Program to Check if String is Empty in Python
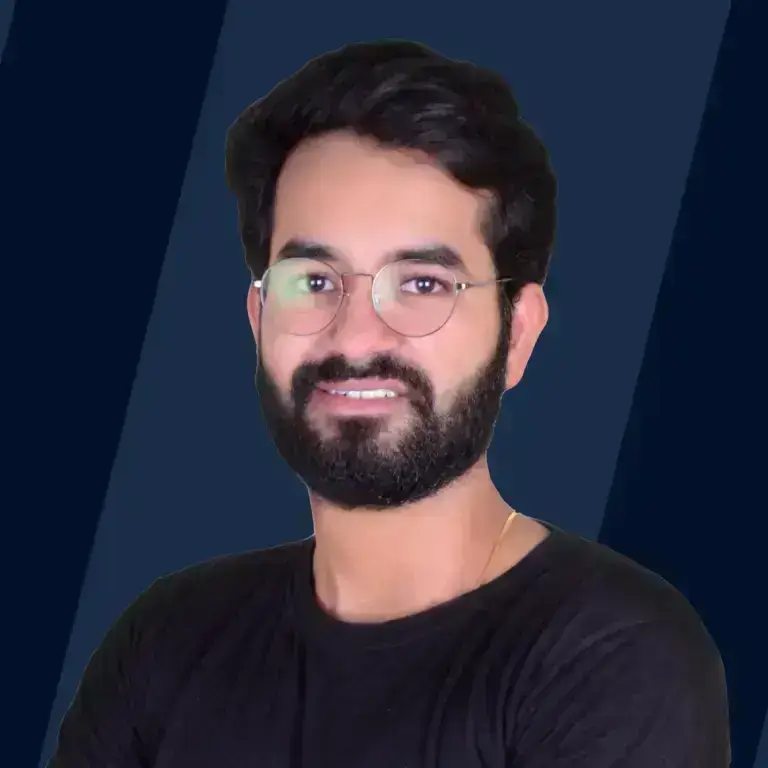
Overview
When we work with strings in Python we know that they are immutable and hence handling the strings while we perform operations becomes tricky. A string with spaces is referred to as an empty string having a non-zero size. With the below article, we shall understand how we can check if a string is an empty Python and explore the various methods with elaborate explanations of the code example.
Empty String in Python
Before we talk about checking if the string is empty in Python, let us understand the problem statement in depth. When applying for any examination, you fill out an application form. This application sometimes requires you to mandatorily fill data into an input field where these empty spaces are marked with an asterisk *. When a user skips the mandatory field and fills the next field and continues to submit or save the application form, a warning popup is always seen on the window. This warning is something like 'one or more of the mandatory fields have not been filled.
One question might pop up in your head like 'How is this implemented in the backend?' or 'How does the application check if the string is empty Python' that is, the data entered by the user is empty or filled.
Well to answer that question, we shall start by understanding the string in Python. A string in Python is an immutable or unchangeable sequence of characters. The string in Python is always written within the single, double, or triple(for multiline comments) inverted quote. Also, a string that contains only whitespaces is usually not considered empty. These strings contain the size of the non-zero value.
Creating an empty string can be in 'n' a number of ways but the cleanest and easiest way to do so is by simply using either single, double, or triple quotes. Whereas you can also implement the string constructor function str() to create an empty string in Python. The below code shows what an empty string looks like.
Code:
All the strings from 1 to 4 elaborate on the multiple ways of representing an empty string in Python.
Another question that must be addressed is if a string consisting of whitespaces is considered an Empty string or not in Python.
Code:
Well, technically this isn’t an empty string in Python. But from a practical viewpoint, this string is empty. Hence, we have a method that considers a string whitespaces as empty and hence we can check if a string is empty in Python or not, while other methods that we shall be discussing considered a string with white spaces as not empty.
Modifying or performing different operations on the string after defining it can get tricky. Well as we move along the article we shall learn how to perform different operations on strings. Be it the len() method or the not operator where it considers the whitespace as an element of the string. As strings in Python cannot be modified once they’re created, hence it is useful to check if a string is empty or not. Let us explore those operations as we move along.
Different Methods to Check is String Empty Python
Let us discuss the six methods to check if a string is empty in Python and learn how we can resolve the problem with immutable empty (full of whitespaces) strings in Python.
Method #1 Using len() Function
To check if the string is empty in Python, we shall be implementing the len() method in Python. As the string with whitespace is not considered empty, it will give a numerical value for the number of whitespaces that have been used in the string. Let us check through a code example how we can check if a string empty Python for a zero-length string using the len() method
Algorithm:
- We define two test strings. One with no whitespaces and another with whitespaces.
- We use a print statement to print the question of check if the string is empty in Python or not.
- Then we implement the if-else loop to check for the condition. The condition to check if the string is empty in Python for both the test string is evaluated by using the len() method and checking if the length of the string comes out to be zero or not.
- Evaluating both the strings with the if-else loop and simultaneously printing the if the string is empty in Python or not.
- The output for test string 1 comes out to be 'yes', as the len() of the string evaluates to 0.
- The output for test string 2 comes out to be 'no', as the len() of the string does not evaluate to 0.
Code:
Output:
Explanation:
Started by initializing both the test strings where one with no whitespaces and another with whitespaces. Then we used the built-in method len() to evaluate the length of the string stored in the variables ‘string_test1’ and 'string_test2'. By using the if-else loop, we check if the condition of 'len(string_test2) == 0' evaluates as TRUE or not. When the condition sets to be true then the string is considered an empty string as evaluated for 'string_test1'. Otherwise, the string is evaluated as empty as with 'string_test2'.
Method #2 Using Not Operator
As studied above, a string with whitespaces is not considered an as empty string while the other without whitespace is considered an empty string. We can use the not operator which when used evaluates the empty string as FALSE. We can either use the not operator to utilize the variable of string instead of a condition or else we can also utilize the equal operator to match with an empty string. Only strings which no whitespaces are considered empty while we implement the not operator and hence it gets evaluated to TRUE.
Algorithm:
- We define two test strings. One with no whitespaces and another with whitespaces.
- We use a print statement to print the question of check if the string is empty in Python or not.
- Then we implement the if-else loop to check for the condition. The condition to check if the string is empty in Python for both the test string is evaluated by using the not operator and then checking if the string calculates to be zero or not.
- Then, we evaluate both the strings using the if-else loop and simultaneously printing 'Yes' or 'No' to check string empty Python or not.
- The output for test string 1 comes out to be 'yes', as the not operator of the string evaluates it as EMPTY.
- The output for test string 2 comes out to be 'no', as the not operator of the string does not evaluate it not being EMPTY.
Code:
Output:
Explanation:
Started by initializing both the test strings where one with no whitespaces and another with whitespaces. Then we used the built-in method not to evaluate the emptiness of the string stored in the variables ‘string_test1’ and 'string_test2'. By using the if-else loop, we check if the condition of 'len(string_test2) == 0' evaluates as TRUE or not. When the condition sets to be true then the string is considered an empty string as evaluated for 'string_test1'. Otherwise, the string is evaluated as empty as with 'string_test2'.
Method #3 Using Not + str.strip()
Before we jump to using the method to check if a string empty in Python, let us clarify the definition of a blank and empty string.
A blank string contains whitespaces while an empty string does not contain any characters or whitespace.
As studied above, a string with whitespace was not considered to be an empty string, and implementing the not() operator evaluates a string without whitespace as EMPTY. Here, we shall also implement the strip() fucntion in python in addition to the not() operator to remove the whitespaces present in a string. For our scenarios, a string with only whitespaces shall be considered an empty string. Now, as we shall be stripping the whitespaces from the string, it will become an empty string, and hence when we evaluate it shall return as an empty string.
With the problem statement where we have blank space in the string, we can implement this method to strip off the white spaces and check if the string is empty in Python.
Algorithm:
- We define two test strings. One with no whitespaces and another with whitespaces.
- We use a print statement to print the question of check if the string is empty in Python or not.
- Then we implement the if-else loop to check for the condition. The condition to check if string is empty in Python for both the test string is evaluated by using the not + strip() method and evaluating if the string calculates to be zero or not.
- Evaluating both the strings with an if-else loop and simultaneously printing is the if string is empty Python or not.
- The output for both test string 1 and test string 2 comes out to be 'yes', as by using the strip() method we strip the whitespace from test string 2 and it evaluates it as EMPTY. And as for test string 1, no whitespace was already present hence it was evaluated to be empty.
Code:
Output:
Explanation:
Started by initializing both the test strings where one with no whitespaces and another with whitespaces. Then we used the built-in method not operator in addition to the strip() method to evaluate if the string is empty or not. We know, a string with whitespace is evaluated as not empty with the not() operator. hence, for test string 1 as no whitespaces are present test string 1 evaluates to being an EMOTY string. Whereas for test string 2, we strip off the whitespace from the string using the strip() method and then evaluate the string against the not() operator which is considered an empty string. Hence, we get 'Yes' marked for both test strings.
Method #4 Using not + str.isspace()
Same as the above scenario, here too the string with whitespace is considered as EMPTY too. As with the strip() method, the operation requires performing that task which asks for the computation load utilization when there is more number of whitespaces. The isspace() method checks strings and gives the output as 'True' when all the characters in the string are whitespace characters else gives the output as 'False'.
As studied, a string with whitespace was not considered to be an empty string, and implementing the not() operator evaluates a string without whitespace as EMPTY. Here we shall also implement the isspace() method in addition to the not() operator to check if the string is empty in Python. Now, as isspace() method gives the output as 'True' when all the characters in the string are whitespace characters. Hence this returns it as an empty string.
Algorithm:
- We define two test strings. One with no whitespaces and another with whitespaces.
- We use a print statement to print the question of check if the string empty in Python or not.
- Then we implement the if-else loop to check for the condition. The condition to check if the string empty Python for both the test string is evaluated by using the not + isspace() method and evaluating if the string calculates to be zero or not as with isspace() method the output is 'True' when all the characters in the string are whitespace characters.
- Evaluating Both the strings are evaluated and simultaneously the output with the print statement is printed using the if-else loop.
- The output for test string 1 comes out to be 'yes', as the not and isspace() method checks a string with no whitespace as EMPTY.
- The output for test string 2 comes out to be 'no', as the isspace() method, first outputs the output as TRUE as the string contains whitespaces whereas with the not operator the string does not evaluate as being EMPTY.
Code:
Output:
Explanation:
Started by initializing both the test strings where one is with no whitespaces and another with whitespaces. Then we used the built-in method not to evaluate the emptiness of the string stored in the variables ‘string_test1’ and 'string_test2'. By using the not + isspace() method and evaluating if the string calculates to be zero or not as with the isspace() method the output is 'True' when all the characters in the string are whitespace characters. The output for test string 1 comes out to be 'yes', as the not and isspace() method checks a string with no whitespace as EMPTY whereas the output for test string 2 comes out to be 'no', as the isspace() method first outputs the output as TRUE as the string contains whitespaces whereas with not operator the string does not evaluate as being EMPTY.
Method #5 Using List Comprehension
The Python list comprehension contains brackets inside which the expression resides. This gets executed for each element in addition to the for loop to iterate over each element present in the Python list. It creates a new list depending upon the values of an existing list.
Here we shall be using the list comprehension in Python along with the if-else loop to check if the string is empty in Python.
Algorithm:
- We define two test strings. One with no whitespaces and another with whitespaces.
- We use a print statement to print the question of checking if the string empty python or not.
- Then we create a variable 'a' for string_test1 and variable 'b' for string_test2. After this, we put our if-else loop where we check if the string is empty in python. This condition is set inside the list as per list comprehension syntax in Python.
- The condition to check if the string is empty in python for both the test string is evaluated by using the list comprehension method and evaluating if the string calculates to be zero or not.
- The output for test string 1 comes out to be 'yes', as the list comprehension method evaluates a string with no whitespaces as EMPTY.
- The output for test string 2 comes out to be 'no', as the list comprehension method evaluates a string with whitespaces as not EMPTY. Both outputs are displayed within a square bracket represented in a list form.
Code:
Output:
Explanation:
Started by initializing both the test strings where one is with no whitespaces and another with whitespaces. Then we used the list comprehension in Python to evaluate the string ‘string_test1’ and 'string_test2'. We set the if-else loop where we check if the string empty python. This condition is set inside the list. The condition to check if the string empty python for both the test string is evaluated. For this scenario, the string with whitespace is considered as NOT EMPTYwhile a string with no whitespaces is considered Empty. Hence, The output for test string 1 comes out to be 'yes', as the list comprehension method evaluates a string with no whitespaces as EMPTY. and the output for test string 2 comes out to be 'no', as the list comprehension method evaluates a string with whitespaces as not EMPTY. Both outputs are displayed within a square bracket represented in a list form.
Method #6 Using the eq Method
The __eq__ method of a class in Python is automatically called whenever we implement the == operator to compare the instances of the class. Python by default uses the is operator when you don’t specifically provide the __eq__ method. Hence, The __eq__ method defines the equality logic when we want to compare two objects using the equal operator (==).
Here we shall be using the __eq__ method in python along with the if-else loop to check if the string is empty in Python.
Algorithm:
- We define two test strings. One with no whitespaces and another with whitespaces.
- We use a print statement to print the question of checking if the string empty python or not.
- Then we create a variable 'a' for string_test1 and a variable 'b' for string_test2. After this, we put our if-else loop with the __eq__ method to check if the string is empty in python.
- Once the condition is evaluated by using the __eq__ method in python it checks if the string calculates to be zero or not.
- The output for test string 1 comes out to be 'yes', as the __eq__ method evaluates a string with no whitespaces as EMPTY.
- The output for test string 2 comes out to be 'no', as the __eq__ method evaluates a string with whitespaces as not EMPTY. Both outputs are displayed within a square bracket represented in a list form.
Code:
Output:
Explanation:
Started by initializing both the test strings where one is with no whitespaces and another with whitespaces. Then we used the list comprehension in Python to evaluate the string ‘string_test1’ and 'string_test2'. We set the if-else loop where we check if the string empty Python using the __eq__ method. For this scenario, the string with whitespace is considered as NOT EMPTY while a string with no whitespaces is considered Empty. Hence, The output for test string 1 comes out to be 'yes', as the __eq__ method evaluates a string with no whitespaces as EMPTY and the output for test string 2 comes out to be 'No', as the __eq__ method evaluates a string with whitespaces as not 'EMPTY'.
FAQs
Q: When is a string considered to be EMPTY?
A: A string with no whitespaces is considered to be EMPTY while in some cases we also consider a string with whitespaces as BLANK STRING.
Q: Why is string calculated to be non-EMPTY with len() method?
A: As the string with whitespace is not considered empty, by implementing the len() method we find out the length of the string (which when non-zero tells that it is NOT EMPTY). Hence, once we get a numerical value for the number of whitespaces that have been used in the string, we can say that the string is NOT EMPTY.
Q: How do the not operator and strip methods work to prove that the string with whitespaces is empty?
A: When we work with the strip() method over a string with whitespaces, then with the strip method the number of whitespaces gets removed(stripped off) which makes the string empty and hence we obtain an empty string as a result.
Related Articles
Some articles which must be revisited to revise the concepts which are also used to implement the various methods we learned so far are listed below:
- if-else loop Python.
- not Python.
- and Python.
- print Python.
- list comprehension Python.
- eq method in Python.
- isspace() method in Python.
Conclusion
-
A blank string contains whitespaces while an empty string does not contain any characters or whitespace.
-
The method where White spaces in strings are considered Empty is:
- not + strip() method. * not + str.isspace() method.
-
The method where White spaces in strings are considered as not Empty or (Blank) is:
- Using list comprehension
- len() method
- not operator
- __eq__ method
-
We learned that for some use cases, string whit whitespaces are considered empty while for some it is not considered empty. hence, depending on the use case we can choose to form the different methods we discussed above.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.