What is Binary Operator in C?
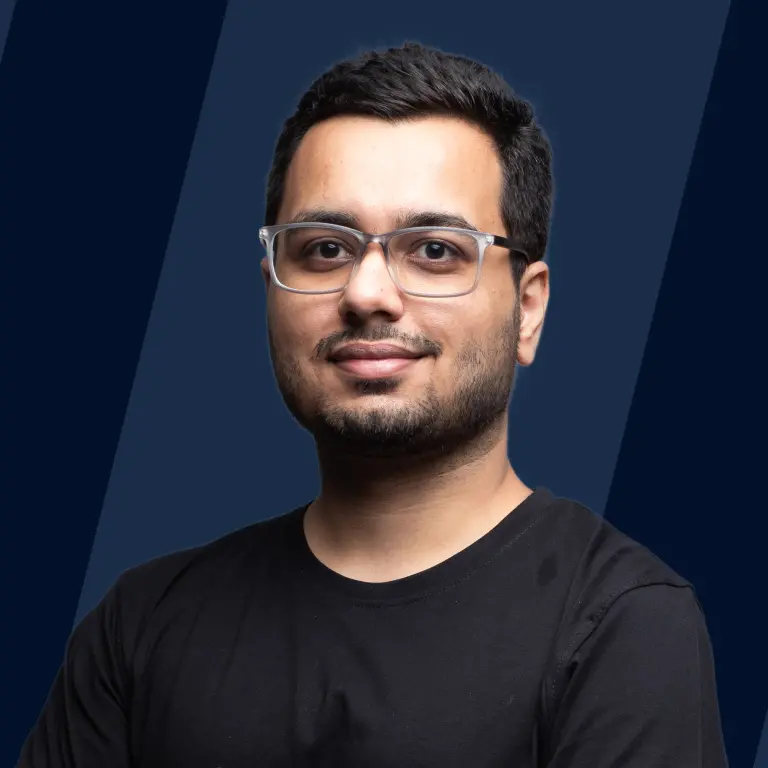
A Binary operator is an operator that operates on two operands to produce a new value (result). Most common binary operators are +, -, *, /, etc. Binary operators in C are further divided into -
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Assignment Operators
Syntax of Binary Operator
Binary operators are presented in the form -
Where Operand_1 and Operand_2 can be any variable, numeric values.
List of Binary Operators in C
Arithmetic Operators
Arithmetic operators are those binary operators in C that are used to perform basic arithmetic operations like addition, subtraction, multiplication, division, and modulus operation. Here we will be seeing examples of different arithmetic operators, if you want to learn arithmetic operators in detail please visit Arithmetic Operators in C
Addition Operator
Syntax - operand_1 + operand_2
Example -
Output -
Subtraction Operator
Syntax -
Example -
Output -
Multiplication Operator
Syntax -
Example -
Output -
Divison Operator
Syntax -
Example -
Output -
Note that here is 3 because we are performing integer division which rounds down the result to the nearest integer value.
Modulus Operator
Syntax -
Example -
Output -
Since 1 remains as the remainder when 10 is divided by 3, hence the output is 1.
Relational Operators
Relational operators are those binary operators in C that are used to compare any two entities, like two integers, characters, floating point numbers, etc. The result produced by them is either 1 or 0, where 1 means the comparison is true and 0 means it is not false. To see more details about Relational Operators please click here
The equal == operator
Syntax -
This binary operator in C evaluates to true if operand_1 is exactly equal to operand_2 otherwise it evaluates to false.
Output -
The not equal != operator
Syntax -
This binary operator in C evaluates to true if operand_1 is not equal to operand_2 otherwise it evaluates to false.
Output -
The Less Than < Operator
This binary operator in C evaluates to true if operand_1 is strictly less than operand_2 otherwise it evaluates to false. Syntax -
Output -
The Less Than or Equal <= Operator
This operator evaluates to true if operand_1 is less than or equal to operand_2 otherwise it evaluates to false. Syntax -
Output -
The Greater than > Operator
This operator evaluates to true if operand_1 is strictly greater than operand_2 otherwise it evaluates to false. Syntax -
Output -
The Greater Than or Equal >= Operator
This operator evaluates to true if operand_1 is greater than or equal to operand_2 otherwise it evaluates to false.
Syntax -
Output -
Logical Operators
We have a set of three logical operators in C language that helps us to combine the result of two or more logical conditions or boolean values. Out of those three AND, OR, and NOT only the first two are binary operators.
Logical AND Operator
Syntax
The Logical AND operator is represented by && in the C programming language. It evaluates to true if all the conditions in the boolean expression are true.
Output -
Logical OR Operator
Syntax -
The Logical AND operator is represented by || in the C programming language. It evaluates to true if at least one of the conditions in the boolean expression is true.
Output -
So this was all about Logical operators, to get more insights about Logical operators in C programming please visit this.
Assignment Operators
Syntax -
Assignment operators are those binary operators in C that assign the values or result of an expression to a variable and the value on the right side must be of the same data type as the variable on the left side. The most commonly used assignment operator is = operator, for example, x=4 means the value 4 is assigned to variable x.
Assignment
Output -
Addition and assignment
Syntax -
Addition and assignment operator is denoted by += sign, it adds the value of right operand to the left operand. Also, this operator can be further simplified, for example, x += 4 can be simplified to x = x + 4.
Output -
Subtraction and assignment
Syntax -
Subtraction and assignment operator is denoted by -= sign, it subtracts the value of right operand from the left operand. This operator can also be further simplified, for example, x -= 4 can be simplified to x = x - 4.
Output -
Multiplication and assignment
Syntax -
Multiplication and assignment operator is denoted by *= sign, it multiplies the value of right operand to the left operand. This operator can also be further simplified, for example, x *= 4 can be simplified to x = x * 4.
Output -
Division and assignment
Syntax -
Division and assignment operator is denoted by /= sign, it divides the left operand by the value of right operand. This operator can also be further simplified, for example, x /= 4 can be simplified to x = x / 4.
Output -
Note that it is integer division hence the result is rounded down to the nearest integer.
Remainder and assignment
Syntax -
Remainder and assignment operator is denoted by %= sign, it assigns the value of remainder to left operand which one will get by dividing the left operand by right operand. This operator can also be further simplified, for example, x %= 4 can be simplified to x = x % 4.
Output -
Note that it is integer division hence the result is rounded down to the nearest integer. To learn more about the Assignment operators, please have a look at "Assignment Operators in C"
Bitwise Operators
Bitwise operators are those binary operators in C that are used to manipulate bits in different ways. They are equivalent to how we use mathematical operations like (+, -, /, *) among numbers, similarly, we use bitwise operators like (|, &, ^, <<, >>, ~) among bits.
Bitwise AND Operator
Syntax -
Bitwise AND operator simply calculates the AND of two operands. For example 4 & 5 = 4.
Output -
Bitwise OR Operator
Syntax -
Bitwise OR operator simply calculates the OR of two operands. For example 4 | 5 = 4.
Output -
Bitwise XOR Operator
Syntax -
Bitwise XOR operator simply calculates the XOR of two operands. For example 4 ^ 5 = 4. Note that ^ does not denote raised to the power, instead it denotes XOR operator.
Output -
Bitwise Shift Left Operator
Syntax -
The shift left operator << shifts the bit pattern of an integer value by a specified number of bits to the left. For example, x >> 3 shifts bits in x three times to right side.
Output -
Bitwise Shift Right Operator
Syntax -
The shift right operator >> shifts the bit pattern of an integer value by a specified number of bits to the right. For example, x << 3 shifts bits in x three times to right side.
Output -
Learn More
So, this was all about the Binary Operators in the C programming language. There exist different types of operators in C programming hence, we would strongly recommend you to learn about them with the below link Operators in C
Conclusion
- Binary Operators in the C programming language are ones that operate on two operands.
- Arithmetic Operators, Relational Operators, Logical Operators, Assignment Operators, and Bitwise Operators fall under the category of Binary Operators.