Strings in C++
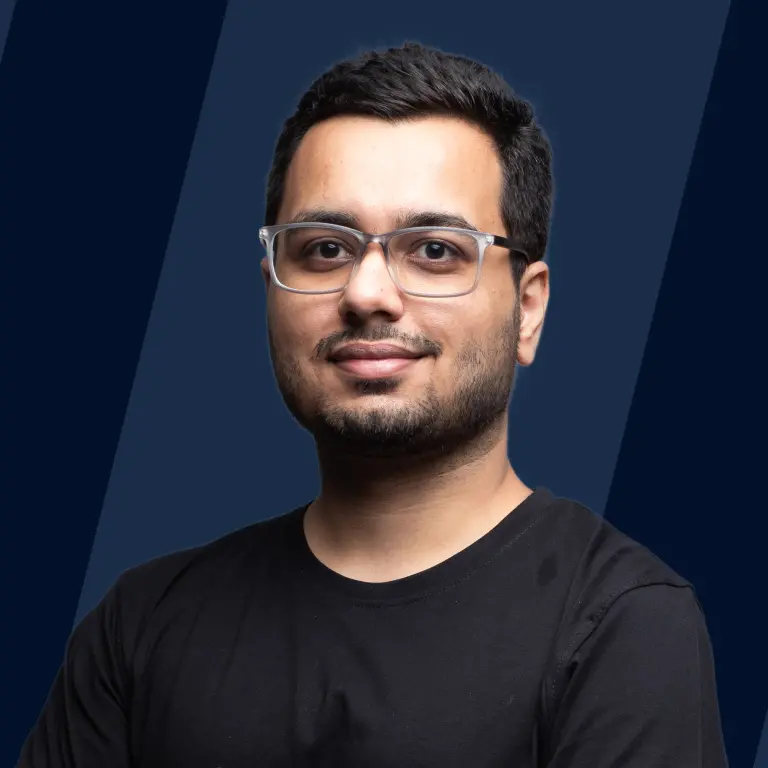
A string in C++ is an object that represents a sequence of characters. On the other hand, a string in C is represented by an array of characters. C++ supports C-style strings as well. The string objects in C++ are more frequently used than the character arrays because the string objects support a wide range of built-in functions.
String in C++
A string in C++ is a type of object representing a collection (or sequence) of different characters. Strings in C++ are a part of the standard string class (std::string). The string class stores the characters of a string as a collection of bytes in contiguous memory locations. Strings are most commonly used in the programs where we need to work with texts. We can perform various operations on the strings in C++. For example, reversing, concatenating, or passing as an argument to a function.
Syntax
The syntax to create a string in C++ is quite simple. We use the string keyword to create a string in C++. However, we also need to include the standard string class in our program before using this keyword.
Besides the method described above, C++ strings can be created in many different ways. The alternative ways of defining a string are explained in the upcoming sections. Before that, let us briefly introduce the C Style Character Strings.
String vs. Character Array
C++ supports both strings and character arrays. Although both help us store data in text form, strings, and character arrays have a lot of differences. Both of them have different use cases. C++ strings are more commonly used because they can be used with standard operators while character arrays can not. Let us see the other differences between the two.
Comparison | String | Character Array |
---|---|---|
Definition | String is a C++ class while the string variables are the objects of this class | Character array is a collection of variables with the data type char. |
Syntax | string string_name; | char arrayname[array_size]; |
Access Speed | Slow | Fast |
Indexing | To access a particular character, we use "str_name.charAt(index)" or "str[index]". | A character can be accessed by its index in the character array. |
Operators | Standard C++ operators can be applied. | Standard C++ Operators can not be applied. |
Memory Allocation | Memory is allocated dynamically. More memory can be allocated at run time. | Memory is allocated statically. More memory can not be allocated at run time. |
Array Decay | Array decay (loss of the type and dimensions of an array) is impossible. | Array decay might occur. |
The C-Style Character String
In the C programming language, a string is defined as an array of characters that ends with a null or termination character (\0). The termination is important because it tells the compiler where the string ends. C++ also supports C-style strings.
Syntax
We can create the C-style strings just like we create an integer array. Here is its syntax.
Because we also have to add a null character in the array, the array's length is one greater than the length of the string. Alternatively, we can define the above string like this as well:
The array str_array will still hold 7 characters because C++ automatically adds a null character at the end of the string. If we use the sizeof() function to check the size of the array above, it will return 7 as well.
How to Define a String in C++
Now that we have a basic understanding of strings in C++ let us go through the different ways of defining a string in C++.
-
Using the string keyword is the best and the most convenient way of defining a string. We should prefer using the string keyword because it is easy to write and understand.
-
Then we have the C-style strings. Here are the different ways to create C-style strings:
Alternatively, we have:
It is not necessary to utilize all the space allocated to a character array (string):
How to Take String Input
To provide our program(s) input from the user, we generally use the cin keyword along with the extraction operator (>>). By default, the extraction operator considers white space (such as space, tab, or newline) as the terminating character. So, suppose the user enters "New Delhi" as the input. In that case, only "New" will be considered input, and "Delhi" will be discarded.
Let us take a simple example to understand this:
Input:
Output:
In the above example, the user entered "New Delhi" in the input. As " " is the terminating character, anything written after " " was discarded. Hence, we got "New" as the output.
To counter this limitation of the extraction operator, we can specify the maximum number of characters to read from the user's input using the cin.get() function.
Let us take an example to understand this:
Input:
Output:
Now, we could get the whole string entered by the user.
Apart from this method, we can also use the getline() function. The getline() function is explained below in this article.
String Operations in C++
Although the implementation of character arrays is faster than that of string objects, string objects are widely used in C++ because of the number of in-built functions it supports. Some of the most commonly used string functions are as follows:
Input Functions
There are three widely used input functions. These are:
-
getline() : The getline() function is used to read a string entered by the user. The getline() function extracts characters from the input stream. It adds it to the string object until it reaches the delimiting character. The default delimiter is \n. The delimiter could either be a character or the number of characters a user can input.
For example:
Assume the Input Given by the User is as Follows:
The Output Will Look Like This:
We can observe in the above example that the user input was "Software Engineer". But, because we had specified a limit of 12 characters, the output we got was the first 12 characters of the input string, i.e., "Software En".
-
push_back() : The push_back() function is used to push (add) characters at the end (back) of a string. After the character is added, the size of the string increases by 1.
For example:
Output:
Using the push_back() function several times, we added the word " Topics" at the end of the string.
-
pop_back() : The pop_back() function is used to pop (remove) 1 character from the end (back) of a string. As the character gets removed, the size of the string decreases by 1.
For example:
Output:
Name before using pop_back: Scaler Name after using pop_back: Scale
As we can see, the last character of the name popped out, and the string is left with one less character.
Capacity Functions
The most commonly used capacity functions are:
-
length() : As the name suggests, the length() function returns the length of a string.
For example:
Output:
-
capacity() : The capacity() function returns the current size of the allocated space for the string. The size can be equal to or greater than the size of the string. We allocate more space than the string size to accommodate new characters in the string efficiently.
For example:
Output:
-
resize() : The resize() function is used to either increase or decrease the size of a string.
For example:
Output:
In the above example, we used the resize() function to change the number of characters in the string. Because we had specified the first ten characters in resize(), all the characters after the first 10 were removed from the string.
-
shrink_to_fit() : The shrink_to_fit() function is used to decrease the string’s capacity to make it equal to the string’s minimum capacity. This function helps us save memory if we are sure that no additional characters are required.
For Example:
Output:
In the above example, after using the resize() function, we realized that the string capacity was 28. When we used the shrink_to_fit() function, the size was reduced to 17.
Iterator Functions
The most commonly used iterator functions are:
-
begin() : The begin() function returns an iterator that points to the beginning of the string.
For Example:
Output:
In the above example, we first had to declare an iterator it. The address returned by the begin() function was stored in this iterator. Then, we used *it to print the value stored at the returned address.
-
end() : This function returns an iterator that points to the end of the string. The iterator will always point to the null character.
For Example:
Output:
-
rbegin() : The keyword rbegin stands for the reverse beginning. It is used to point to the last character of the string.
For example:
Output:
The difference between rbegin() and end() is that end() points to the element next to the last element of the string while rbegin() points to the last element of a string.
-
rend() : The keyword rend stands for the reverse ending. It is used to point to the first character of the string.
For example:
Output:
Manipulating Functions
The most commonly used manipulating functions are:
-
copy() : The copy() function is used to copy one sub-string to the other string (character array) mentioned in the function's arguments. It takes three arguments (minimum two), target character array, length to be copied, and starting position in the string to start copying. For Example:
Output:
-
swap() : The swap() function swaps one string with another. For Example:
Output:
Concatenation of Strings in C++
Combining two or more strings to form a resultant string is called the concatenation of strings. If we wish to concatenate two or more strings, C++ provides us the functionality to do the same.
In C++, we have three ways to concatenate strings. These are as follows.
1. Using strcat() function
To use the strcat() function, we need to include the cstring header file in our program. The strcat() function takes two character arrays as the input. It concatenates the second array to the end of the first array.
The syntax for strcat is:
It is important to note that the strcat() function only accepts character arrays as its arguments. We can not use string objects in the function.
For Example:
The output of the above program is:
2. Using append() function
The append() function appends the first string to the second string. The variables of type string are used with this function. The syntax for this function is:
Let us Take an Example:
Output:
3. Using the '+' operator
This is the easiest way to concatenate strings. The + operator takes two (or more) strings and returns the concatenated string. Its syntax is:
For Example:
Output:
It is up to the programmer to use + or append(). Compared to +, the append() function is much faster. However, in programs that do not have very long strings, replacing + with append() will not significantly affect program efficiency. On the other hand, the strcat() function has some potential performance issues based on the function's time complexity and the number of times the function runs in the program. So, in a nutshell, it is best to use the append() function or the + operator.
Conclusion
- Strings in C++ can be defined in two ways: using the string class or character arrays.
- It is more convenient to use string objects over character arrays.
- The string class provides many functions for the string objects.
- We can take string inputs from the user using the cin keyword or getline() function.
- There are three ways to concatenate strings in C++: using strcat(), append(), or + operator.