Function Overloading in Python
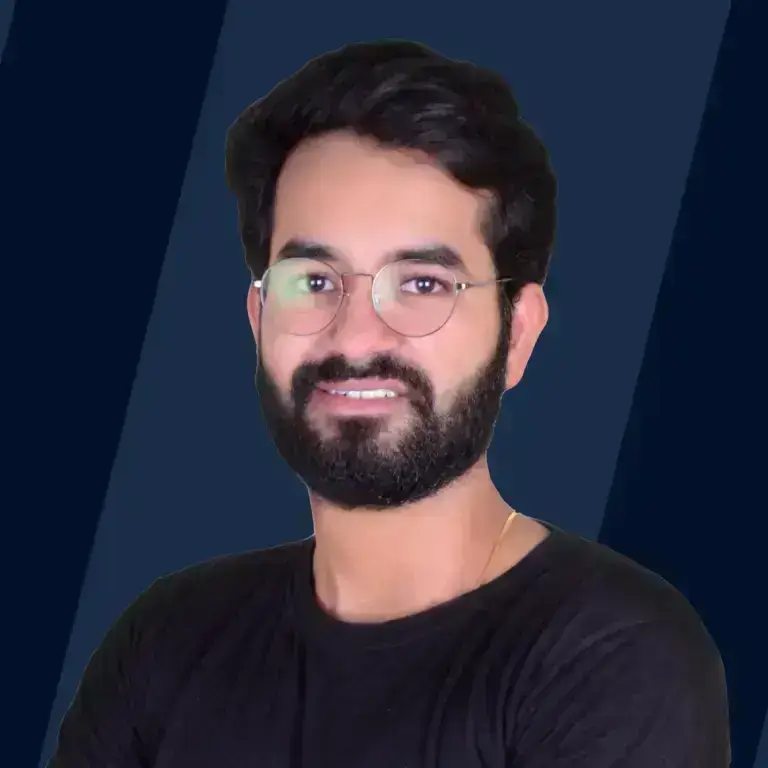
Overview
Function overloading is the feature when multiple functions have the same name, but the number of parameters in the functions varies. Python does not support function overloading as in other languages, and the functional parameters do not have a data type. Suppose we want to use the feature of functional overloading. In that case, we can set the default values of parameters in the method as None, which won’t give an error if that specific value is not passed as an argument while calling the function.
What is function overloading in Python?
As the name suggests, function overloading is the process where the same function can be used multiple times by passing a different number of parameters as arguments. But Python does not support function overloading. An error gets thrown if we implement the function overloading code the way we do in other languages. The reason is as Python does not have a data type for method parameters.
The previous function having the same name(but different parameters) gets overridden with the new function having the same name. So now, if we try to call the first function, which had different number parameters, by passing a different number of arguments defined in the new function, we get an error. Let us try to understand the same.
Code:
Output:
In the above program, we can see that the second sum method overrides the first sum method. When we call the function using three arguments, it gives the output, but when we use two argument, it gives an error. Hence we can say that Python does not support function overloading.
But does that mean there is no other way to implement this feature? The answer is no. There are other ways by which we can implement function overloading in Python.
We can achieve it by setting one or more parameters as None in the function declaration. We will also include the checking condition for None in the function body so that while calling if we don't provide the argument for a parameter that we have set as None, an error won't occur.
Syntax
We will define functions by setting one or more parameters as None and by including the checking condition for None in the function body so that while calling, even if we don't provide the argument for a parameter that we have set as None, an error won't occur.
We set the parameters as None, as it gives us the option of calling the function with or without the parameter.
Let us understand this with an example-
Code:
Output:
Here we can see that after setting the default values of the parameters as None and by adding a few validations, we can implement function overloading.
How function overloading works in Python?
In Python, when we define two functions with the same name, the latest one is considered a valid function. If we want to implement the concept of function overloading, we need to set the default values of the parameters of the function as None. By setting the value of functional parameters as None, we get the option of calling the function either with or without the parameter. So, if we don't include the parameter set as None, it will not give an error.
Overloading Built-in Functions
In the Python Data Model, we have a few special functions and it provides us the means of overloading the built-in functions. The names of the special functions begin with double underscores(__).
In our example, we will change the default behavior of the len() function by overloading it with the special function. So when a pre-defined(built-in) function is declared as a special function inside a class, the interpreter executes the special function as the function definition for the built-in function's call. Let us see an example using a special function in Python.
Code:
Output:
The init method is automatically called every time an object gets created from a class. We are trying to change the default behavior of the len() function in Python, which only displays the object's length. Whenever we pass an object of our class to len(), the custom definition we have written for the __len__() function will fetch the desired results.
In our custom definition for __len__ we have added the code we want. This overloads the len() function.
Example
Using Function Overloading in Python, let us write a code to calculate the area of figures(triangle, rectangle, square). We are setting the default values of the parameters as None, and we will call the same function having varying parameters.
Code:
Output:
We have defined a function area with parameters having default values as None. This prevents an error if the function is called without any argument.
Conclusion
- Function overloading is the feature when multiple functions have the same name but the number of parameters in the functions varies.
- Python does not support function overloading. Suppose we still want to use the feature of functional overloading. In that case, we can set the default values of parameters in the method as None, which won't give an error if that specific value is not passed as an argument while calling the function.
- The latest defined function having the same name as the previous function is considered a valid function.
- Since the method arguments do not have a data type, it does not support overloading.