What are the Primitive Data Types in JavaScript ?
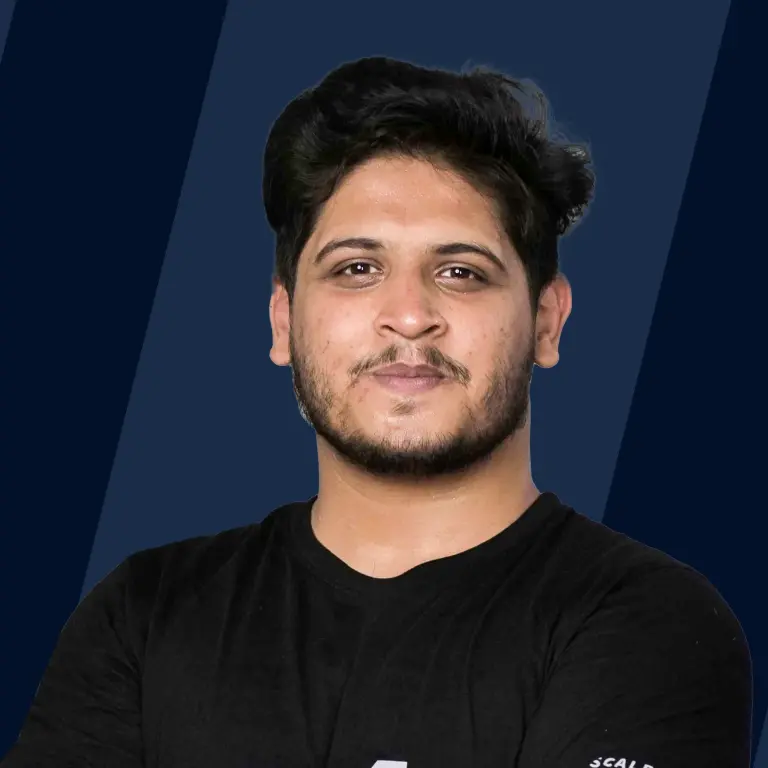
Every programming language has data types that are used for storing values in the variable to perform a logical or mathematical operation. Similarly, we have data types in javascript that can be broadly divided into two groups such as primitive and non-primitive.
The primitive data types have the following properties :
- Primitive data types are introduced in the ECMAScript standard.
- Primitives are built-in data types that are not flexible as user-defined data types.
- Every primitive data declared is stored in the stack identified by its names.
- Every primitive data type has a corresponding object except null and undefined.
- Primitives can't be modified once a value is assigned and are hence called immutable.
Immutable in primitives doesn't mean values can't be changed. It means the current value can't be modified.
Let us understand this with the following example.
Output :
From the example, we can understand that the primitive data types can't be modified but can be changed completely.
When the value is changed, it is stored in a new address in memory and the previous memory address is automatically freed(deleted) by a memory manager called garbage collector in javascript.
In our example, the value "immutable" is stored in an address starting in 2000. When the value is changed to "mutable", a new address 3000 is allocated for the updated value and the old address is freed.
Primitive Data Types in JavaScript
There are different types of primitive data types in javascript. Let us understand each one of them briefly with examples.
Boolean
-
The data type boolean can have two values, true and false.
-
It has a corresponding object called Boolean.
-
The boolean object is far more advantages than the primitive data type boolean as it can take different types of parameters such as string, number, null, undefined, object, or array and return either true or false based on the parameter. The different parameters are discussed below.
-
We can use the ! operator to invert the boolean value.
-
The data type of any data can be found using the typeof() property. The typof() method returns the data type of the value or object passed in the parameter. It has the following syntax,
Let us see an example for the boolean primitive :
The output for the above code will be :
The boolean object can have different values according to the parameter passed. The value can be either true or false.
null
- The null data type is used to represent an empty value. A variable can be marked as having a value using the null data type.
- The null data type can be used if we want to declare a variable that can be assigned a value later.
- We usually use the let or var keyword to declare a variable with a null value. The keyword const is not used to declare a null variable.
- The const keyword is used to declare a variable that can't be reassigned or modified later. The variable declared with the const keyword should be assigned a value on the declaration.
- If a variable is declared const and assigned a value null, we can't reassign it to another value and use it. So it is useless to declare a const variable as null.
The output will be :
There is a lot of confusion regarding whether the null data type is primitive because when using the typeof() operator to find the data type of the null object, the result is Object.
The output is Object which represents it as an object and objects are not primitive data types which are explained in the next section.
undefined
- The data type undefined is automatically assigned to the variable when they are declared without assigning any values.
- The variable declared with the const keyword can't have a data type of undefined.
- If we just declare a variable without a value using the const keyword, we will get an error.
Output :
The null and undefined data types differ in many aspects such as :
-
Null defines a variable with an empty value and undefined defines a variable with no value.
-
The data type null must be assigned by the user whereas, the undefined keyword is automatically assigned by the compiler.
-
In terms of values null and undefined are the same, but they differ in data types. The above statement can be verified using the == operator with compares values and the === operator with compares data types.
Output :
Number
-
The number data type is used to represent numerical values.
-
All Number values are stored in a format that occupies 64-bit of memory called Double-precision floating-point format
-
It also has an object called Number which has many methods and properties to handle numbers which are discussed in consecutive points.
-
We can store values between -( − 1) to ( − 1), which is called the safe limit for numbers. This is a property of the Number object and is represented by the Number.MAX_SAFE_INTEGER and Number.MIN_SAFE_INTEGER variables.
-
If the limit exceeds the safe limit we use a data type called BigInt which is discussed in the latter part of the article.
-
The safe limit depicts the range up to which the numbers can be represented exactly.
-
The highest and lowest values are represented by Number.MAX_VALUE and Number.MIN_VALUE.
-
The positive and negative infinity are represented by Number.POSITIVE_INFINITY and Number.NEGATIVE_INFINITY
-
There is also a property in the number object, Number.NaN which represents something that is not a number and this can be checked using the isNan() property.
The isNaN() returns true if the input is not a number.
Output for the above code will be,
The Number object has some useful properties such as toExponential(), toPrecision(),toFixed() and toString(). Let us look at each of them with examples
-
The toExponential() method is used to find the exponential notation of a number and has the syntax :
The default value for digits_after_decimal_point is the number of digits after the decimal point of the number.
For example,
-
The toPrecision() method is used to make the number precise according to the given number of digits and has the syntax,
The default value for the number_of_digits is the number of digits in the number. For example,
-
The toFixed() method is used to round a number according to the given number of digits and has the syntax,
The default value for the number_of_digits is 0. For example,
-
The toString() method is used to convert the number to a string and return the value and has the syntax,
For example,
BigInt
-
BigInt is a data type used to store numbers that are larger than the safe limit provided by the number data type.
-
BigInt is represented as numbers with a suffix of n . For example, let a =2n. In this example, the variable a is of type bigint.
-
BigInt is flexible as it can take as much space as it needs to store a number. This is also termed arbitrary precise.
-
A Bigint can also be created using the BigInt() property of the BigInt object.
-
The bigint values don't differ too much from real number values and hence can be called equal. Let us consider the following example,
Output :
String
- A string is the most used data type which represents a word or multiple words.
- Simply, a string is a combination of characters.
- There is also a String object in javascript which has many methods that are not present on the primitive data type string.
- The characters in the string can be accessed by using the character location as the index of the string.
- The first letter in the string is indexed 0, then the following letters are indexed continuously as 1,2,3, etc.
Consider the string "sample of". It is indexed as follows,
Note : Spaces are also indexed.
There are many methods present in the String object which can be used to modify a string object. These methods can't be used on the primitive data type string as it is immutable.
This can be visualized with an example :
Output :
The string object can be converted back to the original string using the valueOf() method.
The valueOf() method is present in every class and helps to convert the primitive objects back to the original primitive data type.
It has the syntax :
Consider the following example in which a string object and number object are converted to their primitive types string and number.
Output :
Symbol
-
Symbols are primitive data types in javascript introduced in the ECMAScript 2015.
-
Symbols are unique and can be used as keys for objects.
-
A function Symbol() is used to create a unique symbol that can't be reproduced by another function. The symbol method uses the following syntax to create a new symbol,
Let us consider an example,
Output :
-
The symbol object has a method called Symbol.for("key") that checks whether a symbol with the key already exists. If it does, then it just recreates the symbol, otherwise, a new symbol is created that can be reproduced by another Symbol.for() function.
Note : We can't reproduce a symbol created by the Symbol() method.
Since symbol has already been created with a key called sample, the Symbol.for() command searches for that symbol and returns it.
- We can use the Symbol.Keyfor(symbol) method to print the shared symbol's key.
This only works for shared symbols, that is symbols created using the Symbolfor() method.
Output for the above program will be sample , which is the key value of the symbol
Primitive Types are not JavaScript Objects
- Primitive types are the most basic features in javascript. Primitive data types do not object.
- So, primitive data types have no method or properties associated with them.
- Most primitive objects have a similar class in which methods and properties can be found like the string primitive which has the String class.
- These classes are enveloped around the primitive objects and when a method is not in primitive data type, it is fetched from the class.
Browser Compatibility
- The primitive data types are supported in most browsers as they are the basic building blocks of javascript.
- The Internet explorer browser doesn't support the BigInt and Symbol data types. It also doesn't support many properties of other primitive data types.
- The opera browser doesn't support a few methods in the string and bigint data types.
- There are some functions associated with some primitives such as eval() in string primitive which are not supported.
Learn more
This article gives an idea of primitive data types in javascript, but the real elegance of javascript lies in non-primitive data types such as an array, object, and Regex. You can learn more about these amazing data types in this article.
Conclusion
- Primitive data types are built-in data types and are immutable.
- There are different types of primitive data types for handling different types of values.
- The null and undefined data types are used to handle empty values.
- Primitive data types are not objects and don't have methods.
- Most primitive data types have wrapper class which has the required methods and properties for that data type.