Swapping of Two Numbers in C++
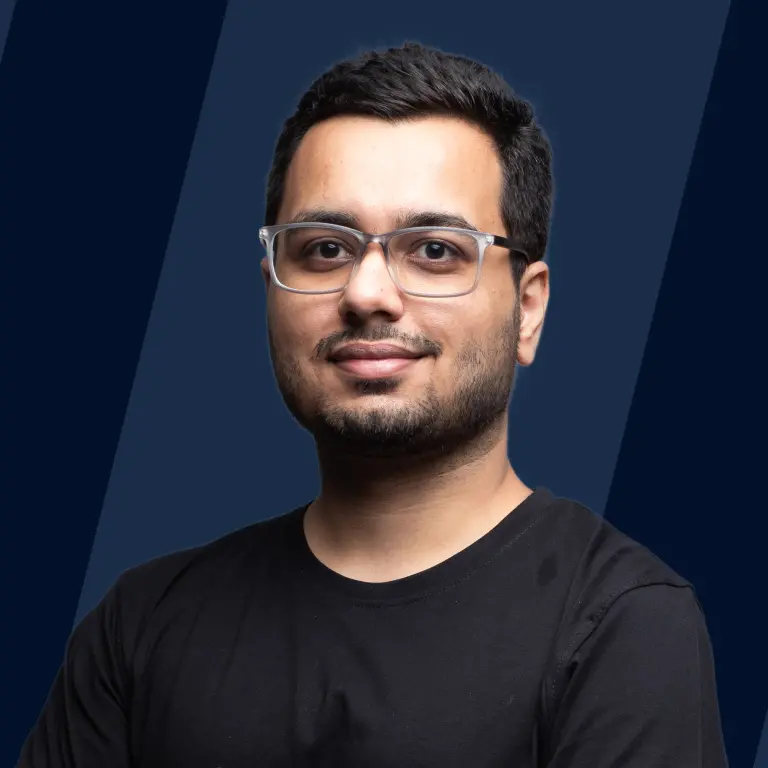
Overview
Swapping two numbers means exchanging the values of two variables with each other. Swapping two numbers in C++ is one of the most basic programs frequently used in various sorting algorithms.
Swap Two Numbers in C++ Using Temporary Variable
Let’s take an interesting example to understand this concept:
Suppose we have three boxes. Box 1 has an apple, Box 2 has an orange, and Box 3 is empty. We need to interchange the fruits in Box 1 & 2 with the help of Box 3.
So we follow the below steps...
- First, we take an apple from Box 1 and place it inside Box 3.
- Now Box 1 is empty, take an orange out of Box 2 and place it inside Box 1.
- Now Box 2 is empty, take an apple out of Box 3 and place it inside Box 2.
- Finally, items of both Boxes are now exchanged, i.e., swapped.
Algorithm
- Start: Begin the process.
- Initialize Variables: Define three variables, a, b, and temp. Assign values to a and b that you want to swap.
- Swap Numbers:
- Assign the value of a to temp.
- Assign the value of b to a.
- Assign the value of temp to b.
- Display Swapped Numbers: Print the values of a and b after the swap.
- End: The process is complete.
Program to Swap Two Numbers using Temporary Variable
Output:
Three variables are created to perform the swapping in which the contents of the first variable, a, are stored in the temp variable. Then, the contents of the second variable b are stored in the first variable a. Finally, the contents of the temp variable are stored back to the second variable b, which completes the swapping operation.
Complexity
Time Complexity: O(1), as only constant time operations are done. Space Complexity: O(1), as no extra space has been used.
Swap Numbers Without using Temporary Variables (using * and / only)
Algorithm
- Start: Initiate the process.
- Initialize Variables:
- Define two variables, a and b.
- Assign the desired values to a and b.
- Check for Zero:
- Ensure neither a nor b is zero, as division by zero is undefined.
- Swap Numbers:
- Multiply a by b and store the result in a.
- Now, a holds the product of the initial values of a and b.
- Divide the new value of a by b and store the result in b.
- Now, b holds the initial value of a.
- Divide the new value of a by the new value of b and store the result in a.
- Now, a holds the initial value of b.
- Multiply a by b and store the result in a.
- Display Swapped Numbers:
- Output the swapped values of a and b.
- End: Conclude the process.
Program to Swap Two Numbers Without using Temporary Variable
Output:
Let us understand the workings of the above program:
- Initially, a = 6 and b = 2.
- Then, we multiply a and b and store them in the program using a = a * b. This means a = 6 * 2. So, a = 12 now.
- Then, we divide a and b and store them using b = a / b. This means b = 12 / 2. So, b = 6 now.
- Finally, we divide a and b again using a = a / b. This means a = 12 / 6. Therefore, a = 2.
Complexity
Time Complexity: O(1), as only constant time operations are done. Space Complexity: O(1), as no extra space has been used.
Swap Numbers Without Using Temporary Variables (using + and - only)
Algorithm
- Start: Initiate the swapping process.
- Initialize Variables:
- Define two variables, a and b.
- Assign the initial values to a and b that you wish to swap.
- Swap Numbers:
- Add the values of a and b and store the result back in a.
- Subtract the value of b from a and store the result in b.
- Subtract the value of a from b and store the result back in a.
- Display Swapped Numbers:
- Output the new values of a and b, which have now been swapped.
- End: Conclude the swapping process.
Program to Swap Two Numbers Without using Temporary Variable
Output:
Let us now understand the workings of the above program:
- Initially, a = 6 and b = 2.
- Then, we add a and b and store them in the program using a = a + b. This means a = 6 + 2. So, a = 8 now.
- Then, we subtract a and b and store them in the program using b = a - b. This means b = 8 - 2. So, b = 6 now.
- Finally, we use a = a - b. This means a = 8 - 6. Therefore, a = 2.
Complexity
Time Complexity: O(1), as only constant time operations are done. Space Complexity: O(1), as no extra space has been used.
Swap numbers using “swap” function
The function std::swap() is a built-in function in the C++ Standard Template Library (STL) that swaps the value of two variables.
Syntax of swap() Function
Parameters
The swap function takes two parameters, a and b, which are to be swapped. These parameters can be of any data type.
Return Values
The swap function does not return anything, as it is used to swap two numbers.
C++ Program to Swap Two Numbers Using Inbuilt swap() Function
Output
FAQs
Q: Can I swap two numbers without using any arithmetic or temporary variable in C++?
A: Yes, you can use the XOR bitwise operator to swap two numbers without using any arithmetic operations or temporary variables.
Q: Is it more efficient to swap numbers with or without a temporary variable?
A: Swapping numbers without a temporary variable generally requires fewer memory resources, but it might be less readable and more prone to errors, especially when arithmetic operations are involved.
Q: What will happen if I try to swap two numbers using division and multiplication if one of them is zero?
A: If one of the numbers is zero, the program will encounter a division by zero error, which is undefined behavior and could cause the program to crash or produce incorrect results.
Q: Are there any built-in functions in C++ to swap two numbers?
A: Yes, C++ Standard Template Library (STL) provides a built-in function std::swap() that can be used to swap two numbers easily and efficiently.
Conclusion
- While some methods like swapping without a temporary variable can be more efficient in terms of memory usage, they may compromise code readability and are more prone to errors. Choosing the right method depends on the specific context and requirements of the task at hand.
- Especially when dealing with arithmetic operations, it is vital to ensure that the code is robust and handles edge cases properly, such as avoiding division by zero.
- The std::swap() function in C++ provides a reliable and straightforward way to swap two numbers, showcasing the power and utility of the C++ Standard Template Library.
- Understanding how to swap values efficiently is applicable in various programming scenarios, including sorting algorithms, data transformation, and memory management.
- Swap operations are not just limited to simple data types; they can also be extended to user-defined types and objects, making the understanding of swapping mechanisms even more crucial for advanced C++ programming.