What is XOR in Python?
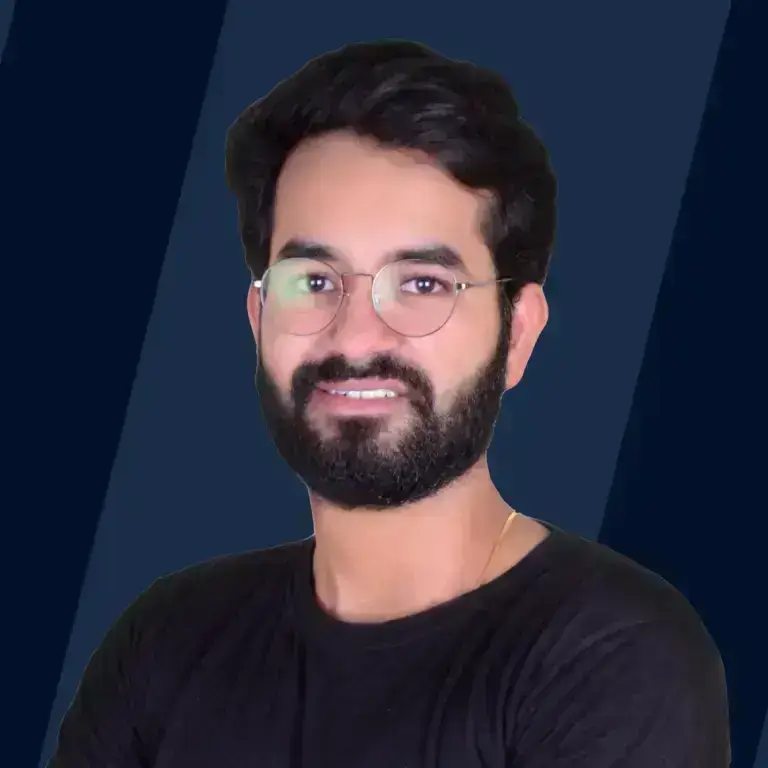
What is XOR in Python?
In Python, XOR is a bitwise operator that is also known as Exclusive OR.
It is a logical operator which outputs when either of the operands is (one is and the other one is ), but both are not , and both are not .
The symbol for XOR in Python is '^' and in mathematics, its symbol is '⊕'.
Syntax
The XOR operator is placed between two numbers or boolean values.
How to perform the bitwise XOR in Python?
In Python, we can perform the bitwise XOR operation using the "^" symbol. The XOR operation can be used for different purposes; XOR of two integers, XOR of two booleans, Swapping two numbers using XOR, etc.
We can also use the xor() function using the operator module in Python.
XOR ^ Operator between 2 integers
As XOR is a bitwise operator, it will compare bits of both the integers bit by bit after converting them into binary numbers.
Truth table for XOR (binary)
A | B | A⊕B |
---|---|---|
1 | 1 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
0 | 0 | 0 |
Code
Output
In the above example, we are finding the XOR of and , the result of which is . The XOR operator first converted both the numbers in their binary form and then compared their bits bitwise.
To understand the working of XOR operation better, let us find the XOR of 15 and 32 by comparing their bits:
The XOR of and is , i.e., .
Performing XOR on two booleans
XOR results when either of the operands are (one is and the other one is ) but both are not and both are not .
Truth table for XOR (boolean)
A | B | A⊕B |
---|---|---|
False | False | False |
True | False | True |
False | True | True |
True | True | False |
Code
Output
In the above example, we are finding the XOR of the boolean values ( and ). Use this Online Python Compiler to compile your code.
Swapping two integers using XOR without a temporary variable
The XOR swap algorithm can swap the values of two integers without the use of a temporary variable, which is normally required in other swapping algorithms.
Code
Output
In the above program, we are swapping two integers without using a temporary variable with the help of the XOR operator.
XOR in Python using Operator Module
We can also use the XOR operation using the xor() function in the operator module. The xor() function can perform XOR operations on integers and booleans.
Code
Output
The above example uses the operator.xor() function with booleans and integers.
Want to become a sought-after Python developer? Our comprehensive Python course is the key to achieving your dreams.
Learn more about bitwise operators
The bitwise operators are used to perform bitwise calculations on integers. The integers are first converted into binary numbers, and then the operations are performed bit by bit. The result is always in decimal format.
They can also be used with boolean values.
To learn more about bitwise operators, click here.
Conclusion
- XOR is a bitwise operator that is short for Exclusive OR.
- It returns when one and only one of the operands are or .
- XOR in Python is denoted using the "^" symbol.
- We can swap two integers without using a temporary variable with the help of the XOR operation.
- XOR operation can also be used with the help of the xor() function by importing the operator module.