Union and Enum in C++
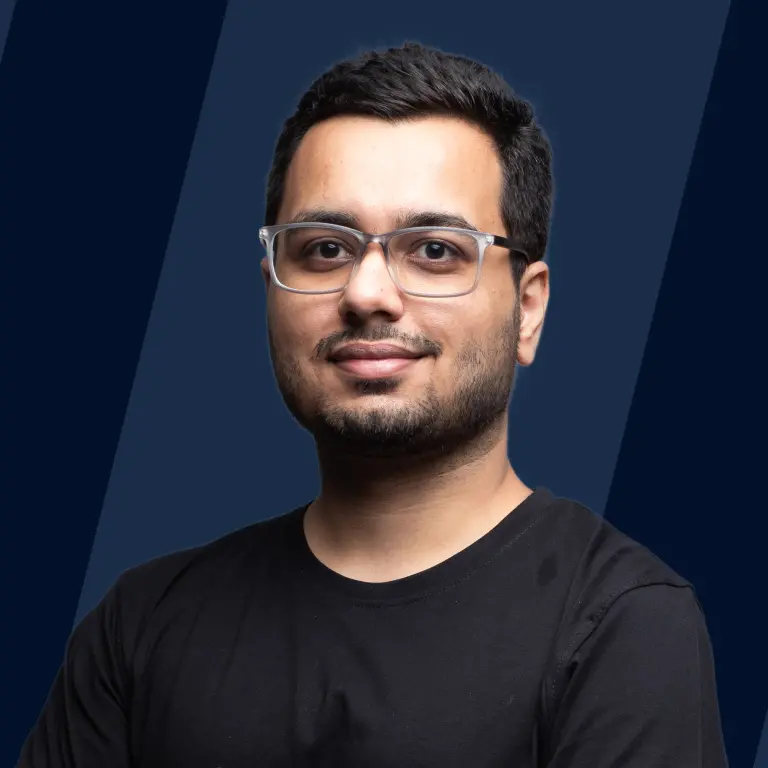
Overview
Union and Enumeration are both user-defined data types. Union is defined using the union keyword, and it is used to bind two or more data types and share the same memory. The memory of union variables is equal to the largest member of the union. The enumeration type contains the constant integral values known as enumerators. Enumerators have default values as array indexes.
Introduction
There are two types of data types in C++ language first Primitive data types, which are built-in or pre-defined in the C++ language like int, char, double, etc., while the other is User-defined data types, which are created by the user using the primitive data types like Structure, Union, array, etc. Union is a user-defined data type that combines one or more primitive or user-defined data types sharing the same memory block. At a given instant we could only use one member of the union variable. Union variable size is equal to the size of the largest member of the union in terms of memory. Also, suppose we use one member of a union variable and assign value to another member. In that case, it will overwrite the memory of that variable which means we will lose the data of the previous member we were using.
Syntax
To create a Union in C++, the union keyword is used followed by the tag name of the union. Then the body of the union is defined, in which the required data members (primitive or user-defined data types) are added.
Here union is the keyword, and union_name is the name of the union data type defined by the user. Data_members could be any C data type, and union_varialbes are the variables defined for the union.
Declaration of Union Variable
To use the properties of the created union in C++, we have to create union variables, and there are two ways to declare variables for union in C++ language:
- Variable declaration with the union definition itself.
- Variable declaration is the same as a primitive data type.
Variable declaration with union definition:
Syntax
Variable declaration as a primitive or basic data type:
As we create a union in C++, this means we have created a user-defined data type. So this data type can be treated as the primitive data type while declaring a variable for that union.
Syntax
C++ program illustrating the implementation of union
We have seen the syntax of the union in C++ and how to declare a union variable. Now let’s see an example of a union consisting of various members of different data types.
Output:
In the above code, we have created a union with character, integer, and double data type variables as the union members. After that created a union variable var1. Then one by one, we assign char, int, and double values to union members ch, it, db, respectively, and simultaneously print them also.
The special thing about the union in C++ is that all these different members of the union use the same memory location, so we could only use one member of the union at a given time.
Proof that all the members of the union share the same memory address:
Let’s create a union in C++ where three members are present with int, float, and double data types. We will try to access their location to get proof that they share the same memory location:
Output:
We can see all of the union members have the same memory address.
Anonymous Unions
Anonymous unions are the unions that are declared without a name. The rule to define anonymous unions is we can declare a union in C++ without a name, but the data members of the union must have a unique name in the scope where the union is defined. Also, as there is no name for the union, we cannot define the variables for the anonymous unions, but we can directly access the data members of anonymous unions. Let’s look into the syntax of the anonymous unions:
Syntax:
We can see from the above syntax there is no name declared for the union. To get more idea, let’s see its example:
Output:
In the above code, we defined an anonymous structure with two data members—assigned value to one member and then printed for all of them.
Union-like Classes
A class consisting of at least one anonymous union is known as a union-like class. The members defined in the anonymous union in C++ are known as variant members in this case. Let's see an example of this for more understanding:
Output:
In the above example, we have created a union-like class. Then, we declared an integer and anonymous union as a class data member. In the main function, we created an object of the class. We accessed integer variables and anonymous union members by our object, assigned values to them, and finally printed them. The advantage of a union-like class is that we could use int or character(in the above example) whenever any of it requires by using common space for both of them.
Difference Between Union and Structure
- While creating a union in C++, union keyword is used and for structure struct keyword is used.
- Memory is the main difference here. A structure variable has memory equal to the sum of the memory of all the data members of the structure. On the other hand, a union variable has memory only equal to the greatest data member’s memory size because, in structure, every member will get separate memory space. Still, in the case of a union, every member will share the same memory space.
- One data member of the union in C++ can be initialized while creating a union, while non of the data members of the structure be initialized.
Read More: Difference Between Union and Structure
What Is C++ Enum?
Enum is a keyword used to create a user-defined data type, also known as Enumerated type, which can take only a limited set of constant integral values. The user defines these values, and after the declaration, if a user tries to assign an enumerated variable a value that is not in the pre-defined set, then the compiler will throw an error. All the values defined in the enumerated type are named by the user and known as enumerators.
Syntax
In the above syntax, we have used the enum keyword followed by the name of our enumerated data type, then defined some values it can achieve in curly braces separated by coma.
By default value1 = 0, value2 = 1, … , also as value7 = 90 so value8 = 91 and so on.
Enumerated Type Declaration
When we create an enum in C++, we need its variables to use its properties and there are two ways to declare variables for the enumerated type.
- Variable declaration with the enumerated type definition itself.
- Variable declaration as a primitive data type.
Variable declaration with the enumerated type definition itself:
Syntax
Using the enum keyword creates an enumerated type in C++, and after defining its body, we could also declare the variables like the above-used syntax.
Variable declaration as a primitive or basic data type:
As we create an enum in C++, we have created a user-defined data type. So this data type can be treated as the primitive data type while declaring a variable for that type.
Syntax
How to Change the Default Value of Enum
Default values of the Enum values are one less than their position in the declaration list. To change that value, we can assign them a new value as we assign to int variables while declaring them, and then the following member will follow the same by having a value one more than their previous member.
Let's see an example for more clearance:
In the above example, we have used the enum keyword followed by the name of our enumerated data type and then defined some values. By default value1 = 0, value2 = 1, we changed the default value of value3 to 98, making the default value of value4 = 99.
Why are Enums Used in C++ Programming?
- Enum in C++ is used to make code neat and more readable.
- To reduce the mistakes in the code.
- We can traverse through the enumerated types using loops.
- Enums in C++ are helpful while writing switch-case statements.
Unscoped Enumeration
When we create an unscoped enum in C++, the enumerators have the same scope in which the enumeration type is defined. If we try to give the same name as the enumerator to another variable in that scope, it will give us an error. Unscoped enumeration is the basic enumeration, as we have seen above.
Output:
In the above code, we tried to redefine the green enumerator, which led to an error, as shown in the output. Because our enumerator is unscoped, and its enumerators share the scope of main function.
Scoped Enumerations
Scoped enum in C++ is created using the class keyword. The scope is only the enum list itself, which means we can give the name to any other variable in that scope similar to the enumerators in the enum list or vice-versa. Let’s see how to declare scope enumerations:
Output:
In the above code, we have defined our enum in C++ by using the class keyword before the name of the enum, which makes the scope of our enum-type limited more-precisely scope is limited only in the enum list, which means we can define a variable with the same name in that scope where enum in C++ is defined.
If we want to access and use the scoped enumerator members, we have to explicitly convert them into integers by using the static_cast<int>{} method and the scope resolution operator. Let’s see this by an example:
Output:
In the above example, we have created a scoped enumeration type with a variable var and initialized it with a value present in the enum. At last, printed that value by explicitly converting it into an int.
Points to Remember for C++ Enum
- Enumerated type data types are defined using the enum keyword.
- Enums consist of integral constants values; by default, they have value as array indexes.
- Enums are of two types scoped and unscoped.
- Scoped enumerated data types are defined using the class keyword.
Examples
Let’s see an example of enums:
Output:
Here, we first created an enum in C++, then created its two variables using both syntaxes, as discussed above. Then we traversed through the enum type using the for a loop.
Conclusion
- Union and enum in C++ are user-defined data types.
- Union is defined using the union keyword, and it is used to bind two or more data types and share the same memory.
- The memory of union variables is equal to the largest member of the union.
- There is a kind of union known as an anonymous union and declared without any name.
- Enum in C++ is defined using the enum keyword and contains the constant integral values known as enumerators.
- There are two types of enum in C++ unscoped enums and scoped enums. Scoped enums are defined using the class keyword.