What is a Move Constructor in C++?
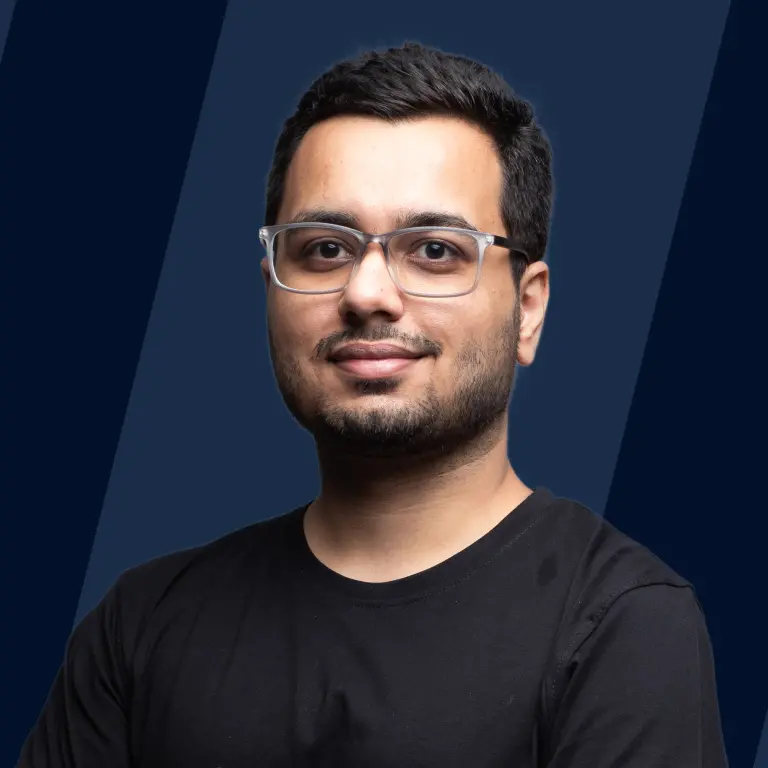
Prerequisites
Rvalue References and Move Constructor
The r-value references and move semantics are used by the C++ move constructors. Move semantics helps in pointing to an existing item in memory using the r-value references.
The l-value references and copy semantics are utilized by the C++ copy constructors. Copy semantics refers to transferring the object's real data to another object by making a copy in the memory, instead of making another object point to an already existing item in the heap.
Why are Move Constructors Used in C++?
As we know, copy constructors are used to copying the data from an old object and assign it to the new object, while the move constructor is used to make a new pointer to the old object and transfers the resources to the heap memory. Simply put, the move constructor nulls away the temporary object's pointer and makes the declared object's pointer point to the data of the temporary object. As a result, the move constructor stops unnecessary data copying in the memory.
Let's see the programs with and without declaring the move constructor in C++.
Program Without Declaring the Move Constructor
Let's first look at a C++ program in which we only use the copy constructor and do not use the move constructor.
Output:
Explanation: First, the default constructor function is invoked when the temporary object of Move is formed as a result of the code that was previously mentioned. The temporary object of Move is placed back into the vector vec, calling the copy constructor function.
There is a significant performance overhead in the code above because the temporary object of the Move class is first generated in the default constructor and then in the copy constructor. To eliminate this speed cost, we use the move constructor.
Program Declaring Move Constructor
Let's look at a C++ program in which we define the move constructor along with the copy constructor.
Output:
Explanation: The move constructor is called during the execution of the above-mentioned code rather than the copy constructor. The copy of the temporary object of Move's class is averted using the move constructor. So, This often costs significantly less than creating a duplicate, using the move constructor in a C++ program rather than a copy constructor is an effective option.
More Examples of Move Constructors
Let's see some examples of move semantics to understand more about the move constructors in C++ programs.
Move Semantics - Illustration 1
We are transferring ownership (pointer) of one object to another using the move constructor in the below C++ program.
Output:
Explanation: When we used the move() function call, we transferred the ownership of data of obj1 to obj2. We can see from the output that obj1 has an empty string, while obj2 has a default string after we have called the move() method of the Move class.
Move Semantics - Illustration 2
We are using inheritance and move semantics in the below C++ program.
Output:
Explanation: In the above C++ program, we are making an object and using move semantics by inheriting a class A from a move constructor class Move in the below C++ program.
Move Semantics - Illustration 3
The below example shows how the move constructor is not invoked if we have a destructor defined in the inherited class.
Output:
Explanation: In the above C++ program, we have our destructor defined for the inherited class B, so the move() won't call the move constructor, instead it uses copy constructor as shown in the output above. If we comment out the destructor of class B in the above program, then object b2 will call the move constructor only.
Move Semantics - Illustration 4
The below example shows the forced invoke of the move constructor if we have a default assigned in the move constructor of the inherited class.
Output:
Explanation: In the above C++ program, we have defined another inherited class C from the Move class which has a move constructor. The move constructor is assigned with the default which makes a forced invoke of the move constructor even if the destructor is defined in the inherited class.
How do Move Constructors Work?
Two new functions, a move constructor and a move assignment operator are defined in C++11 to provide move semantics/functionalities. While the move constructor and move assignment aim to transfer ownership of the resources from one object to another, the copy constructor and copy assignment aim to create a copy of one object to another. Move semantics often costs significantly less than creating a duplicate using the copy constructor.
The move semantics requires non-const r-value reference arguments, on the other hand, the copy semantics of these functions require a const lvalue reference parameter.
A move constructor and move assignment operator won't typically be given by default, unless the class has no defined copy constructors, copy assignments, move assignments, or destructors.
FAQs
Q. Why move constructor is better to use as compared to copy constructor?
A. Copy constructor makes a copy of an object in another object which takes up the space in the memory while the move constructor helps in transferring the ownership of one object to another and stores data in heap memory. Move semantics doesn't take any extra space in the memory.
Q. What distinguishes a move constructor from a move assignment?
A. A move constructor is invoked only when we construct a new object, while a move assignment operator is used with previously constructed objects.
Related articles
Conclusion
- Lvalue, Rvalue, and, copy constructor in C++ are some of the prerequisites to understanding the move constructor in depth.
- The l-value references and copy semantics are utilized by the C++ copy constructors to copy one object's data into another object of the same class.
- The r-value references and move semantics are used by the C++ move constructors. Move semantics helps in pointing to an existing item in memory using the r-value references.
- The move semantics aims to transfer ownership of the resources from one object to another, while the copy semantics aims to create a copy of one object from another.