filter() Function in Python
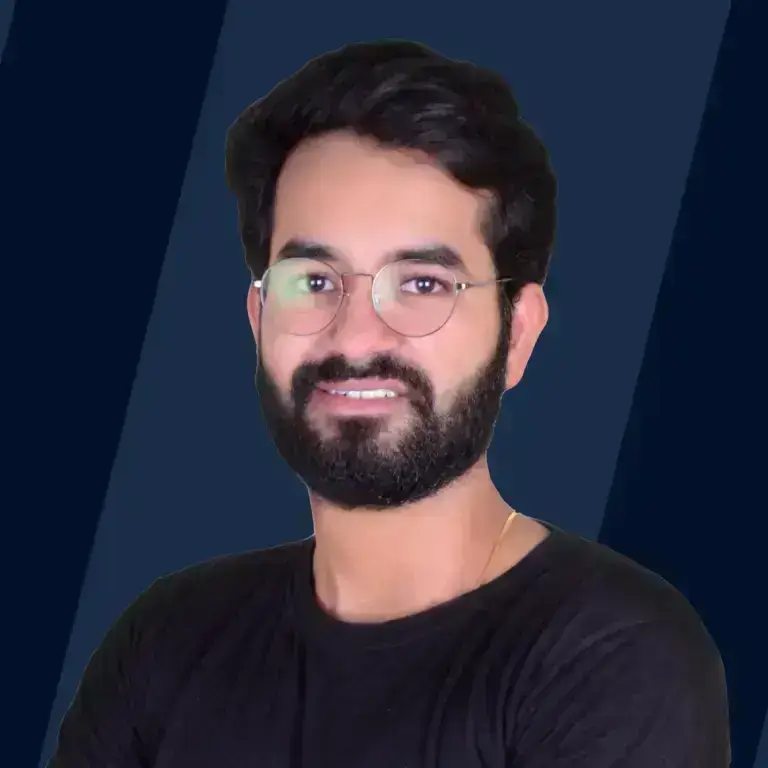
Overview
The built-in filter() function in python extracts only particular elements from an iterable (like list, tuple, dictionary, etc.) It takes a function and an iterable as arguments and applies the function passed as an argument to each element of the iterable; once this process of filtering is completed, it returns an iterator as a result. An iterable is a Python object that can be iterated over.
Syntax of filter() in Python
The syntax of filter function is as follows,
Parameters of filter() in Python
The filter() function takes two parameters:
- function: used to check if each element of the iterable holds true for this function or not.
- iterable: iterables like sets, lists, tuples etc. on which filtering will be done.
Return Value of filter() in Python
Return Type: <class 'filter'>
The filter() function returns an iterator that contains all the elements of the input iterable that have passed the function check.
Example of filter() in Python
We have a list of numbers and want to filter out those numbers that are divisible by 5 by applying a filter on that list. We can create a simple function to check whether a number is divisible by 5 or not, which returns True or False. Subsequently, based on this boolean value, the filter function decides whether to keep that particular element iterable(which is going to be returned) or not.
Output:
Explanation:
In the above example, the filter function is passed on a list of numbers along with our pre-defined divisible as a function argument. Only those elements are returned by the filter function for which the function divisible returns true. Therefore, all the numbers that are not divisible by 5, such as 3, 192, 77, 91 are not filtered out.
What is Filter Function in Python?
The filter() function is used for filtering values from an iterable.
Also, it is more efficient than list comprehension because a list comprehension will create a new list, which will increase the run time for that process and after the list comprehension has completed its processing, we’ll have two lists in memory.
While in the case of a filter() function, it will make an object that holds a reference to the original list, which will take up less memory, thus decreasing the run time of the process.
There are plenty of ways to filter out elements from an iterable.
For example, using a simple for loop, list comprehension, nested for loops, etc. But the filter method is the most widely used among all these methods, as it provides an efficient and optimized way of filtering elements that, too, in fewer lines of code to perform the same task.
Applications of Filter Function in Python
The filter() method in Python is used for selecting particular data from a large set of data.
Also, it is a replacement for list comprehension, as a filter requires relatively low memory and execution time.
The filter function can be used with lambda functions (single-line functions), pre-defined functions to filter elements based on the check performed by the function.
For example, if we want to filter out how many people from a given list of their ages are eligible to vote in elections, we can use the filter function. We can create a function that will return True if a person is eligible to vote or not (i.e., age >=18) and then pass that created function as an argument to the filter function along with the given list of ages of different persons as an iterable.
Traditional For Loop vs Filter Function
Output:
Explanation:
In the traditional for loop approach, we have defined a function eligible which consists of a for loop to iterate over the list and then an if block to decide which element to keep in the final list.
While using a filter method, the function eligible checks whether the particular age is greater than or equal to 18 or not. The function is then passed as an argument to the filter function along with the list ages as an iterable, and as result, a filtered object of valid ages is returned.
More Examples
Example 1: Using a Lambda Function
We will use the lambda function to filter out vowels from the given list of alphabets instead of a traditional function in the parameter.
Output:
Explanation:
A list named letters is passed as an iterable. We have defined a lambda function vowels that takes a list of letters as an argument, and after converting every element of the list into lowercase, function returns True if that item is a vowel, else False. Then, the filter function applies that lambda function to every element in the list of letters.
Example 2: Using None as a Function Inside filter()
We will use None as a function argument in the filter function that will remove any element from the iterable that the filter considers to be false or Null. Some instances of similar elements are empty strings, 0, empty braces, boolean False, etc.
Output:
Explanation:
The list is passed on filter function along with None as a function argument. Hence, only those elements are returned that correspond to being true. Therefore, all the false elements, such as empty braces, 0's, empty strings, etc. are not filtered out.
Example 3: Using filter() with a List of Dictionaries
We will create a list of dictionaries that will store details of food items that as the item name and its price. The aim is to filter out those items that we can purchase within our budget price.
Output:
Explanation:
A list of dictionaries that contains details of food items is passed as an iterable. We have also defined a function that takes a dictionary as an argument and returns True if the price of the item is less than 150, else False.
Then, a filter is used on the list of dictionaries along with the pre-defined budget function. The returned filter object budget_items is iterated to print the name of filtered items.
Conclusion
-
To conclude, we learned the ways of using the filter() function in Python. We can use it with a pre-defined function, a lambda function, or with None by concurrently providing an iterable.
-
The Filter() filters out data based on whether the function provided as an argument returns True or False for that particular data element.
-
We can filter for items in every iterable object of python i.e. lists, dictionaries, and other types of data structures.