Python Program to Swap Two Numbers
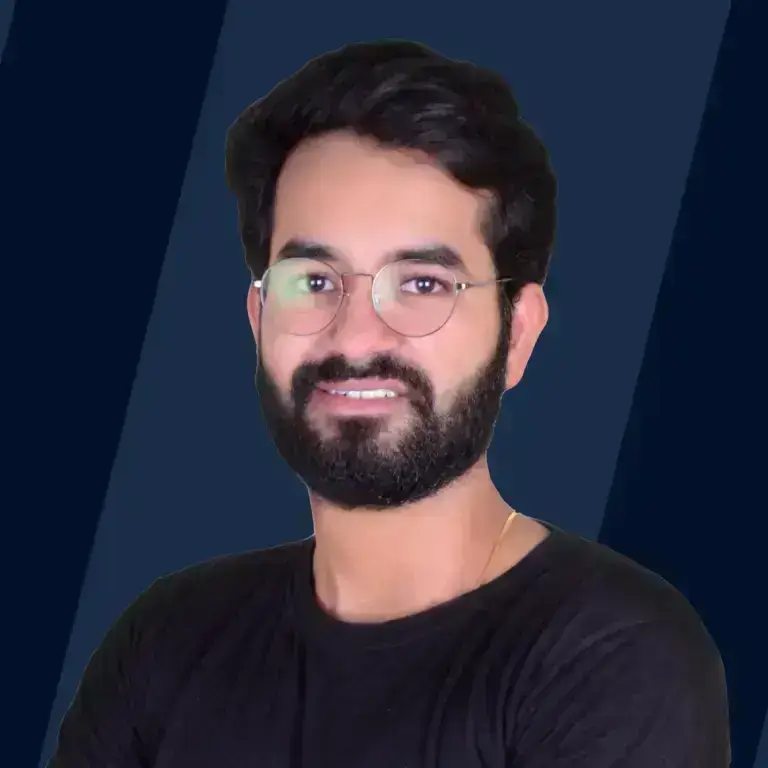
Overview
Through the article, we will explore the swapping of values of two numbers in Python Programming.
There are various applications of the swapping method like games (where the score will swap if the other side gets to know what is unknown) for improving main memory utilization in Operating systems etc.
Swapping of two numbers in python is an approach by which the value of one object gets swapped or exchanged with the value of the second. For example, if was and was , then by using the Swap two numbers in python the result would be - will contain value and will contain value .
Introduction
Swapping of two numbers in python can be explained as the method of exchanging or trading the values which are stored in two different objects.
There is no specific syntax for doing the same. Still, by incorporating the concepts of Python data types, operators, input, output, imports, etc., we shall develop easy-to-understand code that can help us achieve the objective of swapping two numbers in python.
We will not be restricted to the naive approach of swapping two numbers in python but will explore different ways of doing the task with the help of Python.
Python Programs to Swap Two Numbers
When we want to pass back and forth the values stored in two variables, we can use the following methods to achieve our objective of swapping two numbers in python.
The two main methods of swapping two numbers in python are:
a. By using a Temporary Variable
b. Without using Temporary Variable
With Using a Temporary Variable (example program)
The most widely used or naive way of swapping two numbers in python is by using the Temporary variable.
In this method, we first store the value of one variable (say 'num_S') in the temporary variable (say temp). Then we assign the variable 'num_S' to the value for the second variable (say 'num_T'). Finally, we complete this task of exchanging the value between two variables by assigning the variable 'num_T' with the temporary variable (temp) for the value given.
With the code below, we will understand this approach well.
CODE:
OUTPUT:
Without Using a Temporary Variable (example program)
We saw a method where we needed a third variable to store and exchange the value. Now we shall be understanding approaches where we can swap the value of a variable between two numbers without using the Temporary Variable in python.
Below are a few of them:
Using Comma Operator
With the help of the comma operator, we can swap two numbers in python without using any third temporary variable.
The following example will help you understand it.
CODE:
OUTPUT:
Using XOR Operator
Now we shall use the bitwise XOR operator to swap two numbers in python. Lets us briefly understand the concept before jumping to the code.
The result obtained after the XOR operator is applied to two variable (say 's' and 't') follow a simple rule:- The bit '1' will be returned whenever the bits of the two variable differ. Else the bit '0' will be observed.
Now let us dive into the below code to understand swapping two numbers in python using the XOR operator.
CODE:
OUTPUT:
Using Arithmetic Operators - Addition and Subtraction Operator
Using the arithmetic - addition, and subtraction operator, we add the two numbers in one variable and then follow the subtraction method to both variables to swap the two numbers in python.
The following example will help you understand it.
CODE:
OUTPUT:
Using Arithmetic Operators - Multiplication and Division Operator
Using the arithmetic - multiplication, and division operator, we first multiply the two numbers in one variable and then follow the division method to both variables to swap the two numbers in python.
The following example will help you understand it.
CODE:
OUTPUT:
Check out this article to learn more about Arithmetic Operators.
Conclusion.
- Swapping of two numbers in Python can be explained as the method of exchanging or trading the values which are stored in two different objects.
- The two main methods of swapping two numbers in python are: a. With Using Temporary Variable b. Without Using Temporary Variable
- While Using a Temporary Variable to swap two numbers in python we use a third variable, temp to temporarily store the value to it and then equate the two variables and finally assign the second variable to the value of the temp variable.
- Without Using Temporary Variable can be diversified as below:
- Using Comma Operator
- Using XOR
- Using addition and subtraction operator
- Using multiplication and division operator