encode() in Python
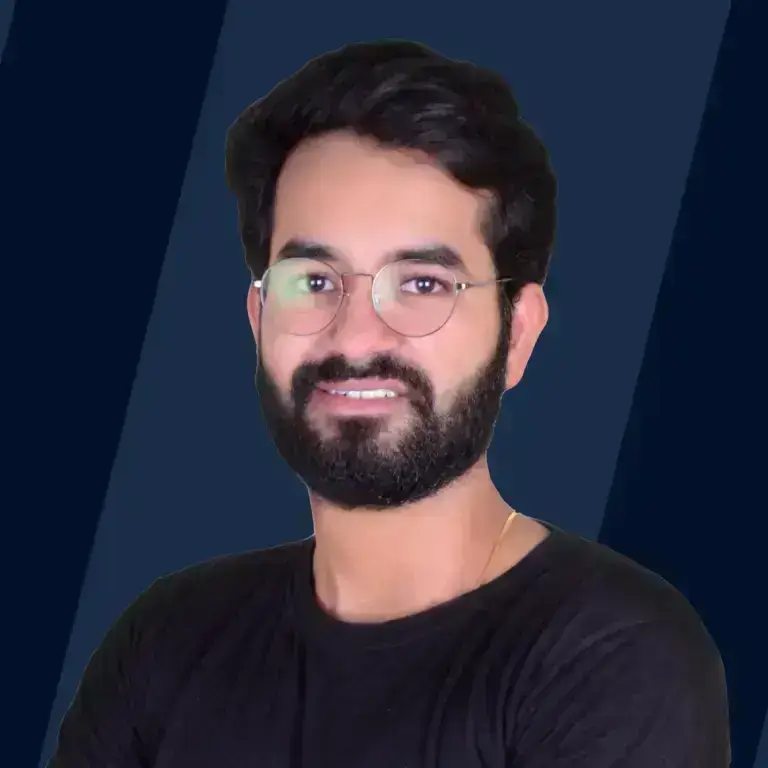
Overview
The encode() function in Python is responsible for returning the encoded form of any given string. The code points are translated into a series of bytes to efficiently store such strings. This process is defined as encoding. Python uses utf-8 as its encoding by default.
Syntax for encode() function in Python
Syntax:
Both the parameters used in the encode() function are optional, and the encoding standard used for Python programming is UTF-8. The second parameter, the error, also has a default value of strict and possible alternative values of 'replace' and 'ignore'.
Parameters of encode() in Python
-
encoding: As we know, the default encoding standard in Python is known to be UTF-8 which means that if no encoding is mentioned, then UTF-8 in python will be used.
-
errors: The parameter errors mode is responsible for ignoring or replacing the error messages. Determines how problems are handled or taken care of if any arise; There are six different error responses. Let's discuss a few of them.
- error name -Strict: If we put the error parameter as strict. It is Python's default answer and is responsible for issuing a UnicodeDecodeError exception in case of a breakdown.
- error name –Ignores: If we assign the error parameter to ignores, It ignores the unencodable Unicode.
- error name - Replaces: In this case, the unencodable Unicode is replaced by a ?
Return Values of encode() function in Python
The encode() function in python returns a string that has been encoded.
Example of encode() in Python
Let's use a simple program to understand how to encode in Python.
Code:
Output:
Explanation:
The output of the above code is b'ciao' and ciao. The encode() function in Python converts the given string 'ciao' into its encoded form, and the decode() function converts it back to its original form.
What is encode() function in Python?
These days security is essential in a diverse range of applications. As a result, there seems to be a requirement for safe password storage in the databases and the ability to preserve encoded varieties of any given strings.
To encode strings, a function in Python named "encode()" encodes texts using the chosen encryption algorithm. The programming language specifies numerous encoding techniques. This Python String encode() function is responsible for encoding the string utilizing the provided encoding. When no encoding is provided, the type of encoding that will be used is UTF-8.
How to use encode() in Python?
- Strings in Python are always in the format -utf-8, which implies that each letter refers to a distinct code point.
- We utilize the encode() function on the string taken as input, available on all string objects.
- The encode() function encodes any given string given as input using encoding, along with errors determining the behavior to be performed if the encoding fails on the given string.
- The result of encode() is a series of bytes.
More Examples
Let's go through a few examples to understand the concept of encoding in a conceptual manner.
Example 1: Encoding String with default values
Code:
Output:
Example 2- Encoding String with Error Parameter
Code:
Output:
Example 3- Encoding a Latin Character using Encode in Python
Code:
Output:
Example 4- Encoding a String by passing the second parameter error with ignore
If we wish to disregard failures, we may substitute the second parameter with ignore.
Code:
Output:
Example 5- Encoding a String by passing the second parameter error with replace
If we wish to disregard failures, we may substitute the second parameter with replace. It disregards the mistake and it replaces the character with a question mark.
Code:
Output:
Example 6- Encoding a String by passing the second parameter, error with backslashreplace
If we wish to disregard failures, we may substitute the second parameter with replace. It disregards the mistake and it replaces the character & instead of an unencodable unicode, \uNNNN escape sequence is inserted.
Code:
Output:
Example 7- Encoding a String by passing the second parameter, error with xmlcharrefreplace
If we wish to disregard failures, we may substitute the second parameter with replace. It disregards the mistake and it replaces the character & replaces unencodable unicode with an XML character reference.
Code:
Output:
Conclusion
- Encoding and decoding may be utilized simultaneously in basic applications such as conserving passwords on the back end and various complex applications such as cryptography, which deals with preserving information secrets.
- In Unicode, every character in the given string in Python is denoted by a certain code point. Therefore, every string in Python can be said as a series of Unicode code values.