Static Keyword in Java
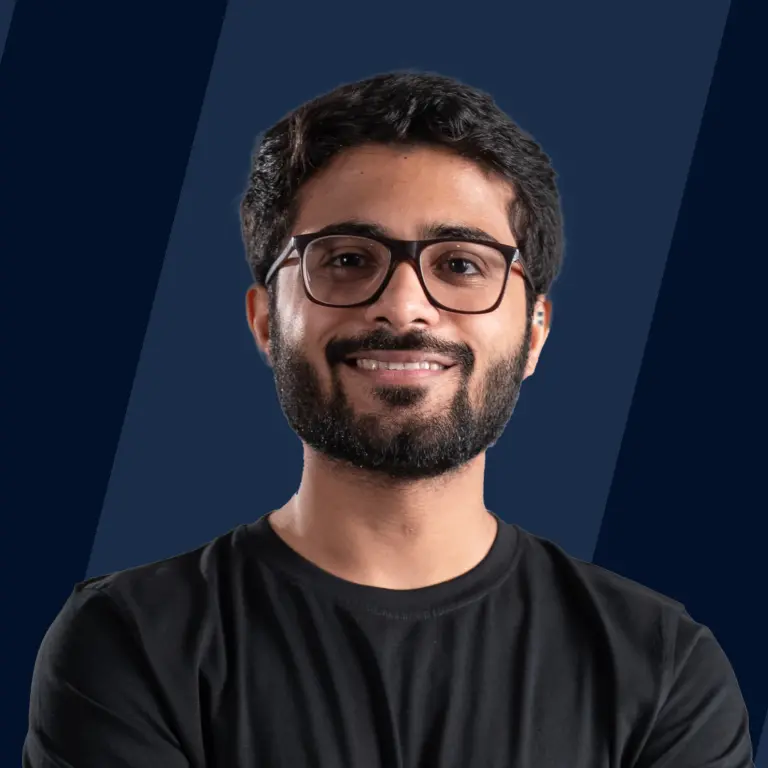
Overview
The static keyword is mostly used in Java for efficient memory management of variables and methods. We can apply a static keyword to variables, blocks, methods, and nested (inner) classes in a Java program.
What is a Static Keyword in Java?
In Java, there are several reserved keywords for a specific use, we cannot use these pre-defined keywords as variable names or identifiers in a Java program.
The static keyword in Java is one most commonly used keywords. The most fundamental reason for the widespread usage of static keyword in Java is to efficiently manage our program memory.
In general, we first have to make an instance/object of a class in order to access variables or methods declared and defined inside the class. However, there may be times when you simply need to access a few of a class's methods or variables and don't want to create a new instance of the class merely to access these members. This is when Java's static keyword comes in handy.
The Anatomy of the static keyword in Java
The term static in the Java programming language denotes that a certain member belongs to a type rather than to a particular instance of that type.
Let's understand this with a real-life example, we know that in India a particular branch of a bank has a common IFSC_CODE for all their account holders. Here, we can make the IFSC_CODE a static member variable of the BankAccount class, so that the IFSC_CODE variable will belong to the branch of the bank (a class) with equal value for all bank account holders (all instances) rather than to a single bank account holder (a particular instance) of this class.
We can understand from the above image that the IFSC_CODE variable has the same value for all the instances of BankAccount class, i.e., object 1, object 2, ..., object n. This indicates that when we create one static member, it will be shared by all instances/objects of that class.
Let's see more about the static variables in general, then we will see an example Java program related to the above explanation.
static Variable in Java
Static variables are fundamentally considered as global variables. When we declare a static variable in a class, a single copy of that variable is made at the class level and is shared across all of its objects.
Some important points related to static variables:
- Static local variables are not permitted in a Java program, we can only create static member variables at the class level.
- In a Java program, static blocks and static variables are executed in the order in which they appear.
To get a more detailed explanation, visit this link Static Variables in Java.
Syntax
Example Java Program
Output:
Explanation:
- We have created a BankAccount class with a static variable IFSC_CODE and five instance member variables, accountNumber, balance, customerName, email, and phoneNumber.
- In the main() function, we have created two instances/objects A1 and A2 of the BankAccount type.
- We can interpret from the output that the IFSC_CODE static variable has the same value for all the instances/objects of the BankAccount class.
- We can access and manipulate the static class variables using the class name (BankAccount) and also with the instances/objects of that particular class.
Java Static Blocks
When we use the static keyword with a block, we call that block a static block.
Some important points related to static blocks:
- Static blocks are used to set the static member variable's initial value.
- Static blocks are called before the main function.
- It was possible to execute the code of a static block without using the main function while using Java version 1.6 or below versions. It will throw an error with the latest versions of Java.
Syntax
Java static block declaration:
Example Java Program
Output:
Explanation:
- In the above Java program, we have created two static integer variables radius and area.
- Also, we have created a static block outside the main() function, in which we have initialized the radius and area static variables.
- We can see from the output that the static block is executed before the main() function.
Static Method in Java
The method is known as a static method if it is declared using the static keyword. The main() method in a Java program is the most typical example of a static method.
Some important points related to static methods:
- Any static member of a class can be accessed before any objects of that class are created with the use of the class name.
- A static method can view and edit the value of a static member variable.
Syntax
Example Java Program
Output:
Explanation:
- We have created an Employee class with two static variables companyName and count, two instance member variables employeeId and employeeName, we have also created two static methods setEmployeeId() and setCompanyName().
- In the main() function, we have set the company name to Alphabet using the setCompanyName static method, now it will be shared with all the instances of the Employee class.
- We have created three instances/objects e1, e2, and e3 of the Employee type.
- We can interpret from the output that companyName is the same for all the instances/objects, and employeeID increases consecutively.
- We can access and manipulate the static method using the class name (Employee) and also with the instances/objects of that particular class.
Restrictions for the Static Method
Static methods are subject to the following restrictions:
- Non-static data members or non-static methods cannot be used by static methods, and static methods cannot call non-static methods directly.
- In a static method, this and super keywords cannot be utilized. It will throw an error as follows:
Java Static Nested (inner) Classes
In Java, a class can be declared within another class and it is known as a nested class. We can't use static keywords with the top-level outer class, but we can use static keywords with nested classes. So, the nested class declared with a static keyword is known as a static nested class.
Some important points related to static nested class:
- We can instantiate a static nested class, even if we have not created an instance of its outer class first.
- Static nested classes can't access the outer class's non-static members, it can only access the static member variable of the class.
Syntax
Example Java Program
Output:
Explanation:
- We have created a staticNestedClass in the Main class itself.
- We have defined a display() function in the static nested class which uses the outer static variable programmingLanguage.
- In the main() function, we have instantiated an object of staticNestedClass type and used the display() method to get an output JAVA.
Static vs Non-Static
Let's talk about a few differences between static and non-static variables and methods in Java before continuing with this Static in Java tutorial to examine static blocks and classes.
Static Variables | Non-Static Variables |
---|---|
The class names can be used to access them. | Objects can be used to access them. |
Both static and non-static methods can be used to access them. | Only non-static methods can be used to access them. |
Only one memory allocation is made for them when the class is loaded. | Memory is allotted for each object. |
All objects or instances of the class share these variables. | Each object has its own copy of the non-static variables. |
Static variables have global scope. | Non-Static variables have local scope. |
Static Methods | Non-Static Methods |
---|---|
Early or compile-time binding is supported by these methods. | They can support run-time, dynamic, or late binding. |
Only the static variables of their own class and other classes can be accessed by these methods. | Both static and non-static members are accessible to them. |
Static method overrides are not permitted. | They can be overridden. |
Less memory is used since just one memory allocation is made when the class is loaded. | Each object has its own allotted memory. |
Conclusion
- static keyword in Java is mostly used for efficient memory management.
- The static keyword in Java can be used with variables, methods, blocks, and nested (inner) classes in a Java program.
- When we create one static member it will be shared by all instances/objects of the class with equal value.
- Static local variables will give an error in Java, we can only create static member variables at the class level.