String Stream in C++
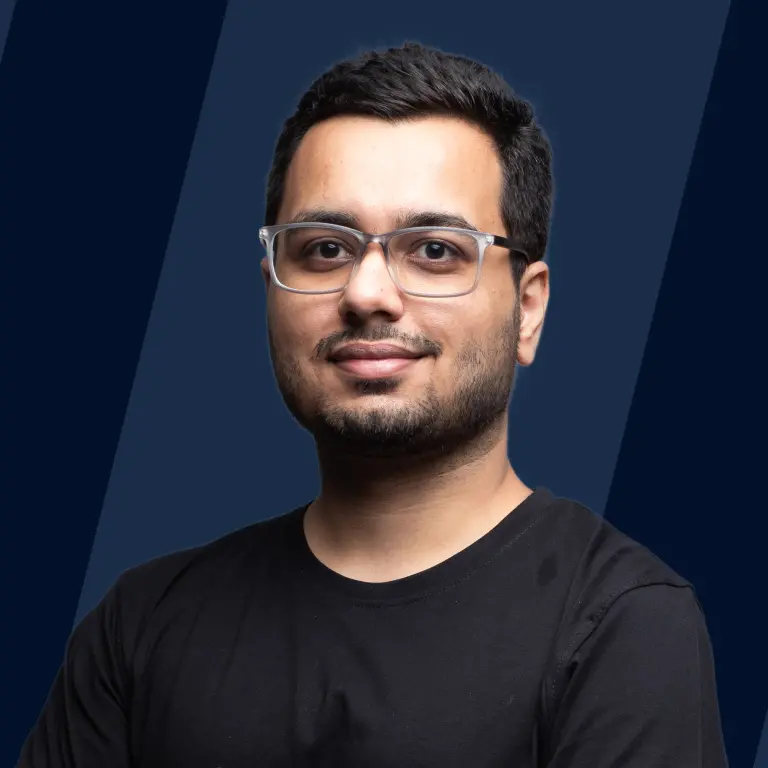
Overview
While programming in the C++ language, we deal with many streams, and the most common are cin and cout streams, which are used to take the user input and provide the output. Where the stream is just the flow of data into the program, from the program, or stored in some buffer memory where we can perform read, write, and clear operations.
stringstream is a stream that allows the user to work with a string, and the user can perform various operations on the string.
What is Stringstream Class in C++?
C++ programming language is an Object Oriented Programming language where the concept of classes makes it easy to re-use a code script and easily puts data in a mannered way. For input/output operation on objects like primitive data types or user-defined data types, we have the iostream class present, which stands for or works for input/output stream.
Let’s see an image showing for C++ programming languages how classes are derived from one another, which means this is the representation of which class is a parent and which is derived from it. Then we will move to the stringstream class:
We can see the ios_base class is the main class then there are classes derived from it, with the stringstream class a part of it. The stringstream class is the driven class from the iostream class, which works especially on the string objects. Stringstream class in C++ allows users to treat strings as the stream of characters and make it easy to parser the input ( parsing the input means a variable of any input can be converted into the string easily and vice versa ). To use the stringstream class, we have to include the <sstream> library in our code, as the stringstream class is defined in it.
Commonly Used Methods of Stringstream Class in C++
The main application of any stream is to let the user perform some operations like adding data to the stream, extracting data from the stream, or performing some other function like manipulating data, etc. Let’s see some of the commonly used methods of the string stream class in C++:
- clear(): In String stream in C++, as the name suggests clear() function clears the stream, erasing data from the stream.
- str(): str() function of the stringstream in C++ class can perform both read and write methods which means with the help of the str() function, we can write in the stringstream object and by using it, we can get the data from the object which is written in the object.
- operator <<: We have seen the insertion operator (<<) many times in the C++ programming language, most commonly while providing user output data. We use it with ‘cout’ to write data in the output stream. Similarly, we will add data to the stringstream class object by using the insertion operator.
- operator >>: We have seen the extraction operator (>>) many times in the C++ programming language, most commonly while getting user input data. We use it with ‘cin’ to extract data from the input stream. Similarly, using the extraction operator, we will get data from the stringstream class objects.
How to Create a Stringstream Object in C++?
We have discussed the basics of the stringstream until now in this article. Now we will create the string stream in a C++ object. stringstream object is created by the keyword ‘stringstream’ and the object's name. Let’s see the syntax to create an object of the stringstream:
In the above syntax, we can see how to create a stringstream object. It is similar to creating an object for a normal class. Also, we need the <sstream> header file in our code to include the stringstream library.
Let’s see a code example to declare a String stream in a C++ object:
Code:
Explanation: In the above code, we have included the <sstream>header file and then implemented the above-declared syntax in code format.
How to Perform Write Operations in Stringstream in C++?
We have three methods to perform the write operation in the stringstream object. Writing in stringstream means adding the data in the object of the stringstream class for which we can:
- Using Constructor: While declaring the stringstream object by passing the string or any other data variable to the stringstream constructor.
- Using insertion (<<) operator: We can use the insertion operation in the same way we use it with cout and can write data in stringstream object.
- Using the str() function: As we have seen the str() function of the stringstream class, we can insert any string value to the stringstream class object.
We have seen the methods to perform the write operation in the stringstream object. Now let’s move to the code implementation of these methods to get a better idea:
Code:
Output:
Explanation: In the above code, we have used above defined three methods to perform the write method in the String stream in a C++ object. First, we used the constructor method for obj1, then for obj2, we used the insertion operation, and at last, we used the str() function of the stringstream class to add data in obj3. Also, for each object of stringstream in C++, we have printed the value using the str() method.
How to Perform Read Operations in Stringstream?
We have two different methods to perform the read operation from the stringstream object. Reading from the stringstream means getting the data written in the object of the stringstream class for which we can:
- Using extraction (>>) operator: We can use the extraction operation in the same way we use it with cin to get data from the input stream and can read data from the stringstream object.
- Using the str() function: As we have seen the str() function of the stringstream class above in the article, we can use it to get data from the stringstream class object.
We have seen the methods to perform the read operation on the stringstream object. Now let’s move to the code implementation of these methods to get a better idea of how to use them:
Code:
Output:
Explanation: In the above code, we have declared object obj1 of the stringstream class, and by using the extraction operator (>>), we have added the data into obj1. Similarly, by using the str() method of stringstream in the C++ class, we added the data to the obj2 object. Also, by using the stringstream in the C++ class function str(), we printed the data presented in the objects.
How to Clear the Content of Stringstream Objects in C++?
To clear the content from the stringstream object means deleting data in the stringstream in the C++ object. We have already seen the clear() function above in the article, using which we can delete the stringstream data.
Let’s see the code implementation to use the clear() function to erase the data of the String stream in the C++ object:
Code:
Output:
Explanation: In the above code, we added the data into the stringstream object using the constructor method. Then we read that data by using the extraction operator to get proof that data is present in the object. After that, we used the clear() method to delete the data, and for proof, we again tried to extract the data from the object of stringstream but got nothing as it was cleared.
Applications of Stringstream Class in C++
There are various applications of the stringstream class in C++. Let’s see some of them:
- A string object can be treated as a stream by adding the stringstream class in C++ program, which means the user can write data to it, read data from it, and finally clear data from it.
- Stringstream in C++ allows us to perform the insertion and extraction to/from the string similar to the cout and cin streams.
- Stringstream in C++ makes the parsing of the input very easy, and users can convert any primitive data type to string easily.
Applications of Stringstream in C++
Stringstream in C++ makes it easy to play/work with strings. There are several functions, such as counting the number of words in the string, getting the frequency of any particular word in a given string, converting any primitive data type into a string variable/object, converting strings to integers, etc. Let’s see some of the stringstream in C++ applications:
Counting the Number of Words in a String
In C++, we can have a string consisting of some words that are differentiated by spaces. If we have to find the number of words in any given string, we can manually traverse over the string, and at every whitespace or at the end of the string, we can increase the count of the number of words. String stream in C++ provides a short and straight method to find the number of words in a string. Let’s see its code and discuss it:
Code:
Output:
Explanation: In the above code, we added the data into the stringstream object using the constructor method. Then we created a string to extract words from the stringstream object and an integer to get the count of the number of words. We iterated a while loop, which will work until every word is extracted from the given object, and at every iteration, we increased the count and, at last, printed it.
Printing Frequencies of Individual Words in a String
In the above section, we have seen the method to find the number of words in a string, and that was done by getting words with the help of an extraction operation. Now, we can store the words on a map and count their frequency. Let’s see its code and understand it in a better way:
Code:
Output:
Explanation: In the above code, we added the data into the stringstream object using the constructor method. Then we created a string to extract words from the stringstream object and a map to store the frequency of the particular word. We iterated a while loop, which will work until every word is extracted from the given object, and at every iteration, we increased the count of every word and, at last, printed it all of the words and their frequency by iteration over the map.
Conclusion
- Stringstream is a type of stream that allows the user to work with a string, and the user can perform various operations on the string.
- Stringstream class is the driven class from the iostream class, which works especially on the string objects and allows users to treat strings as the stream of characters.
- Stringstream is defined in the <sstream> header file of the C++ programming language.
- There are four common methods used in the stringstream class:
- clear(): Used to clear the data present in the stringstream object.
- str(): Used to add or read data from the stringstream object.
- Extraction operator(>>): As the name suggests, it reads data from the object.
- Insertion operator(<<): It adds data to the stringstream object.
- There are three ways to add data to a stringstream object: constructor, str() function, and insertion operator.
- There are two ways to read data from the stringstream object: inbuilt str() function and extraction operator.
- Stringstream in C++ makes the parsing of the input very easy, and users can convert any primitive data type to string easily.