Building a Student Record Management System in C - C Projects
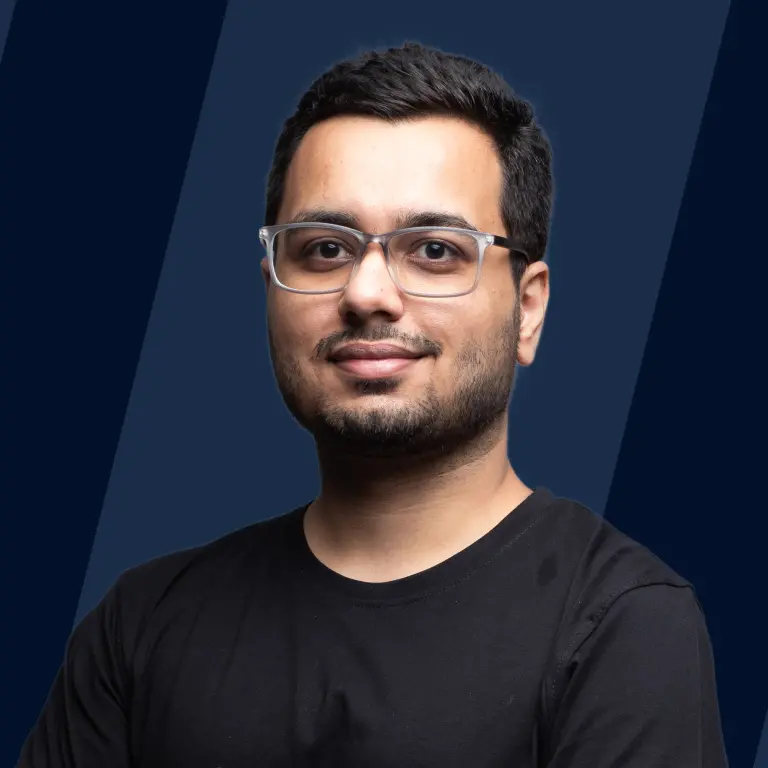
Overview
In this article, we are going to build a mini Student Record Management System using C language . This project is based on the concepts of arrays, and in this we can conveniently add, manage and modify the data record of students of a school or a university, like adding a new Student's record, modifying existing records, searching if a student exists or not and more. In this project, each task is distributed efficiently among different functions to improve the readability of the code and to help the reader better understand the concepts.
What are We Building?
In this project, we will be building a mini Student Record Management System in C language, in which we can add a new student's record, modify existing student records, search if a student exists or not, delete a student's record, and more.
Pre-requisties
For this project, the reader is only required to have a basic understanding of
Key Features of the Project
In this project, the user can
- Add a new student's record
- Modify the record of existing students
- Search if a student exists or not
- Delete a student's record
- Change the user password and more.
How are We Going to Build the Survey Form
The approach that we are going to follow is, to create individual functions for every task, and all the functions will be then unified together to make the project functional, the data structure that we will be using in this project are simply the user defined structure arrays.
- Adding Student Details - Ask the user to enter the student's detail, and add it to the list of students, while adding the new student to the list, check the uniqueness of the roll number.
- Finding a student by the entered roll number - Asking the user to enter a roll number and then printing the details of the respective student.
- Finding the student by the first name - Asking the user to enter the first name of the student and then printing the details of the respective student.
- Finding the students registered in a course - Asking the user to enter a course id and then printing the details of the respective students.
- Count of Students - Prints the total number of students in the system.
- Delete a Student - Ask the user to enter a roll number and then delete the details of the respective student.
- Update a Student - Ask the user to enter a roll number and then updates the details of the respective student.
Final Output
After writing the complete program we will compile and then run it to see its outcome and to start using a mini project on students' record management system of our own.
Requirements
We will be needing the following functions
- addStudent()
- findByRollNumber()
- findByName()
- findRegisteredStudent()
- totalCount()
- deleteStudent()
- updateStudent()
Building Student Record Management System in C
Now as we have gone through the overview of the project and the approach that we are going to follow in creating it, so now it is a good time to start writing the code itself.
Adding the required header files
Defining the Structure StudentInfo and the Counter Variable
Function to Add Student Details
Function to Find Students Using Roll Number
Function to Find Students by their First Name
Function to Find Students Enrolled in a Specific Course
Function to Print the Total Number of Students
Function to Delete a Student
Function to Update a Student's Data
The main() Function
Explanation
- Starting from the main() function, we are declaring a variable taskToPerform to know which specific operation the user wants to perfrom into the Student Record Mangement Systems (SRMS for short), and then we are are starting an infinite while loop which will end, but on the user's command, by default it will be keep getting executed always.
- After that we are asking the user to enter what operation they want to perform and are storing the entered response into the taskToPeform varaible, here the response value 8 will be used to exit the infinite loop.
- Now, we have got the response of the user, and we are starting a switch block after that, and are calling the respective methods as per the response given.
- Now lets understand what each method is doing
- First of all we are creating a structure data type structureInfo to store the data records of the students in the required format, and are declaring the initial capacity to 500 students by creating a structure array st.
- addStudents() method - This method will let the user add a new student's record, by providing its firstName, lastName, rollNumber, CGPA and the courses in which they are enrolled and in the end we are incrementing the global variable i which we defined to keep track of number of students.
- findByRollNumber() method - This method will let the user to find if a student exists or not using its rollNumber provided, in this we are asking the user to enter the roll number, and then are checking if any currently existing student has the matching roll number or not, by iterating over the complete array database with the help of a for loop. If found we are printing its details and if not found we are exiting the loop after completing the check.
- findByName() method - This method will let the user to find if a student exists or not by verifying its firstName, in this we are asking the user to enter the first name of the studnet, and then are checking if any currently existing student has the matching firstName or not using the strcmp() function present in the standard string.h header file. If found we are printing the details and if not found we are exiting hte loop after completing the check.
- findRegisteredStudent() method - This method will let the user to find if a student exits or not using the course ids, in this we are asking the user to enter the course id, and then are checking if any currently existing student is enrolled into that specfic course, if found we are printing the details of that student, and if not found we are exiting the loop after completing the check.
- totalCount() method - This method will display the total number of students into the system using the global variable we have declared in the start to keep track of the number of students.
- deleteStudent() - This method will let the user to delete a student's record, in this we are asking for the roll number of a student, and are iterating over a for loop onto the complete array database for that student, once found, we are simply updating the value at that position in the memory by its succssive position and hence removing the record, and then we are also decrementing the value of the i variable by 1 to keep the count of students accurate.
- updateStudent method - This method will let the user to update the records of a student using its roll number, in this we are asking the user to enter the student's roll number and then are checking if a student with that roll number exists or not, if found we are then asking what specific detail that the user wants to update, and then are updating that, after successful completion, we are simply printing the success message.
google drive link for 1080p video
Now you can try writing the code by yourself by taking a reference from the above and start using your own program of Student record management system written in C language.
What's Next
In this article, we have got a clear understanding of how a management system works, what approach we need to follow, and how we can break each operation into small pieces of code to ease our work. In this article, we have used the concept of arrays to implement this project, but it has a problem, the data that we are writing into the console or the terminal is available for that specific session only, meaning as soon as we will close the terminal, all the entered data will be vanished, so to overcome it, what can be done is, to take this program a step further.
How? By implementing the file handling concepts into it, where the data entered by the user will not be stored in an array but will be stored in the local files using the different modes of file streams, and hence all the entered data will be preserved for future usage.
Conclusion
- In this article, we have built a mini Student Record Management System in C language using the concepts of arrays.
- In this project, the user can add a new student's record, modify existing records, search for a specific student's record, delete a student's record, and more.
- We have built it by creating individual functions for each of the operations that can be performed, hence improving the code readability.
- After this project, the user should try to implement the same project using the file handling concept, which will keep the entered data persist even after closing the program.
- The user should also try to add some new features, like mark sheet info of a student, attendance system, etc. to improve their understanding of the concepts.