Hierarchical Inheritance in Java
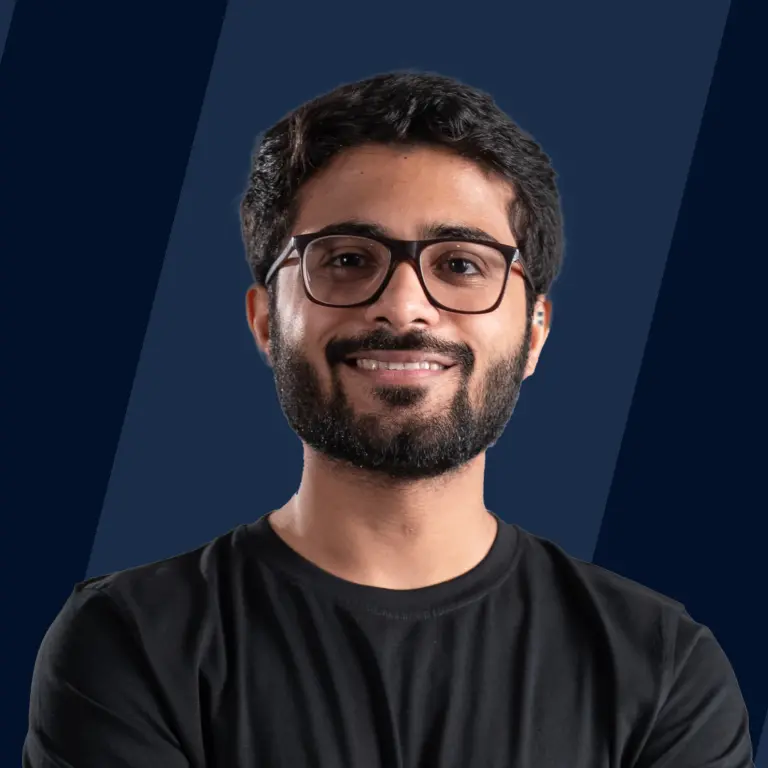
In Java, Inheritance is a core concept in object-oriented programming that facilitates the creation of new classes based on existing ones, promoting code reuse and extension. It establishes the IS-A relationship, where a child class inherits properties and behaviours from its parent. Through keywords like 'extends', Java supports five types of inheritance: single, multilevel, multiple, hybrid, and hierarchical.
Hierarchical Inheritance in Java
Hierarchical Inheritance in Java is one type of inheritance where multiple child classes inherit the methods and properties of the same parent class. As child classes get access to the methods and properties of the parent class using Hierarchical inheritance, they don't have to create those methods and properties separately; they just need to create the class-specific methods or properties.
How Does It Work?
We already know that "Hierarchical inheritance" occurs when multiple child classes inherit the methods and properties of the same parent class. In Java, this simply means we have only one superclass and multiple subclasses in hierarchical inheritance.
The idea is simple: To use the methods and properties of the parent class instead of creating them again in the sub-class. This reduces the code length and increases the code modularity.
The working of hierarchical inheritance in Java is very straightforward. We need a base class and some subclasses that inherit the base class. Hence, first, we create a base class (also called a Superclass), and then we create some subclasses that inherit that same base class. This is how we can use the code of the base class in subclasses of that base class.
Why Use Hierarchical Inheritance in Java?
In programming, code repetition is considered a bad practice as it unnecessarily increases the length of the code. A good programmer is expected to deliver clean and reusable code. You may read Clean Code in Java article to learn more about clean code in Java. Inheritance is one of the codes that can be reused to a great extent. Hierarchical inheritance is beneficial in reusing a piece of code. Now, it's time to discuss how hierarchical inheritance works in Java. Here we go.
Hierarchical Inheritance Example
For better understanding, we will start by explaining a basic example of Hierarchical inheritance in Java and then move on to a real-world example.
Basic Example of Hierarchical Inheritance in Java
Code:
Output:
Explanation:
- In the above-given example, we have one parent class(base class) called BaseClass and three subclasses that inherit the BaseClass.
- We created the object of each subclass. Then we multiplied the parentNum variable, which belongs to the parent class, with the variable of each child class.
- Even though the parentNum variable is absent in the child classes, we can access it quickly. This is just because of Hierarchical Inheritance in Java.
Remember: There is no limit on the sub-classes of one parent class. This means that any number of child classes can inherit the methods and properties of the same parent class.
Real-World Example of Hierarchical Inheritance in Java
Code:
Output:
Explanation:
- In the above-given example, we created a parent class(base class) called Vehicle and two subclasses, TwoWheeler and FourWheeler, which extend the base class Vehicle.
- Hence, these classes can access the methods and properties of the "Vehicle" class in addition to their own.
- Here, the subclasses got access to the variable basePrice and the method showPrice() of the base class.
- We created two objects, bike and car, from the subclasses TwoWheeler and FourWheeler, respectively.
- We modified the price of the bike and car by referring to the base price of the vehicle in the base class and the increasePriceBy variable of each subclass, respectively. This is how we utilized the power of Hierarchical inheritance in Java.
Conclusion
- Inheritance is one of the four pillars of OOPs(Object Oriented Programming System).
- Hierarchical inheritance is one of the types of inheritance where multiple child classes inherit the methods and properties of the same parent class.
- Hierarchical inheritance reduces the code length and increases the code modularity.
- For Hierarchical inheritance to occur, there must be at least two or more sub-classes that extend (or inherit) the same superclass.
- The base class is also called superclass or parent class, while the sub-classes are called child classes.