versions:
- Chrome: Supported from version 41.
- Firefox: Available since version 17.
- Safari: Introduced in version 9.
- Edge: Supported in all versions.
- Internet Explorer: Not supported.
- Opera: Available from version 28.
For Internet Explorer or other older browsers, consider using a polyfill or alternative methods to ensure functionality.
Conclusion
- The startsWith() method in javascript checks whether a given character or string is present in a string.
- The startsWith() method in javascript returns true if the string is found, or the method will return false.
- The method takes two parameters, searchString and position(optional). searchString is the string to be checked and position is the index from where the method has to check.
- The startsWith() method in javascript is case sensitive and is valid only for characters or strings.