Python Sum() Function
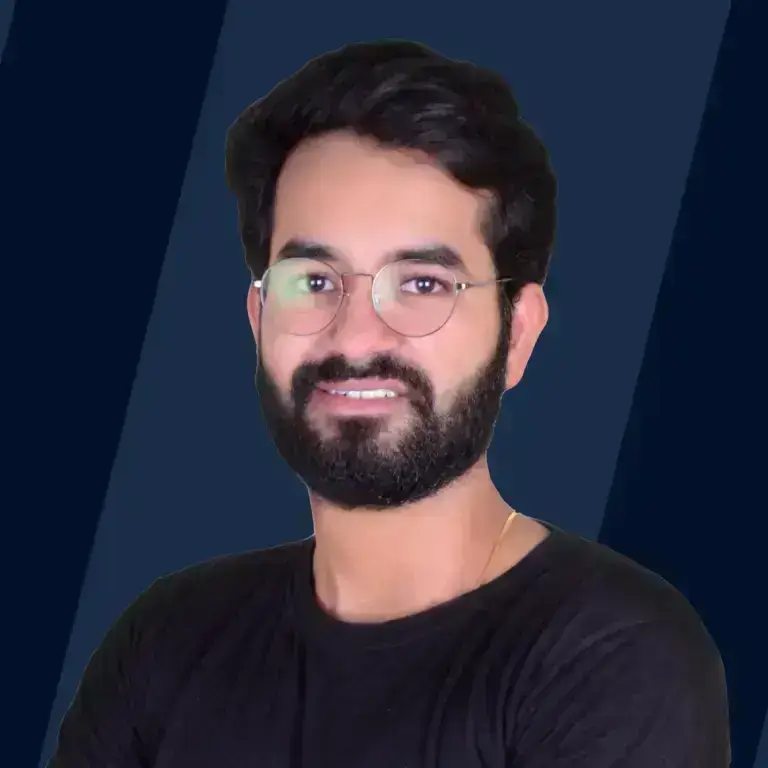
The sum() in Python is a built-in function that calculates the sum of elements of an iterable (such as lists, tuples, etc). An optional second offset argument can also be provided as a "starting point" for your sum, by default, it is equal to 0.
Example of Python sum()
Let consider a list of numerical elements (that could be integer, floating-point numbers, and complex numbers) to find the sum.
Output:
Explanation:
Since the value of start parameter is 0, therefore the value calculated by sum() function in variable result1 is 1+2+3...9+10 = 55. On the other hand, since we passed a second parameter with start = 4, we got result2 = result1 + start
Syntax of sum() function in Python
The syntax of sum() is as follows:
Parameters of Python sum()
There are two passable parameters while calling sum() in Python:
-
iterable: This is an iterable object like a list, tuple, dictionary, set, etc. This is a required as well as a positional parameter, that is, it can't be left out while calling the function and must be specified as the first argument, respectively.
-
start: This is the second parameter which is optional. This must be of some appropriate type depending on the type of contents of the iterable. Examples below will make this statement more clear. This argument can be passed using both position and name.
Return Value of sum() function in Python
The Return Type can be,
- integer
- float
- complex number
It depends on the type of the contents of the iterable passed in the function. The sum() in Python returns an appropriate value which is equal to
Here sum can refer to numerical sum or concatenation operation for lists and tuples.
More Examples
Implemantation of sum() in Python
We can also implement our own sum() function in Python, as illustrated below. Remember, this is not the exact implementation but successfully imitates the functionality of sum() in Python in most cases. For the exact implementation, you can check out Python's source code.
Code:
Explanation:
We pass param, an iterable (which can be a list, tuple, etc.) to the function mySum() where a for loop iterates over its contents and keeps adding them to the variable sum. In the end, the function returns sum+start, where the value of start is 0 by default.
Example 1: Find the sum of a tuple in Python
The steps for finding the sum of elements of a tuple are the same as that of a list. Just call the function by passing the tuple as a parameter.
Code:
Output:
Explanation:
Here we called the sum() function by passing tuples of integers, floating-point numbers, and complex numbers. As you can see, the data types of the corresponding results are also integer, a floating-point, and a complex numbers only.
Example 2: Find the sum of set elements in Python
Just like list and tuple, we can also use sum() in Python to obtain the sum of elements of a set as illustrated below:
Code:
Output:
Explanation:
The sum() functions returns the expected results. Two things are worth noticing in the above example:
- While calculating result2, we gave a set of floating-point numbers starting with type complex numbers. Hence in the total sum, we get a complex number with the contents of the set being added to its real part and start as an imaginary part.
- While calculating result3, we gave a set of complex numbers with a start value of type floating numbers. As a result, the summation of start ended up being included in the real part.
Example 3: Find the sum of dictionary keys in Python
Surprisingly, the sum() function in Python also works with Python dictionaries. If you pass a dictionary with numeric keys, the function will return the summation of the numeric keys present in that dictionary. The following code examples will help you understand it better.
Code:
Output:
Explanation:
- In the first function call, we provided the dictionary as input, and it calculated the sum of key values, 1+2+3+4+5+6 = 21.
- The next function calls sum() to calculate the sum of key values of the dictionary along with an offset value of 4.55. Here you can see some strange behaviour concerning the result's precision (we will discuss it later).
- In the last function call, we had complex numbers as the key, and consequently, the sum obtained of the key using sum() in Python is also a complex number.
Example 4: Finding the sum of the list which contains floating point number
Code:
Output:
Explanation:
As you can see, the precision of the first output doesn't match the precision of the inputs. But in the second output, it does. This "strange" behaviour is because of how the floating-point operations are handled internally in Python.
Example 5: Passing a List of string and Numbers
Code:
Output:
Explanation:
Here we got an error because while summing up the contents of mylist1, the program encountered a string, which could not be added to the integer using the + operator.
Example 6: String offset along with List of numbers as Iterable
Let's see what will happen if there is a mismatch in the datatypes for the contents of iterable and the start parameter.
Code:
Output:
Explanation:
The error came because when we pass a string as the second parameter to sum(), the program guessed that we're trying to concatenate or sum the strings, and throws an error showing the function sum() in Python cannot sum strings.
Example 7: Passing different data type as offset
Let us see another error case where the data types of iterable and start parameters don't match.
Code:
Output:
Explanation:
Here the contents of the iterable are of type list while the start parameter is of type int, and hence because of this type incompatibility, the program showed an error. You'll get similar errors in other incompatibility cases like when the iterable is a list of tuples, and the start value is either an integer or a list.
Example 8: Using sum function with List of set
Let's see what will happen if the contents of the iterable are not summable under the addition + operator.
Code:
Output:
Explanation:
We can see here that the program gave an error specifying that two set objects are not proper operands for the addition (+) operator. Understand, this is different from the case when we were adding the contents of the set. Here we are trying to somehow add the sets themselves. The same kind of error would be shown if we tried to "add" dictionaries.
But would we get the same error if tried something similar with lists and tuples? The answer is no! Why? It is because lists and tuples possess the concatenation property (similar to strings). A point worth noticing is, the + operator in Python is capable of not only adding numbers but also of concatenating. This is the reason why the sum function in Python would not give an error if we try to "add" them up. See the subsequent example.
Example 9: Using sum function with List of List and Tuple
Code:
Output:
Explanation:
- In the first case, the sublists present inside the exp1 are concatenated to the empty list [] (given as the start parameter). The final list is shown as the output.
- In the second part of the code, the tuples present inside the list are concatenated with the tuple (1,3) given as the start parameter.
- One important thing to observe is that in both these cases, the order of the contents of the lists and tuples are preserved in the final output.
Sum of floating-point numbers in Python
As we saw in earlier examples, using sum over floating-point numbers yields strange results in Python. Therefore, it is recommended to use fsum() function provided in the math module of Python's Standard Library to add floating-point numbers in a list, tuple, etc.
Let's see an example to show the difference in the results we get:
Code:
Output:
Explanation:
- We can see that fsum() returned the sum with the correct precision, while sum() gave a strange result, because of Python's internal calculation with floating point numbers.
- Here we called the fsum() function from the imported math module, which is the recommended over sum for adding floating-point numbers. It is important to remember that unlike sum, the fsum doesn't take an optional second argument, that is, it takes only one argument, that is, the iterable.
Conclusion
- Python sum() can sum over the elements of iterables such as lists, tuples, dictionaries, sets, but not strings.
- We can also provide a second parameter called start while calling the sum() function which acts as an offset/starting value for the sum of the iterables.
- This second parameter can be passed as a positional argument as well as a keyword argument. Also, this second argument can never be a string.
- Python sum() can also be used to concatenate lists and tuples.