Types of Errors In Java
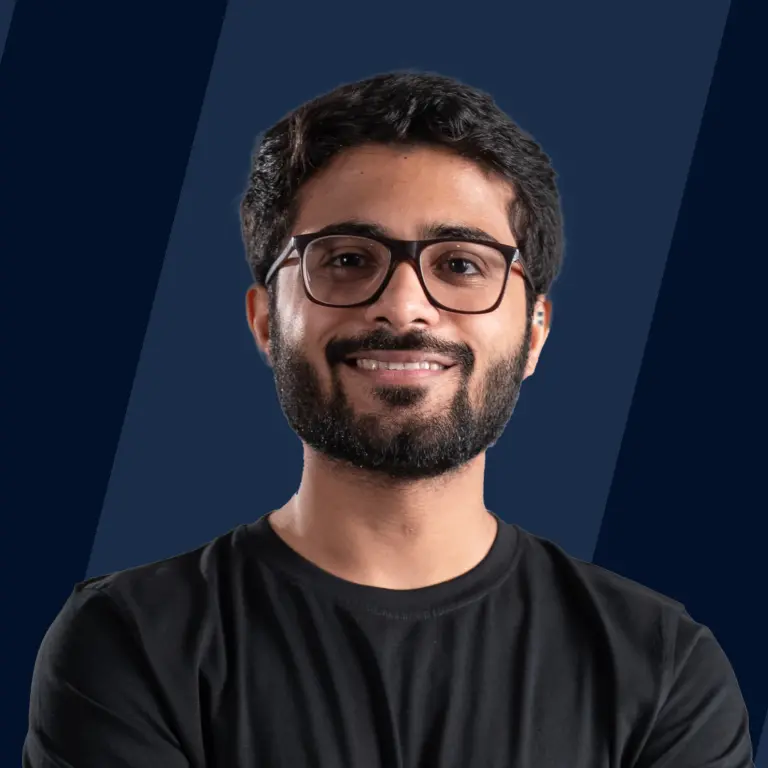
Overview
If you have chosen software engineering as your career, then one thing that's going to be with you till the end of your career journey is error or commonly known as a bug.
Error is an illegal operation performed by the programmer which causes the program to behave abnormally.
To solve errors and make your application perform as intended, it's really important to understand errors. In this article, we are going to learn about different types of errors in Java.
What is an Error in Java ?
An error is an illegal statement or operation performed by the programmer that causes the application or the program to behave abnormally and restricts the application from performing its tasks efficiently. Errors usually remain undetected until the program is compiled or executed. Some may cause the program's termination or may inhibit the program from getting compiled or executed.
When can an error occur :
- Java programming language has a set of rules to write its program. An error can occur if the programmer will not follow these rules and regulations.
- When a programmer performs an operation that is not intended because of a wrong idea or concept.
Types of Error in Java
Now we know what is an error in Java, let's now learn about different types of errors in Java.
1. Syntax Errors
- Syntax errors occur when syntactical problems occur in a Java program due to incorrect use of Java syntax.
- These syntactical problems may be :
- missing semicolons,
- missing brackets,
- misspelled keywords,
- use of undeclared variables,
- improperly named variable or function or class,
- class not found,
- missing double-quote in Strings, etc.
- These errors are detected by the Java compiler at compile time of the program which is why they are also known as compile-time errors.
- Syntax errors are easy to spot and rectify because the Java compiler finds them for you. The compiler will tell you which piece of code in the program got in trouble and its best guess as to what you did wrong. Usually, the compiler indicates the exact line where the error is, or sometimes the line just before it.
- When the Java compiler encounters syntax errors in a program, it prevents the code from compiling successfully and will not create a .class file until errors are not corrected. An error message will be displayed on the output screen.
Examples :
Missing semicolon :
Output :
Explanation :
In the above code semicolon is missing at the end of the int statement.
Missing bracket :
Output :
Explanation :
In the above code the closing curly bracket of the main() method is missing.
Misspelled Keyword :
Output :
Explanation :
In the above code, the System keyword in System.out.println() name is misspelled as a system.
Invalid Variable or Function or Class name :
There are certain rules to name an identifier(variable, function, and class name are called identifiers) in Java :
- All identifiers should begin with a letter (A to Z or a to z), a currency character ($), or an underscore (_).
- After the first character, identifiers can have any combination of characters.
- A keyword cannot be used as an identifier.
- Most importantly, identifiers are case-sensitive.
- Examples of legal identifiers :
name3, $uid, _value, _3b__age, roll_no. - Examples of illegal identifiers :
13ab, -age.
Output :
Explanation :
In the above code, the name of the main class is invalid as it starts from a number.
Cannot find the symbol :
Output :
Explanation :
In the above code, we have not imported the java.util.Scanner package and using Scanner to take integer input. This has caused the error.
Missing double-quote in String :
Output :
Explanation :
In the above code the Java compiler is throwing multiple errors because it is not considering the "Hello World!" as a String as it is missing the double quotes.
2. Runtime Errors
Runtime errors occur when the program has successfully compiled without giving any errors and creating a ".class" file. However, the program does not execute properly. These errors are detected at runtime or at the time of execution of the program.
The Java compiler does not detect runtime errors because the Java compiler does not have any technique to catch these errors as it does not have all the runtime information available to it. Runtime errors are caught by Java Virtual Machine(JVM) when the program is running.
These runtime errors are called exceptions and they terminate the program abnormally, giving an error statement.
Exceptions are errors thrown at runtime. We can use exception handling techniques in Java to handle these runtime errors. In exception handling, the piece of code the programmer thinks can produce the error is put inside the try block and the programmer can catch the error inside the catch block.
Example of exception handling in Java program :
Output :
Without exception handling :
Output :
We can see from the above examples that when we want to handle the runtime error explicitly then we use exception handling or a try-catch block. Using this a programmer can perform a specific task when he/she encounters a runtime error or exception during the execution of the program.
A runtime error can happen when :
- Dividing an integer by zero.
- Trying to store a value into an array that is not compatible type.
- Trying to access an element that is out of range of the array.
- Passing an argument that is not in a valid range or valid value for a method.
- Striving to use a negative size for an array.
- Attempting to convert an invalid string into a number.
And many more.
Examples of Runtime Errors :
Accessing array index that does not exists :
Output :
Explanation :
When we try to access an array index that is out of bounding of the size of the array(in this case it's 5), we get runtime error of array out of bound.
Dividing an integer by zero :
Output :
Explanation :
We cannot divide anything from zero so when we try to do that we get an arithmetic exception at runtime.
Using the negative size of an array :
Output :
Explanation :
We cannot have a negative-sized array, it is just not possible. We get a Negative array size exception when we try to do that.
3. Logical Errors
Logical errors are the hardest to identify and rectify. They are hardest to detect because they are neither identified by the Java compiler nor by the JVM. The programmer is entirely responsible for them.
The program with a logical error will get compiled and executed successfully but will not give the expected result.
Logical errors can be detected by application testers when they compare the actual result with the program's expected result.
Examples :
Program to print even numbers :
The programmer wants to print even numbers but, instead of using a modulus operator(%) he/she uses a divide operator (/).
Actual Output :
Expected Output :
Explanation :
The actual output and the expected output do not match which means the programmer has committed a logical error somewhere in the program. We wanted to print even numbers, but we got 0 and 1 because we were using "/" and not "%".
Errors v/s Exceptions
Errors | Exceptions |
---|---|
Cannot be handled by any method and always leads to the termination of the program. | Can be handled through exception handling, using try and catch block. |
Can be a Syntax or Logical error. | Runtime errors are called exceptions. |
They are defined in the Java.lang.Error package. | They are defined in the Java.lang.Exception package |
Classified as unchecked type | Classified as both checked and unchecked |
Check the detailed comparison between Error and Exception from here.
Conclusion
- Errors are a vital part of programming.
- Learning about errors and how to solve them is an essential skill for every developer.
- The process of finding errors or bugs in your code is called debugging.
- The article aimed to introduce you to different types of errors that you will encounter in the Java programming language.
- To keep your program error-free as much as possible and prevent it from behaving abnormally, follow all the Java programming language rules and regulations.