Bitwise Operator in Python
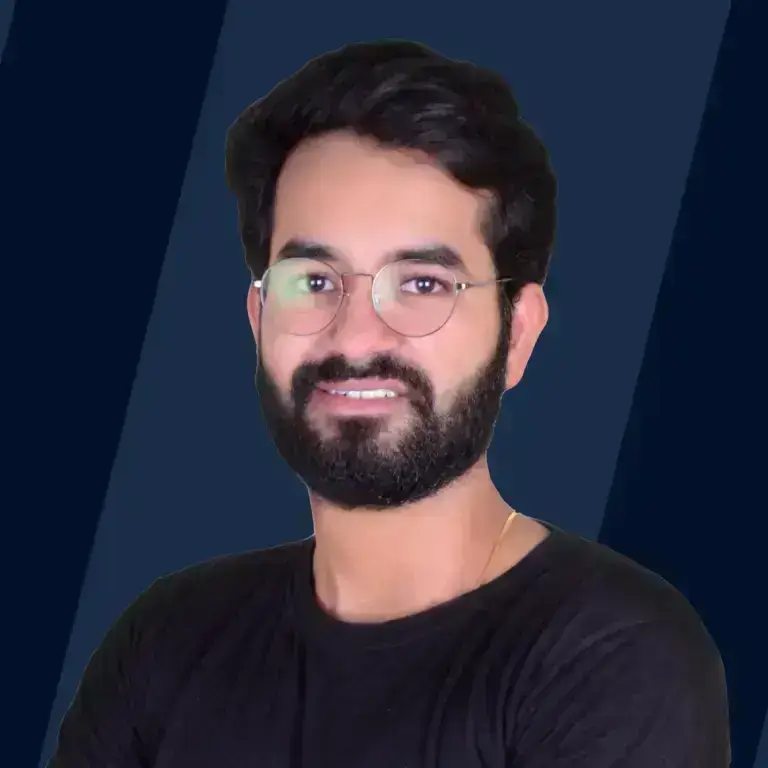
Operators play a crucial role in performing operations on values and variables, serving as special symbols for arithmetic and logical computations. The operands, on which operators act, contribute to these computations. In computing, information is stored as binary digits or bits, allowing Python's bitwise operators to manipulate data at a granular level. These operators prove useful in implementing algorithms like compression, encryption, and error detection, as well as controlling physical devices in projects such as Raspberry Pi. This tutorial guides you through using Python's bitwise operators for manipulating bits, binary data handling, bitmasks, and even digital watermarking.
What are Bitwise Operators in Python?
While studying python we do get intrigued by the Bitwise Operations in Python, but before we jump on to understanding the different operations that we can perform in Python, let's first understand what the Bitwise Operations generally mean.
Bitwise Operator in Python can be defined as performing the calculations on integers by first converting the integers to its binary format and then simply performing the operations on it bit by bit as the name suggests (bitwise operators). Then the result we get which is in binary format is converted back to decimal portion.
We mostly use the Bitwise operators in Python to compare the two integers which under operations gets converted to binary and then after the result is found, it again gets converted back to the decimal format to get the output in a more systematic and human-readable format.
Hence, we can also name these Python Bitwise operators as Binary operators. Binary refers to a numbering scheme in which the two possible output that one could get is 1 or 0.
Various Types of Bitwise Operators in Python
- Bitwise Logical Operators
- The Bitwise AND operator
- The Bitwise OR operator
- The Bitwise NOT operator
- The Bitwise XOR operator
- Bitwise Shift Operators
- The Bitwise Right Shift operator
- The Bitwise Left Shift operator
- Bitwise Operator Overloading
- Built-In Data Types
- Third-Party Modules
- Custom Data Types
The Bitwise Logical Operators in Python
The Bitwise Logical Operators can be defined as the Operator used for controlling the bits that are to perform Boolean logic on individual bits of an integer. The following table shows the different Bitwise Operators in Python. Let's dive deeper into understanding the concept in a better way.
It can also be considered to use the logical operators such as and, or, and not, but on a bit level.
DESCRIPTION | OPERATOR | SYNTAX |
---|---|---|
Bitwise AND | & | x & y |
Bitwise OR | | | x |
Bitwise NOT | ~ | ~x |
Bitwise XOR | ^ | x ^ y |
The Bitwise AND Operator:
The Bitwise Operator ‘AND’ in Python, returns value 1 if both the bits are 1, if not, it will return 0 meaning if either of the two bits are 0, then it shall return 0. It is represented by the & sign.
The Bitwise OR Operator
The Bitwise Operator ‘OR’ in Python, returns value 1 if either of the bits is set to 1, if not, it will return 0 meaning if the both the bits are 0, then it shall return 0. It is represented by the | sign .
The Bitwise NOT Operator:
The Bitwise Operator ‘NOT’ in Python, works by using the formula .
S + ~(S) = - 1 i.e., ~(S) = - ( 1 + S )
It is represented by the ~ sign It can also be represented as being 1’s complement of the given integer.
The Bitwise XOR Operator:
The Bitwise Operator XOR in Python, returns the opposite of the bit in the binary number.
To simply put it up, if the two bits are different that is one bit is 1 and the other is 0 then it will return 1.
If both the bits in the binary format of the number are the same hat is both are 1 or both are 0 then it shall return 0.
It is represented by the ^ sign.
Output:
The Bitwise Shift Operators in Python
Now as we know what are Logical Bitwise Operators in Python, Python also offers us Shift Operators or Python Bitwise Shift Operators.
As the name suggests we can use the Bitwise Shift Operators in Python to shift the bits of an integer right or left.
Mathematically, when we need to multiply or divide the number by 2 we make use of Bitwise Shift Operators to shift the bits of an integer in its binary form, left or right respectively.
Let's dive deep to understand both operators better:
DESCRIPTION | OPERATOR | SYNTAX |
---|---|---|
Bitwise right shift | >> | x>>y |
Bitwise left shift | << | x<< y |
The Bitwise Left Shift Operator:
The Bitwise Shift Operator ‘LEFT’ in Python can be used when we want to shift the integer to the left. The voids created after the number shifts to left can be filled up substituting 0.As when we shift the bits to the left side, the voids always come on the right and so we will always fill it with 0. The sign bits are not affected when using left shift operators.
This filling of voids can also be considered as having the similar effect of dividing the number with some power of 2.
Mathematically, It is represented by << sign.
The Bitwise Right Shift Operator:
The Bitwise Shift Operator ‘RIGHT’ in Python can be used when we want to shift the integer to the right. The voids created after the number shifts to right can be filled up substituting 0 in the case of a positive number and 1 in the case of a negative number.
This filling of voids can also be considered as having the similar effect of multiplying the number with some power of 2.
Mathematically, It is represented by >> sign.
Output:
The Bitwise Overloading Operators in Python
Now let us understand the concept of Operator Overloading in python. We use the '+' operator to add the two operands but in Python when we use the + operator we not only do the simple addition but it can also be used to join two strings and merge two lists.
This functionality of an operator to be able to perform extended functions over its defined operational meaning can be termed as Operator Overloading. For the example let us consider
When we do + (simple addition), it will return us 10 as in python the operator + is understood as an additional function.
But what if we want to concatenate the two strings?
Here it has taken the strings into account and applied the add method that is overloaded by the int class and str class and hence we can see the output as 'The House'.
As can be seen above the ability to overload and extend functionality beyond its defined meaning can be termed as Operator Overloading.
In Python, we might have observed that the same built-in operator tends to show different behavior for objects of different classes, which is the defined meaning of operator overloading. hence, Python provides an alternative way of implementation for its selected bitwise operators and lets us overload it for new data types. The idea for logical operators in Python was rejected.
Output:
Some Operators are Listed Below for Quick Reference
The Binary Operators:
Magic method | Operator |
---|---|
add(self,other) | + |
sub(self,other) | – |
mul(self,other) | * |
truediv(self,other) | / |
floordiv(self,other) | // |
mod(self,other) | % |
pow(self,other) | ** |
rshift(self,other) | >> |
lshift(self,other) | << |
and(self,other) | & |
or(self,other) | | |
xor(self,other) | ^ |
The Comparison Operators :
Magic method | Operator |
---|---|
LT(SELF,OTHER) | < |
GT(SELF,OTHER) | > |
LE(SELF,OTHER) | <= |
GE(SELF,OTHER) | >= |
EQ(SELF,OTHER) | == |
NE(SELF,OTHER) | != |
The Assignment Operators :
Magic method | Operator |
---|---|
ISUB(SELF,OTHER) | -= |
IADD(SELF,OTHER) | += |
IMUL(SELF,OTHER) | *= |
IDIV(SELF,OTHER) | /= |
IFLOORDIV(SELF,OTHER) | //= |
IMOD(SELF,OTHER) | %= |
IPOW(SELF,OTHER) | **= |
IRSHIFT(SELF,OTHER) | >>= |
ILSHIFT(SELF,OTHER) | <<= |
IAND(SELF,OTHER) | &= |
IOR(SELF,OTHER) | |= |
IXOR(SELF,OTHER) | ^= |
The Unary Operators :
Magic | Method |
---|---|
NEG(SELF,OTHER) | – |
POS(SELF,OTHER) | + |
INVERT(SELF,OTHER) | ~ |
The Three Broad segments in Operator Overloading in Bitwise Operator in Python
The Built-In Data Types:
We can define the Bitwise operators in Python for Built data types as performing the operations from set algebra ( intersection, union, symmetric difference as well as updating and merging dictionaries ) for the built-in data types:
• bool • int • edit
Consider s and t to be Python sets, then bitwise operators can be represented as below:
Set Method | Bitwise Operator Representation |
---|---|
s.union(t) | s |
s.intersection(t) | s & t |
s.update(t) | s |
s.symmetric_difference(t) | s ^ t |
s.symmetric_difference_update(t) | s ^= t |
s.intersection_update(t) | s &= t |
Let’s see them in action by assuming two boxes with different color papers:
We see that in Python for the Built-in data type 'diet' is only supported for bitwise OR, which works like a union operator which can be used to update a dictionary in place or merge two dictionaries into a new one.
The Third-Party Modules:
With Many popular libraries, including NumPy, Pandas, and SQLAlchemy, overload the bitwise operators for their specific data types. This is the most likely place you’ll find bitwise operators in Python because they aren’t used very often in their original meaning anymore. Things get more interesting with third-party modules that give the bitwise operators in Python entirely new dimensions and meanings.
let us consider an example, NumPy applies them to vectorized data in a pointwise fashion:
As can be seen above, we don't need to apply the same bitwise operator in python manually to each element of the array. This functionality cant be applied to ordinary lists in Python.
We know that Pandas uses NumPy behind the scenes, and they behave as we expected as it provides overloaded versions of the bitwise operators for its DataFrame and Series objects.
Sometimes we can see that in certain cases of SQLAlchemy provides a compact syntax for querying the database:
session. query(User) \ .filter((User. age > 40) & (User. name == "Doe")) \ .all()
As shown above, The bitwise AND operator (&) will eventually translate to a piece of SQL query. However, as for some IDE, it complains about the unpythonic use of bitwise operators when it sees them in this type of expression which is not obvious to find out. And in most cases, it immediately suggests replacing every occurrence of '&' with a logical 'and', which in turn pushes the code to stop working!.
We can see that for some programming languages like Java this kind of abuse gets prevented by disallowing operator overloading altogether whereas for Python this becomes a more liberal case and trusts the users that they know what they’re doing.
The Custom Data Types:
As the name suggests, the Custom data types can be defined as the ability to customize the behavior of Python’s bitwise operators where we have to first define a class, and then we can implement the methods in it. These methods are popularly known as magic methods. The Custom Data Types in Operator overloading is possible only on new data types and we cant redefine the behavior of the bitwise operators in python for the existing data types.
Now let's consider an example for a better vision of the concept :
Output:
Above we see that both print statements are returning the same value i.e. 12. the first print syntax is operating over two integers whereas in the second print syntax the 'int' class is calling the 'add' method to perform the addition on s and t. We can see how the class is calling the method as can be seen by the second print statement.
But, for the third print statement, we obtain no response and this is because the inbuilt 'int' class doesn't have the add method for the int and str to add up.
Here’s a quick run-through of special methods that let you overload the bitwise operators in python:
Expression | Magic Method |
---|---|
instance&value | .and(self,value) |
value&instance | .rand(self,value) |
instance&=value | .iand(self,value) |
instance|value | .or(self,value) |
value|instance | .ror(self,value) |
instance|=value | .ior(self,value) |
instance^value | .xor(self,value) |
value^instance | .rxor(self,value) |
instance^=value | .ixor(self,value) |
~instance | .invert(self) |
instance<<value | .lshift(self,value) |
value<<instance | .rlshift(self,value) |
instance<<=value | .ilshift(self,value) |
instance>>value | .rshift(self,value) |
value>>instance | .rrshift(self,value) |
instance>>=value | .irshift(self,value) |
Output:
FAQs
Q. What is the purpose of bitwise operators in Python?
A: Bitwise operators in Python are used to perform operations at the bit level, working with the individual bits of binary numbers. These operators are useful for tasks such as manipulating binary data, setting or clearing specific bits, performing bitwise calculations, or working with flags or bitwise representations of data.
Q. How can bitwise operators be used for manipulating flags?
A: Bitwise operators are commonly used for manipulating flags or boolean values represented by individual bits. By using bitwise AND, OR, and XOR operations, you can set, clear, toggle, or check the status of specific flags within an integer variable. For example, to set a flag, you can use the bitwise OR operation (|) to turn on the corresponding bit. To clear a flag, you can use the bitwise AND operation (&) with a complement mask. To toggle a flag, you can use the bitwise XOR operation (^) with a mask.
Q. How do I perform a bitwise shift operation in Python?
A: Python provides two shift operators: left shift (<<) and right shift (>>). The left shift operator (<<) shifts the bits of a number to the left by a specified number of positions, effectively multiplying the number by 2. The right shift operator (>>) shifts the bits to the right by a specified number of positions, effectively dividing the number by 2.
Conclusion
Summing up the important points to remember for Bitwise Operators in Python:
- The Bitwise Operators in Python can be defined as the implementation of operations on integers which are first converted into binary digits and then after the operation is executed, the output is given in decimal format.
- There are three different types of Bitwise Operators in Python:
a. The Bitwise Logical Operator consists of four different operators: AND, OR, NOT, and XOR.
b. The Bitwise Shift Operator consists of two different operators: Right and Left.
c. The Bitwise Operator Overloading consists of three different operators: Built-In Data Types, Third-Party Modules and Custom Data Types
- The Bitwise AND Operator: If both the bits are 1 it returns 1 else 0.
- The Bitwise OR Operator: If either of the bit is 1 it returns 1 else 0.
- The Bitwise NOT Operator: It returns one’s complement of the number.
- The Bitwise XOR Operator: If one of the bits is 1 and the other is 0 it returns 1 else returns 0.
- The Bitwise Right Shift Operator: As the name suggests it shifts the bits of the integer to the right and fills 0 on voids left. In case of a negative number, the voids are filled by 1.
- The Bitwise Left Shift Operator: As the name suggests it shifts the bits of the number to the left and fills 0 on voids right.
- Operator Overloading can be defined as the ability of the operators to work as an extended functionality beyond its original defined meaning.