C++ Program to Convert Decimal to Binary
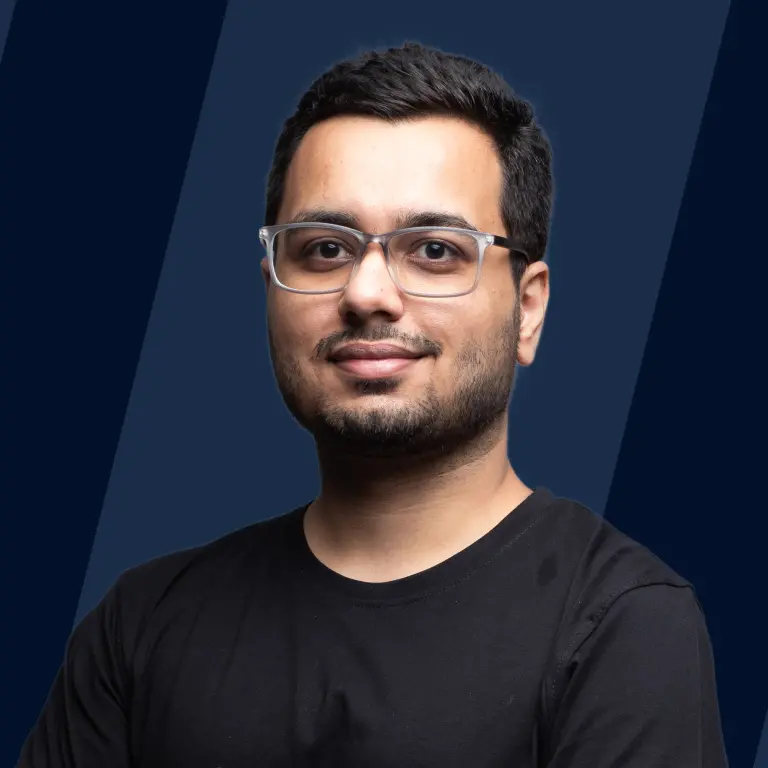
A Decimal number is a number that is represented in the base-10 numeral system using the digits 0, 1, 2, 3, 4, 5, 6, 7, 8, and 9. A Binary number is a number represented in the base-2 numeral system using the digits 0 and 1. Decimal to Binary in C++ can be achieved with or without using an array. Predefined functions can also be used to convert Decimal to Binary in C++.
Algorithm for Decimal to Binary Conversion in C++
Now since you know decimal numbers as well as binary numbers, let's learn how we can convert a decimal number n to a binary number.
- Divide the number n by 2 and store the remainder in the array using the modulus operator %.
- Divide the number by 2 using the division operator /.
- Go to step 1 while the number n is greater than 0.
- Reverse the array that is storing the remainder; this reversed array is the binary representation of the number n.
Let's understand the above algorithm using the number 13.
The highlighted part in the above image is the array that stores the remainder of the number n. Reversing this array will result in 1101, the binary representation of the number 13. Notice here in the number by 2, we are taking only the integer part of the number, and in the last operation, n becomes 0. Therefore, we do not store the result of n % 2 in the array.
C++ program to convert Decimal Number to Binary Number using Array
Output:
We store the remainder result in the array arr, and in the end, we print the array in reversed order.
C++ Program to Convert Decimal Number to Binary Number Without using Array
Output:
This time instead of using an array, we are maintaining counter i; this counter keeps getting multiplied by 10, and we add the multiplication of i and remainder to our answer since our answer can exceed the 32-Bit memory of an integer that's why we are using a long long.
C++ Program to convert Decimal Number to Binary using predefined functions
The itoa function in C++ converts an integer value to a null-terminated string with the given base and stores the result in the provided str parameter.
Syntax
Parameters
val It is the value that will be converted to the string. str It is the Array that will store the null-terminated string result. base The base is used to represent the value as a string. It ranges from 2 to 36, where 16 means hexadecimal, 10 means decimal, 8 means octal, and 2 means binary.
Return Value
It returns the pointer to the null-terminated string.
Example of itoa to Convert Decimal Number to Binary in C++
Output:
This is because the itoa function is not a standard library function in gcc and C++ 11. Instead of itoa, to_string is used, but to_string doesn't provide us the option to specify the base of the result string.
Conclusion
- A Decimal number is a number represented in the base-10 numeral system, while a Binary number is a number represented in the base-2 numeral system.
- We can convert a binary number to a decimal number by multiplying the digits of the binary number by the corresponding power of 2.
- To convert a decimal number to a binary number, store the number % 2 in the array and divide the number by 2 using the division/operator, repeat the process while the number is greater than 0, and in the end, reverse the remainder array.
- itoa function can be used to convert a decimal number to a binary number, but it is not a standard function in gcc and C++ 11.
- bitset can be used to convert a decimal number to a binary number, but it comes with the limitation of specifying the size of the bitset at compile time.