Functions with Variable Number of Arguments in C++
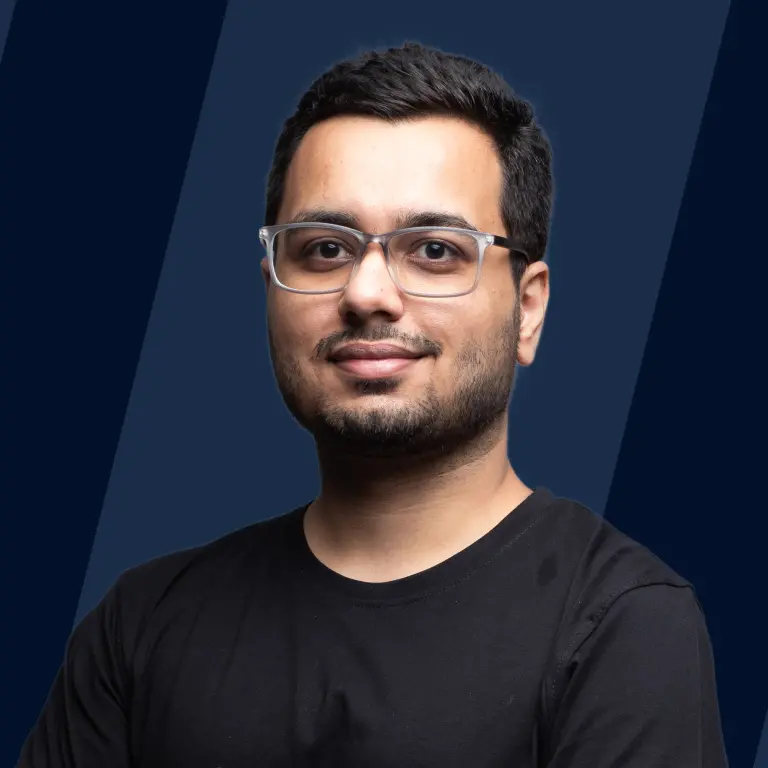
Overview
The variable number of arguments in C++ is a feature that permits a function to accept any number of arguments. This type of function is called variadic functions or functions with a variable number of arguments in C++.
Introduction to Variable Arguments
In C++ programming, you can find yourself in a scenario where you need a function that can take a variable number of arguments, i.e., parameters, rather than a fixed number. The C/C++ programming language has a solution for this problem; you can construct a function that accepts various parameters depending on your needs.
Based on user requirements, a variable number of arguments can be of two types:
- When the data type of all the arguments is the same
- When the data type of all the arguments is different.
Implementation Technique of Variable Arguments before C++11 Update
When the Data Type of all the Arguments Is the Same
In this case, a vector or an array of the required data type can be passed in a function.
Syntax:
When the Data Type of all the Arguments is Different.
In this case, variadic functions are declared under the cstdarg header file.
Implementation Technique of Variable Arguments after C++11 Update
When the Data Type of all the Arguments is the Same
After the C++11 update, we have the concept of an initializer list that can take the same data type variable arguments. For this, the header file <intializer_list> needs to be included.
Syntax:
When the Data Type of all the Arguments is Different.
Variadic templates can also be used to generate functions with variable arguments. Variadic templates have the advantage of not imposing limits on the types of arguments, not performing integral and floating-point promotions, and being type-safe.
Steps To Implement the Functionality of Variable Arguments
In this section, we will see some examples of functions with a variable number of arguments in C++.
Implementation of Same Data Type Variable Arguments
Implementation Using "vector":
- Include <vector> header file.
- Define a function having parameter as a vector of required data type.
- Call the above-defined function from the main() function with parameter as the variable arguments inside the curly braces.
Code:
Output:
Implementation Using "initializer_list":
- Include <initializer_list> header file.
- Define a function having a parameter as an initializer_list of the required data type.
- Call the above-defined function from the main() function with parameter as the variable arguments inside the curly braces.
Code:
Output:
Implementation of Different Data Type Variable Arguments
Implementation Using "cstdarg"
The header file cstdarg contains variadic functions and macros for implementing a function's variable number of arguments.
Variadic functions take a variable number of arguments (for example, std::printf). An ellipsis appears after the list of parameters, e.g., int printf(const char* format...);, which may be preceded by an optional comma, to declare a variadic function.
The following library facilities are given to access the variadic arguments from the function body:
Macro | Usage |
---|---|
va_start | Allows variable function arguments to be accessed |
va_arg | Accesses the next variadic function argument |
va_copy | Makes a copy of the variadic function arguments |
va_end | Ends traversal of the variadic function arguments |
va_list (not a macro, but typedef) | Stores the information that va_start, va_arg, va_end, and va_copy require |
Code
Output:
Implementation Using Variadic Templates
- Declare a template with typename T.
- Define a function with a parameter t of datatype T.
- Declare another template with typename T and typename... Args.
- Define another function with the same name having two parameters. First, t with datatype T, and the second, args with type Args....
- Call the above function from the main() function with variable number of arguments.
Code:
Output:
Examples of Functions with Variable Number of Arguments In C++
Finding Maximum of Integer and Floating Point Numbers
Code:
Output:
Conclusion
- The variable number of arguments in C++ is a feature that permits a function to accept any number of arguments. This type of function is called variadic functions.
- Functions with a variable number of arguments in C++ can be easily implemented using a vector or an array if all the arguments are the same type.
- If all arguments are of different types, then we need to use the cstdarg header file to implement variadic functions.
- Variadic functions do not put restrictions on the types of arguments. They also do not perform integer and floating-point enhancements.
- The header files to be imported are cstdarg.