C++ Program to Convert Binary Number to Decimal
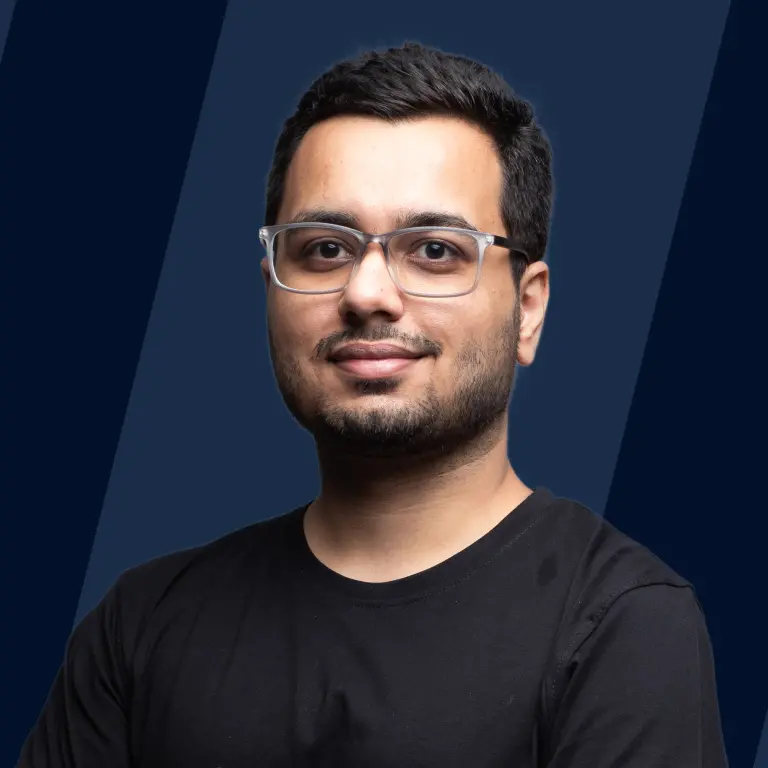
Overview
In this article, we will discuss how to convert a number from binary format to decimal format in the C++ programming language.
For example, 100 in binary when converted to a decimal number is 4. Binary numbers are composed of only 0 and 1, whereas decimal numbers are composed of digits from 0 to 9.
Algorithm to Convert Binary Number to Decimal Number
Algorithm to Convert Binary Number to Decimal Number:
-
Initialize a variable decimal to 0. This variable will store the decimal equivalent of the binary number.
-
Initialize a variable base to 1. This variable will represent the current position's weight in the binary number.
-
Starting from the rightmost digit of the binary number (the least significant bit) and moving to the leftmost digit (the most significant bit), do the following steps for each digit:
a. Extract the current binary digit (0 or 1).
b. Multiply the extracted digit by the current base value.
c. Add the result to the decimal variable.
d. Double the base value, which simulates moving to the next position in the binary number (i.e., shifting left).
-
Continue these steps until you have processed all the binary digits.
-
The final value of the decimal variable will be the decimal equivalent of the binary number.
Example:
Let's convert the binary number 1101 to its decimal equivalent using the algorithm:
-
Initialize decimal = 0 and base = 1.
-
Start from the rightmost digit (LSB) of the binary number (1):
- Extract 1, multiply by base (1 * 1 = 1), and add to decimal.
- decimal = 1.
-
Move to the next digit (0):
- Extract 0, multiply by base (0 * 2 = 0), and add to decimal.
- decimal = 1.
-
Move to the next digit (1):
- Extract 1, multiply by base (1 * 4 = 4), and add to decimal.
- decimal = 5.
-
Move to the leftmost digit (MSB) of the binary number (1):
- Extract 1, multiply by base (1 * 8 = 8), and add to decimal.
- decimal = 13.
So, the decimal equivalent of the binary number 1101 is 13.
Program to Convert Binary to Decimal in C++
Without Function
Here's a C++ program to convert a binary number to its decimal equivalent without using a separate function:
Output Explanation:
Let's consider an example where the user enters the binary number "1101":
-
The program prompts the user to enter a binary number.
-
The binary number is stored as a string variable called binary.
-
The program initializes decimal to 0 and base to 1. These variables are used to calculate the decimal equivalent.
-
The program enters a loop that iterates through the binary number from right to left (from the least significant bit to the most significant bit).
-
For each digit in the binary number, it checks if the digit is '1'. If it is, the program adds the current base value to the decimal variable.
-
After processing each digit, the base is doubled to simulate moving to the next position in the binary number (i.e., shifting left).
-
Finally, the program prints the calculated decimal equivalent.
Example Output:
If the user enters "1101" as the binary number, the program will output:
Complexity:
- Time Complexity: The time complexity is O(n), where n is the number of binary digits in the input. The program iterates through each digit once.
- Space Complexity: The space complexity is O(1) because it uses a fixed amount of memory regardless of the input size.
Using String Variables
To convert a binary number to a decimal number in C++ using string variables, you can follow the following program. This method involves treating the binary number as a string and parsing it character by character to calculate the decimal equivalent.
Output Explanation:
Let's say we input the binary number "1101" into the program:
The program first prompts the user to enter a binary number. In this case, "1101" is provided.
-
The binaryToDecimal function processes the binary string from right to left. Starting from the rightmost digit (1), it checks if the digit is '1'. If it is, it adds the appropriate decimal value to the result. In this case, 1 is added.
-
Then, it moves to the next digit (0), which doesn't contribute to the decimal value, so nothing is added.
-
It continues this process for the subsequent digits (1 and 1), adding 4 and 8 to the result, respectively.
-
The final result is 13, which is the decimal equivalent of the binary number "1101."
Complexity:
-
Time Complexity: The time complexity is O(n), where n is the length of the binary string. The program processes each digit in the string once.
-
Space Complexity: The space complexity is O(1) because it only uses a few integer variables and string variables that don't depend on the size of the input.
Using std::bitset Class
You can convert a binary number to a decimal number in C++ using the std::bitset class from the C++ Standard Library. This class makes the conversion process quite straightforward. Here's the code:
Output Explanation:
Let's walk through the code and provide an example with its explanation:
-
The program prompts the user to enter a binary number.
-
The user enters the binary number (e.g., "1101").
-
The binary number entered by the user is stored as a string in the variable binaryInput.
-
The std::bitset class is used to create a bitset with 64 bits. You can adjust the number of bits depending on your needs or use a fixed number if your binary numbers have a specific size.
-
The binary string stored in binaryInput is assigned to the binary bitset.
-
binary.to_ulong() is used to convert the binary bitset to an unsigned long integer, representing its decimal equivalent. This is the key step in the conversion.
-
The program then prints the decimal equivalent.
Example:
Let's assume the user enters the binary number "1101." The output will be:
Complexity:
- Time Complexity: The time complexity is O(n), where n is the number of bits in the binary number. The std::bitset class efficiently handles the conversion.
- Space Complexity: The space complexity is O(1) because the storage used is constant and does not depend on the input size.
Binary to Decimal using Function
Here's a C++ program to convert a binary number to its decimal equivalent using a function. This program takes a binary number as input and returns its decimal representation.
Code:
Output:
Here's an example of the program's output:
Output Explanation:
In this example, the program takes the binary number 1101 as input. The binaryToDecimal function converts it to its decimal equivalent, which is 13. The program then displays the result.
Complexity:
-
Time Complexity: The time complexity of this program is O(log2(N)), where N is the input binary number. It iterates through each digit of the binary number, and the number of iterations is proportional to the number of digits in the binary number.
-
Space Complexity: The space complexity is O(1) because it uses a constant amount of memory for variables and does not depend on the input size.
Binary to Decimal using Function using predefined functions
Here's a C++ program that converts a binary number to a decimal number using predefined functions like stoi to convert the binary string to an integer. This program also includes the complexity analysis for each part.
Output Explanation:
-
The program asks the user to input a binary number as a string.
-
It then calls the binaryToDecimal function with the entered binary string.
-
The binaryToDecimal function converts the binary string to an integer using std::stoi with a base of 2 (indicating binary representation).
-
The decimal equivalent is calculated and stored in the decimal variable.
-
The program prints the decimal equivalent.
Sample Output:
Let's say you input the binary number "1101" as the input:
Complexity Analysis:
-
Input: Taking the binary input requires O(n) time, where 'n' is the number of bits in the binary number.
-
Conversion (std::stoi): Converting the binary string to an integer using std::stoi takes O(n) time because it needs to parse each character in the binary string.
-
Output: Printing the decimal equivalent takes constant time, O(1).
Overall, the time complexity of this program is O(n) due to the conversion step, where 'n' is the number of bits in the binary number. The rest of the program has minimal impact on the overall complexity.
Conclusion
- In this article, we learned how to convert a Binary to decimal in C++. To convert a binary number to a decimal, we traverse the given binary number in reverse form
- Logic Behind the conversion of binary numbers to decimal. Every digit in a binary number is multiplied with its base raised to the power-dependent on its position in a binary to decimal conversion.
- Implementation of the program to convert binary to decimal.
- Dry Run or working of Code in both iteration and tabular format.
- Code Implementation of Conversion using User-defined Function.
- Code Implementation of conversion using Pre-defined Function i.e. std::stoi.