strcmp() in C++
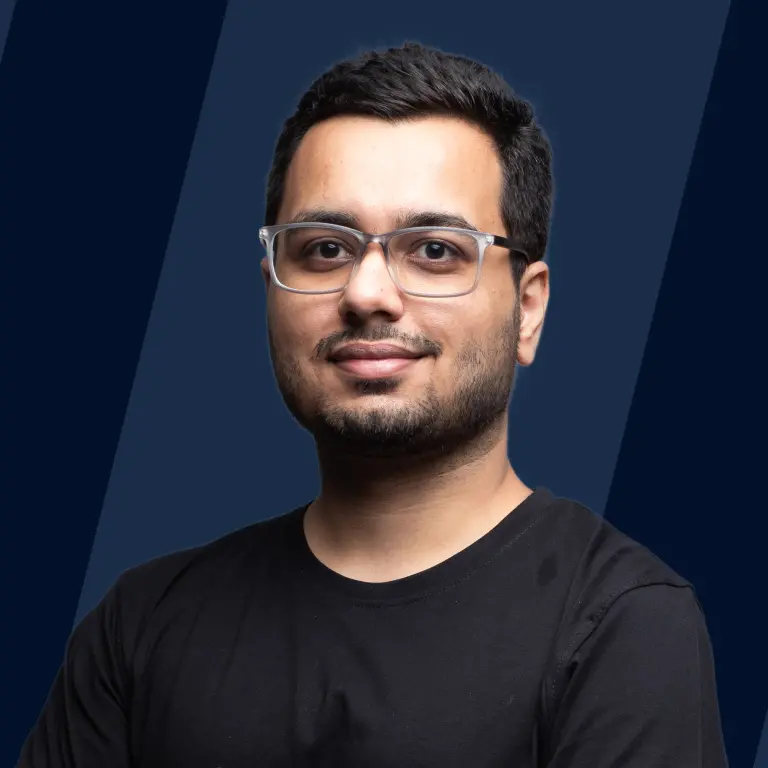
Overview
The strcmp() function is a built-in library function in C++ that compares two character arrays or null terminating strings (C-strings) lexicographically. The strcmp() function takes the two character arrays as parameters that must be compared. The strcmp() function in C++ returns an integer value calculated according to the first mismatched character among the two strings.
What is strcmp() in C++ ?
The function strcmp() is a built-in library function of C++, which is prototyped and defined in the <cstring> header file. This function takes strings as arguments and compares them to check equality. It matches strings lexicographically, which implies that it matches every character for every index in both strings. The comparison begins from the first character of strings and carries on for every character until any unmatched character or NULL character gets encountered.
Syntax of strcmp() in C++
The syntax for using strcmp() function in C++ is:
Where s1 and s2 are the names of strings.
Parameters of strcmp() in C++
The strcmp() function contains two parameters, s1 and s2, that are pointers to the character arrays required to be compared.
Return Value of strcmp() in C++
The strcmp() function in C++ returns an integer value calculated according to the first mismatched character among the two strings. There are three types of return values generated by the strcmp() function.
- Zero (0): The return value is equal to zero if both strings are the same. That is, elements at the same index in both strings are equivalent.
Example:
Output:
Explanation:
In the above code, two strings, s1 and s2, are declared and initialized, respectively. They are then passed through the strcmp() function. The function's return value gets stored in the variable returnvalue. A conditional statement is used to check whether strings are the same by verifying whether returnvalue is equal to zero.
- Greater than zero (> 0): The return value is greater than zero if the ASCII value of the first unmatched character of the string on the left-hand side (s1) is greater than that of the corresponding character in the right-hand side string (s2). The resultant value is the difference between the ASCII values of the first unmatched characters of the strings. i.e., (s1-s2).
Example:
Output:
Explanation:
In the above code, two strings, s1 and s2, are declared and initialized, respectively. They are then passed through strcmp() function. The function's return value gets stored in the variable returnvalue. The first unmatched character in the strings is found at index 0, where characters in both the strings are ‘s’ and ‘S’, respectively. The ASCII values of both characters are 115 and 83, respectively. Hence, the difference in the ASCII value turns out as 32. A conditional statement is used to check whether strings are the same by verifying whether returnvalue is equal to zero.
- Less than zero ( <0 ): The return value is less than zero if the ASCII value of the first unmatched character of the string on the left-hand side (s1) is less than that of the corresponding character in the right-hand side string (s2). The resultant value is the difference between the ASCII values of the first unmatched characters of the strings. i.e., (s1-s2).
Example:
Output:
Explanation:
In the above code, the first unmatched character in the strings is found at index 0, where characters in both the strings are S and s, respectively. The ASCII values of both characters are 83 and 115, respectively. Hence, the difference in the ASCII value turns out as -32.
strcmp() Prototype
The prototype of the strcmp() function in C++ is described in the cstring header file is:
- The strcmp() matches the elements of strings s1 and s2 lexicographically.
- The return value is the difference between the first unmatched pairs of characters that occur in s1 and s2.
strcmp() Undefined Behavior
The strcmp() function in C++ shows undefined behavior or exceptions if one of the parameters does not point to C++ character arrays or null-terminated strings.
Example:
Compile Errors:
Output:
Explanation:
In the above code, two strings, not null-terminated, s1 and s2, are declared and initialized. The strings are passed as parameters to the function strcmp(). The function throws a compile-time error showing undefined behavior.
Implementation of strcmp() Function Using an User-Defined Function
Output:
Explanation: In the above code, two strings, s1 and s2, are declared and initialized, respectively. They are then passed through a user-defined function. In the user-defined function, the strcmp() function is called, and the function's return value gets stored in the variable returnvalue. A conditional statement is used to check whether strings are the same by verifying whether returnvalue is equal to zero.
Conclusion
- The function strcmp() is a built-in library function of C++, prototyped and defined in the “string.h” header file.
- The strcmp() function takes the two character arrays as parameters that must be compared.
- The strcmp() function in C++ returns an integer value calculated according to the first mismatched character among the two strings.
- The strcmp() function in C++ shows undefined behavior if one of the parameters does not point to C character arrays or null-terminated strings.