How to Write HTML Calculator Code?
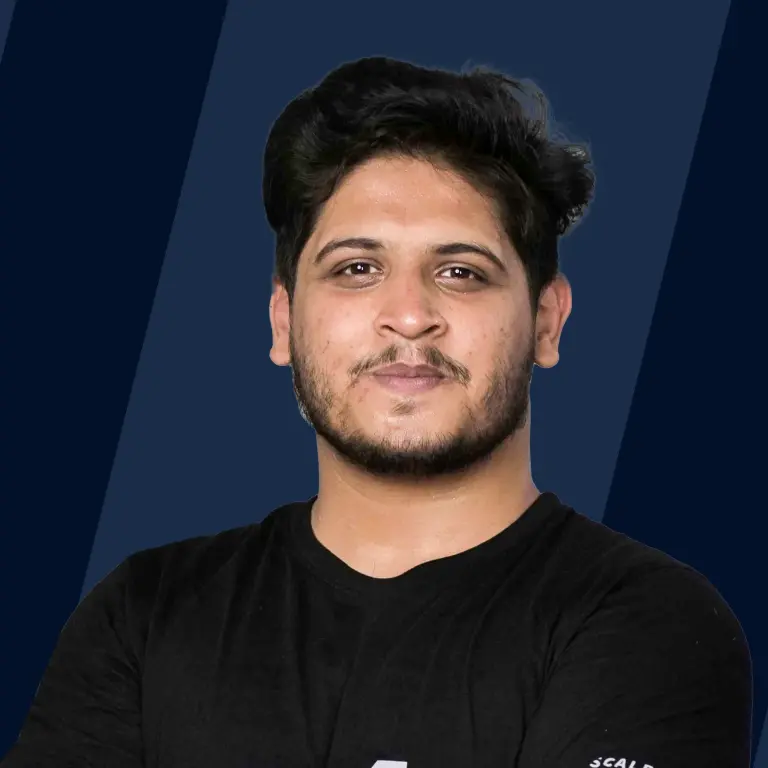
Overview
In this article, we will write a HTML calculator code that performs the basic mathematical operations like addition, subtraction, multiplication, and division. This article is a step-by-step guide that will help us create a fully working calculator using only HTML, CSS, and Javascript. To design the basic structure of the calculator we need HTML. CSS is used to apply styles on the calculator and JavaScript adds the functionality.
Through this beginner-friendly project, we shall learn about topics like event handling and DOM manipulations.
Pre-requisites
What are We Creating?
To build the basic calculator, we will write the HTML calculator code and CSS and JavaScript to it. The calculator will have the ability to perform basic functionalities like addition, subtraction, multiplication, and division.
The calculator code in HTML will contain two sections:
- Display Screen - To display the result.
- Keys - To carry out their assigned functionalities as discussed below.
Features of the Calculator
In this project, you are going to develop an HTML calculator code from scratch. The features or functionalities of the calculator include:
- Basic arithmetic operations like addition, subtraction, division, and multiplication.
- Decimal operations.
- Displaying 'ERROR' for invalid expressions.
- Displaying 'Infinity' when a number is divided by zero.
- Clearing the display screen whenever the user wants, with the help of the clear screen feature.
Components of the Calculator
The HTML calculator code contains two main sections:
- Display Screen
- Keys
- Number keys (0, 1, 2, 3, 4, 5, 6, 7, 8, 9)
- Operator keys (+, -, ×, ÷)
- Decimal key (.)
- Calculate Button / Equal key (=)
- Clear Screen Button (AC)
How to Create a Calculator with the Help of HTML?
Step 1 - Boilerplate and Including Files
At the initial step, we create a root folder 'Calculator' that contains the HTML, CSS, and JavaScript files. Name the HTML, CSS, and JavaScript files index.html, styles.css, and script.js respectively.
At the initial state, we have a standard HTML5 boilerplate. The JavaScript file script.js is included using the <script> tag, and the CSS file style.css is included using the <link> tag.
HTML
Step 2 - Adjusting the Containers
The bttns class holds all the buttons who individually have a class of btn. These divs contain the keys of the keypad, that is, operators and digits.
HTML
Step 3 - The Equal Button
The equal button signals the program to carry out the calculation. We make a separate div to include it.
HTML
Combined HTML Code
HTML
Styling the Calculator
Step 1 - Basic Styling to put Items in Place
- In the style.css file, we set a width for the container.
- We set the height and the line height equal to center the text vertically. We then set the font size to a decent amount of paddings.
- To position the buttons, we use a CSS grid so that we have 4 buttons of equal size in each row.
- For the button, we set borders and font-size. The line-height positions the item in the center vertically, and text-align: center positions it in the center horizontally.
CSS
Output
Step 2 - Better UI
To make the HTML calculator code appealing for the user, we add styling elements, for example, box-shadow to the calculator background-color to certain keys, alignment to text in the display area, and some hover effects. To have a better user experience set cursor to pointer, so the user will know that this is a clickable element.
CSS
Output
Combined CSS Code
CSS
Adding Functionality
- In the script.js first save a reference to your result dom element.
- Next we store the button references in an array. The function document.getElementsByClassName('btn') returns a NodeCollection. We use Array.from(document.getElementsByClassName('btn')) to convert this into an array.
JS
-
In the final step we add an event listener to the buttons and build the functionalities. For this, we map through the buttons array and add a click event listener for each.
-
We use e.target.innerText, to get the label of the button that was clicked and then allot functions accordingly.
- 'AC' signals to clear the display.
- If the equal (=) button is clicked the in-built eval function of JavaScript is called. To handle errors we use the try catch block.
- For all other buttons, we signal the += operator to append the clicked button's innerText to the display's innerText.
JS
Output
Combined JavaScript Code
JS
Conclusion
- A basic calculator code in HTML performs mathematical operations like addition, subtraction, multiplication, and division.
- To HTML calculator code contains the basic structure, CSS applies styles, and JavaScript adds the functionality.
- The calculator code in HTML contains two main sections:
- Display Screen
- Keys
- The different keys used are:
- Number keys (0, 1, 2, 3, 4, 5, 6, 7, 8, 9)
- Operator keys (+, -, ×, ÷)
- Decimal key (.)
- Calculate Button / Equal key (=)
- Clear Screen Button (AC)