What is Identity Operator in Python?
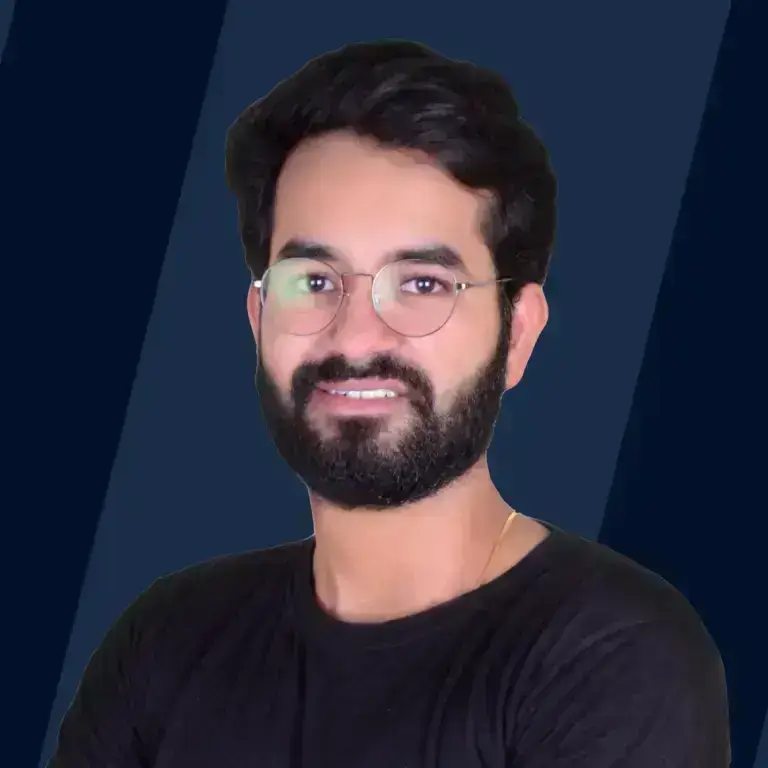
What is an Identity Operator in Python ?
Python is a purely object-based language as everything in python is an object. Every object in python is stored at a memory location. The memory location forms a unique id for the respective object. Python provides a built-in function id() to read the unique id of an object.
The syntax of id() function is :
The return value is a unique number that corresponds to the memory address of the object_name passed as an argument to the function
Example to Understand the Use of id() Function :
Code:
Output :
Notice that the id for variable string and literal "Hello" is the same, the reason for this is the memory manager in python reuses the objects instead of creating another object for the same data.
In Python, this unique id turns out to be a greatly useful property. The unique id provides a way to efficiently manage the objects in memory, assists the garbage collector to collect unused objects efficiently, and helps to check if two reference variables are pointing to same object.
Python provides operators that perform comparisons on these unique ids. These operators are called Identity Operators in Python.
The identity operator in python is useful to compare the strong equivalence of two variables. It can help to find out if two variables that may have the same name (a situation that may occur in the case of global and local variables) are pointing to the same object at the same memory location or not.
Types of Identity Operators in Python
Identity Operator in Python has two types :
- "is" operator
- "is not" operator
They are binary operators that can be used between two operands and perform a comparison on the operands returning boolean value.
is Operator
is operator is a positive equivalence checking operator. It is used to determine if two variables refer to an identical memory location or not.
is operator returns True if both operands have the same unique id (points to the same memory location).
Example : The Use of "is" Identity Operator in Python
Code:
Output :
Explanation :
The id() function shows that the vatiable_one and varaible_two are stored at the same memory location by the Python memory manager. The is operator will return true when used with variable_one and variable_two as operands. In the above example, various use cases of is operator are demonstrated.
is not Operator
is not operator is a negative equivalence checking operator. It is used to determine if two variables refer to a distinct memory location or not.
is not operator returns True if both operands are stored at different memory locations.
Example : The Use of "is not" Identity Operator in python
Code:
Output :
Explanation :
The vatiable_one and varaible_two points to the same memory location. The is not operator will return true when used with variable_one and 44 as operands since they are distinct objects stored at different locations.
Also click here to learn about Not Operator in Python.
Is There a Difference Between == and is ?
Yes, there is a huge difference between == and is operators. The is operator computes equivalence based on the unique id of a particular object. If two reference variables pointing to the same memory address are compared using is then and only then does it returns true. Since the memory address is identical it also means that the value will also be the same.
The == operator computes the equivalence based upon values and not unique ids. In a scenario where two different objects with the same value are stored at different memory locations, the == operator will return True while is operator will return False.
Example : Difference Between "==" and "is" operators
Code:
Output :
When should we use the is Operator ?
As we understood the working of is operator is based on the unique ids of its operands the best place to use is operator is when we need to check the absolute equivalence of two variables. Only if the two variables are pointing to the same object in memory then only the is operator will return true.
Another use of is operator is to compare against built-in python constants.
Use "is" operator when comparing against :
- False
- None
- True
- built-in classes int, str, etc
- NotImplemented
- debug
More Examples
Example 1 : Using "is" operator between objects of different data types
Code:
Output :
Explanation:
As the variable_one and variable_two are integer and string respectively the is operator returns False as they are stored at different memory locations.
Example 2: Using "is" operator to compare the data type of object with the class
Code:
Output :
Explanation :
The data type of variable_one is a string and is operator returns True when the type of variable_one is compared with the str class.
Learn more
Take a look at other interesting operators in Python
Conclusion
In this article we have understood :
- Every object in Python has a unique id associated with it whose value corresponds to its memory location.
- The identity operator in Python is used to perform comparisons based on the unique id.
- There are two identity operators in python :
- is : checks if operands are equivalent.
- is not : checks if operands are not equivalent.
- The == operator operates on value for checking equivalence.
- Identity operators in Python must be used when comparing against built-in classes.