Difference Between Error and Exception in Java
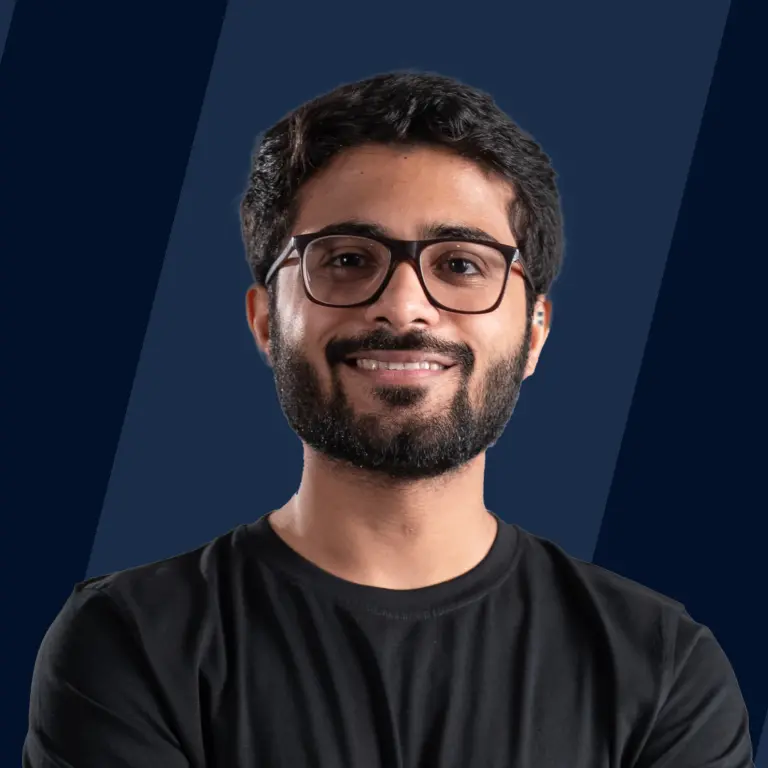
In Java, both Error and Exception are subclasses of Throwable, with Error often indicating severe system resource issues, while Exceptions can occur during runtime and disrupt normal program flow, often being manageable through specific code constructs.
Errors to Exceptions
Researchers in the tech industry analyzed the reasons behind the failures of real-world software & hardware devices and found out there were errors in the programs used in the devices. They found out some unique scenarios in programs that can lead to error and gave them the name of exceptions, so that if in the future that condition tries to happen again, then the compiler or the program halts the compilation or execution and terminates the program so that some disastrous event can be prevented if there might be chances of its creation.
These special error scenarios which have been encountered or predicted in advance are known as exceptions. Handling these special error scenarios gave rise to a new field in computer science which is known as Exception Handling.
In the context of “Errors vs Exceptions”, Errors are system-level issues that cannot typically be recovered from, while Exceptions are application-level anomalies that can be caught and handled by the developer.
The image shown below depicts the hierarchy of Error and Exception classes.
Which came first, error or exception?
Errors came first, the people started analyzing the reasons behind errors, which gave rise to exceptions.
Definition of Exception
An Exception is the occurrence of an event that can disrupt the normal flow of the program instructions.* Exceptions can be caught and handled in order to keep the program working for the exceptional situation as well, instead of halting the program flow. If the exception is not handled, then it can result in the termination of the program. Exceptions can be used to indicate that an error has occurred in the program.
When an exception occurs, it creates an exception object. It holds information about the name and description of the exception and stores the program's state when the exception occurred.
The below image shows the flow of an exception.
There are 2 types of Exceptions:
- Checked Exceptions
- Unchecked Exceptions
Checked Exceptions
Exceptions that occur and can be detected at compile time are known as Checked Exceptions. These exceptions prevent the program from running, and so they must be handled by the programmer. Otherwise, the program will not get compiled.
For example, ClassNotFoundException, FileNotFoundException, IOException, SQLException, etc.
Unchecked Exceptions
Exceptions that occur at the time of the program execution, i.e., at runtime, are known as Unchecked Exceptions. These exceptions are ignored at the time of compilation.
They occur due to the following main reasons:
- Invalid User Input
- Bugs in the program
- Improper usage of an API
- Memory limit exceeded
Examples of unchecked exceptions include ArrayIndexOutOfBoundsException, NullPointerException, IllegalArgumentException, ArithmeticException, NumberFormatException, etc.
Definition of Error
Error is an unexpected event that cannot be handled at runtime. Errors can terminate your program. Most of the time, programs cannot recover from an error. Errors cannot be caught or handled. They are generally caused by the environment in which the code is running. e.g, The image below is seen by almost all the computer users.
Handling an error is out of the scope of a program. It can be handled externally.
There are 3 types of Errors:
- Syntax Error
- Runtime Error
- Logical Error
Syntax Errors
Syntax Errors are those errors detected during the compilation phase by the compiler when your code does not follow the syntactical rules of the programming language you are using. e.g, missing semi-colon(s), missing parenthesis, using else if() block directly without using if block first, returning nothing from the function when the return type is some data type, say, int, etc.
Example
In the below example we are trying to find out if a number n is even or odd.
Output:
Explanation:
Since we have used else if and else condition blocks without providing an if condition block, which is a syntax error, so the compilation is terminated.
Runtime Errors
Runtime Errors occur during the execution of a program, due to lack of system resources, or due to irrelevant input by the user. The compiler has no idea whatsoever how to detect these kinds of errors. For example, dividing a number by 0, accessing an element from an array that is out of range, trying to convert an invalid string to an integer, out of memory error, etc.
Example
In the code below, we are trying to find the count of the odd numbers present in an integer array.
Output:
Explanation:
Since we have used 'i <= arr.length' and in java arrays have 0 based indexing, so arr[i] for i = arr.length is an illegal operation as no such index exists, which throws a runtime error.
Logical Errors
Logical Errors are those errors where the program returned incorrect results when you were expecting the desired result. These occur due to some mistake in the code logic made by the programmer. The compiler cannot detect these errors. The user can just understand them after seeing the output. These are also known as Semantic Errors.
For example, When the programmer writes mistakenly, if(i = 1) instead of, if(i == 1): This will change the program's narrative.
Example
In the below example, we are trying to find out the XOR (Exclusive OR) of all elements from 1 to 5.
Output:
Note:
The expected output is:
Xor of all numbers is: 1
Explanation:
The actual output is different from the expected output because in the above code, a semi-colon is used after the for loop, which ultimately leads to just incrementing i up to 6 when the condition breaks, and after that, it runs the statement of the xor operation, which just runs only once and that also for i = 6. Hence the output is 6, which should have been 1, which is the correct xor of all the numbers from 1 to 5.
Key Differences Between Error and Exception
Origin:
- Error: Arises due to system abnormalities.
- Exception: Arises from the application code.
Recovery:
- Error: Usually fatal and non-recoverable.
- Exception: Can often be recovered from using try-catch blocks.
Hierarchy:
- Error: Extends the Error class in Java’s class hierarchy.
- Exception: Extends the Exception class.
Examples:
- Error: OutOfMemoryError, StackOverflowError.
- Exception: IOException, NullPointerException.
Difference Between Error and Exception in Java
Error | Exception |
---|---|
An error cannot be handled at runtime. | An exception can be handled at runtime |
An error can occur both at compile time and during runtime. | Although all the Exceptions occur at runtime. But checked Exceptions can be detected at compile time. |
There are 3 types of Errors: Syntax Error, Runtime Error and Logical Error | There are 2 types of Exceptions: Checked Exceptions and Unchecked Exceptions |
An error has the capacity to terminate your program and maybe your system as well. | An exception has the capacity to distract the normal flow of the program and change its direction to somewhere else when an exceptional case has occurred. |
An Error is such an event that no one can control or guess when it is going to happen. | An Exception can be guessed, handled, and utilized in order to change the original flow of the program. |
An Error can be thought of as an explosion that happens when there is no defense or checks against a particular failure condition. | An Exception can be thought of as a last line of defense to prevent errors. |
Examples of Exception
- Checked Exception: In the below example, we are using the sleep() function of the thread class, which throws a checked exception named InterruptedException, but we have not knowingly handled the exception.
Output:
Explanation:
As the method sleep() of Thread class requires throwing an InterruptedException, and we have not thrown it or used try-catch block to handle it, so the program terminated abruptly with the above error.
- Unchecked Exception: In the below example, we perform division by 0, which is an illegal operation.
Output:
Explanation:
Since division by 0 is an illegal operation, which should be handled by throwing an ArithmeticException, the program terminated with the above error message, as it requires the programmer to handle such divisions using ArithmeticException. Use this Compiler to compile your Java code.
Conclusion
- Errors occur at compile time and run time, which can terminate the compilation or execution.
- Exceptions occur only at run time, just that checked exceptions can be detected at compile time.
- Errors are also unchecked like Runtime Exceptions.
- Exceptions provide you the opportunity to make your program run in normal flow.
- Code should be in such a way that it has very few errors and a high number of managed exceptions.
- Error is an irrecoverable condition. However, we can recover from an exception.