Types of Exception in Java
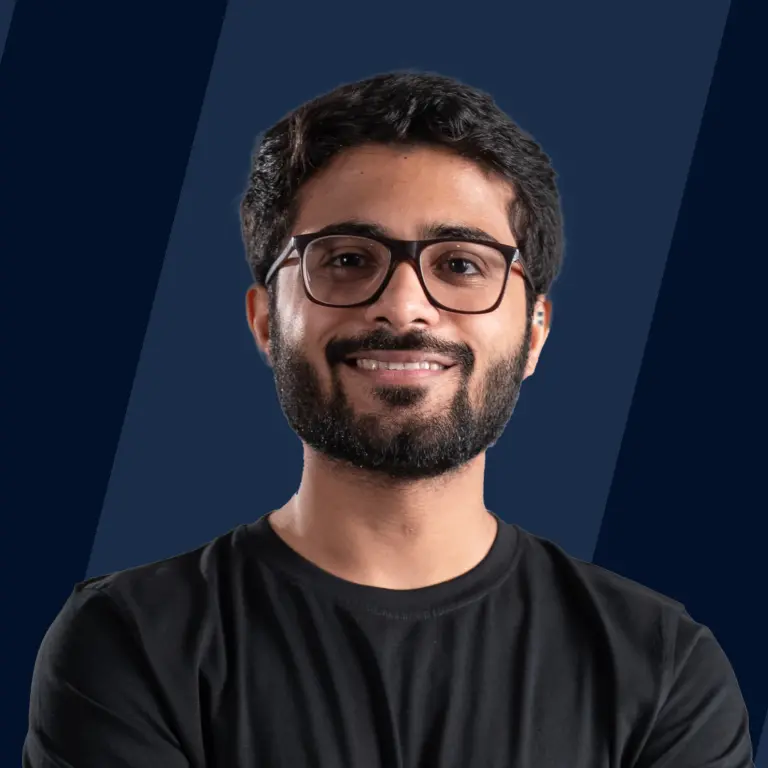
An exception in Java is an unpredicted condition that arises during the execution of the code either at compile-time or at run-time. It can abruptly affect the flow of our program by terminating the execution of the code. It can be an error or bug in code.
All the exceptions are handled by the predefined parent class known as java.lang.Exception in Java. The Throwable class has Exception and Error as its subclass. Exceptions are recoverable and can be recovered using try-catch blocks or throws keywords.
There are mainly two types of exception in Java:
- Built-in Exception
- User Defined Exception
Java Built-in Exceptions
Built-in Exceptions are those exceptions that are pre-defined in Java Libraries. These are the most frequently occurring Exceptions. An example of a built-in exception can be ArithmeticException; it is a pre-defined exception in the Exception class of java.lang package. These can be further divided into two types:
- Checked Exception
- Unchecked Exception
Checked Exceptions
Checked exceptions are caught at compile time, indicating potentially recoverable errors. The compiler enforces handling them before runtime. For instance, accessing a missing file like "file.txt" can throw a FileNotFoundException, which can be handled using the throws keyword to specify potential exceptions at compile time.
Class Not Found Exception
The ClassNotFoundException occurs when the Java Virtual Machine cannot locate a required class, typically triggered by functions like Class.forName() or ClassLoader.loadClass().
Code:
Output:
Explanation: The Class.forName() function returns the object of the class or interface whose name is passed in the parameter as a string. Now, if there is no class with the given name, then this will cause the ClassNotFoundException and terminate the execution of our code.
NoSuchMethodException
A NoSuchMethodException in Java occurs when you attempt to invoke a method on an object, but the method doesn't exist within the class or interface of that object.
Code:
Output:
Explanation: In this example, the nonExistentMethod() does not exist in the String class. When the main method tries to call it on the str object, a NoSuchMethodException is thrown.
Interrupted Exception
InterruptedException is raised when a thread is interrupted while waiting, sleeping, or processing. In Java, threads are used to enhance code efficiency by executing multiple tasks concurrently. This exception occurs when a thread's execution is disrupted while it's paused or waiting.
Code:
Output:
Explanation: Here, we first made an object of the class and then used the threads to start the execution. While the thread is occupied with the task of sleep, we interrupt it using the interrupt function. This causes the InterruptedException since the thread is disturbed.
IO Exception
The IOException is a frequently encountered exception in programming, typically arising from input or output discrepancies. It indicates a failure or interruption in input-output operations and can be handled using "throws" or will result in a compile-time error if not addressed.
Code:
Compile Time Exception:
The possible exception of type java.io.FileNotFoundException needs to be handled.
Explanation: Since opening the file can cause IOExceptions, the compiler gives a compile-time exception, and the code is not compiled.
AssertionError
This type of exception is typically thrown when an assertion made by the programmer fails at runtime.
Code:
Explanation: In this example, if the age variable is not greater than or equal to 18, the assertion will fail, and an AssertionError will be thrown with the message "Age must be at least 18".
Instantiation Exception
InstantiationException occurs when attempting to create an instance of a class that cannot be instantiated, such as abstract classes or interfaces, using the newInstance method. This exception is thrown at compile time, often encountered when instantiating abstract classes.
Code:
Compile Time Exception
The possible exception of type java.lang.InstantiationException must be handled.
Explanation: Here, we use the forName function (discussed in ClassNotFoundException) to create an instance (object) for the class. While doing so, the newInstance function might throw InstantiationException, which is why the compiler gives a compile time error.
SQL Exception
SQLException is thrown if there is an error in database access or other database errors.
Code:
Output:
Explanation: Here, we are using the getConnection method to access a database, but since the URL is not accessible, the code throws an SQLException.
FileNotFoundException
FileNotFoundException is thrown when we try to access a file in a directory and the file is not found. Let us see an example of a code throwing this error:
Code:
Output:
Explanation: Here, we are trying to access a file using the File class. But the file is not present in our system. We have used throws to suppress the compile time error. This code throws the FileNotFoundException while executing since the file is not found.
Unchecked Exceptions
An Unchecked Exception is an exception that occurs during runtime, often due to logical errors or improper usage of functions. These exceptions, also known as Runtime Exceptions, don't require explicit declaration using the throws keyword and can lead to bugs or unexpected behavior in code. Arithmetic Exception, such as division by zero, is a typical example of an unchecked exception.
Let us discuss some unchecked exceptions in detail:
Arithmetic Exceptions
An ArithmeticException is thrown when the code does the wrong arithmetic or mathematical operation while executing. Divide by 0 is the most common type of wrong mathematical operation.
Code:
Output:
Explanation: Since we are trying to divide 10 by 0, we are causing a mathematical error. This is predefined in the ArithmeticException of Exception class in Java. Hence, the code is throwing the exception.
NumberFormatException
A NumberFormatException in Java occurs when you try to convert a string into a numeric value (like an integer or a floating-point number). Still, the string does not represent a valid number format. This exception typically happens using methods like Integer.parseInt() or Double.parseDouble().
Code:
Output:
Explanation: In this example, we're trying to parse the string "abc" into an integer, which is not a valid number format. As a result, a NumberFormatException is thrown, and the program handles it by printing an error message.
StringIndexOutOfBoundsException
A StringIndexOutOfBoundsException is a common exception in Java that occurs when trying to access a character in a string using an index that is either negative or greater than or equal to the length of the string.
Code:
Output:
Explanation: In this example, since the index 10 is greater than the length of the string Hello, a StringIndexOutOfBoundsException is thrown
StackOverflowError
A StackOverflowError in Java occurs when a program recurses too deeply, exhausting the available stack space.
Code:
Output:
Explanation: In this example, the recursiveMethod is called repeatedly without a base case, causing the stack to fill up until it overflows and the StackOverflowError is thrown.
NoClassDefFoundError
A NoClassDefFoundError is an unchecked error in Java that occurs when a class that was available during compilation is not found at runtime.
Code:
Output:
ExceptionInInitializerError
An ExceptionInInitializerError in Java indicates that an exception occurred during the initialization of a class or interface. This typically happens when the initialization code throws an exception that is missed
Code:
Output:
IllegalArgumentException
An IllegalArgumentException is an unchecked exception in Java that occurs when a method receives an argument of an inappropriate type or value.
Code:
Output:
Class Cast Exception
Type casting involves changing the type of a variable or object. A ClassCastException occurs when attempting to cast an object to an incompatible type, such as trying to cast a String array to a List.
Code:
Output:
Explanation: Here, we are trying to convert the string array to ArrayList. Since this type of operation is not supported by the as List method, the code is throwing the ClassCastException.
Null Pointer Exception
NullPointerException is thrown by the JVM when we try to access a pointer pointing to Null (or Nothing). Pointing to Null means that no memory is allocated to that specific object.
Code:
Output:
Explanation: Here, we have defined the s as null. Since the length method does not support null strings, we get the NullPointerException.
ArrayIndexOutOfBounds Exception
ArrayIndexOutOfBounds is thrown when we try to access an array index that does not exist. Let us say that we have an array of size 10, and we try to access the 15th element. Then, JVM will throw an ArrayIndexOutOfBounds exception.
Code:
Output
Explanation: Here, we have defined an integer array of size 10. We are trying to access the 15th element, but since 15 is out of bounds, the code throws the ArrayIndexOutOfBoundsException.
ArrayStoreException
ArrayStoreException is thrown by JVM when we try to store the wrong type of object in the array of objects. Let us say we have an object array of double, and we try to store an integer. This will cause an exception at run time since a type mismatch exists. Let us look at the code for the same:
Code:
Output:
Explanation: Here, we have defined the array as a double type. Then, we are trying to store an Integer value in it, causing the ArrayStoreException.
IllegalThreadState Exception
The IllegalThreadStateException occurs when a thread is not in the appropriate state for specific operations, such as giving commands while it's sleeping. To handle potential InterruptExceptions, we can use the throws keyword in our code.
Code:
Output:
Explanation: Firstly, we have started processing the thread using the start method. Then we asked it to sleep for 1000 ms. Now, while it was sleeping, we again used the start method. This has caused the IllegalThreadStateException.
Java User-Defined Exceptions
In Java, besides using the Built-in Exception, if we want, we can create our Exceptions with messages and conditions for JVM to understand when to throw them. User-defined Exceptions are also called custom exceptions since they are not predefined and can be altered by the programmer. It is a beneficial tool to debug programs and handle edge cases without terminating the execution of the whole code.
To create a user-defined exception in Java, follow these steps:
- Create a new class that extends the Exception class:
- Optionally, define constructors for your exception class. For example, a default constructor:
Or a parameterized constructor to provide more details about the exception:
- To raise this exception, create an instance of your custom exception and throw it using the throw keyword:
By following these steps, you can create and use your custom exceptions in Java to handle specific situations that are not covered by built-in exceptions.
Code:
Output:
Explanation: Here, we have made a custom exception by extending the Java Exception class. We have also defined a String to pass a message to display if the exception is thrown. Since x is negative, the code is throwing the exception. It is also printing the message.
Difference between Checked and Unchecked Exception
Checked exception | Unchecked exception |
---|---|
Predicted exceptions that are handled before execution of the code | Unpredicted exceptions that are thrown at the run time due to logical errors. |
It does not include the run-time exceptions | It includes the run-time exceptions. |
We must address checked exceptions by the throws keyword or try-catch block(s). Otherwise, it will not compile. | We need to address unchecked exceptions, and the code will compile just fine. |
Examples: ClassNotFoundException, InterruptedException, IOException, InstantiationException, SQLException, FileNotFoundException | Examples: ArithmeticException, ClassCastException, NullPointerException, ArrayIndexOutOfBounds Exception, ArrayStoreException, IllegalThreadStateException |
Conclusion
- Exceptions are the unpredicted condition, which stops the further execution of the code.
- Built-in Exceptions are further divided into Checked and Unchecked Exceptions. Checked exceptions are checked at compile-time (or can be suppressed at compile-time by throws keyword), while unchecked exceptions are checked at runtime.
- Java also allows for user-defined exceptions, which can help a programmer handle the edge cases and debug the program.