What is Infinite Loop in Python?
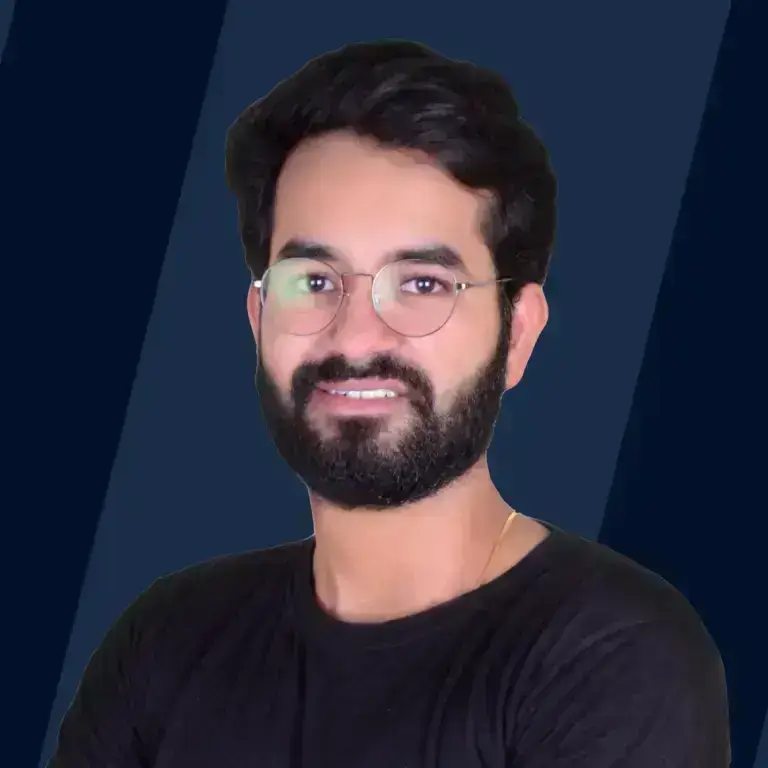
Before learning about the infinite loop in Python, let us first understand the term loop in Python.
A loop is a sequence of iterative statements or instructions that are continuously repeated until a certain condition meets. Loops are used in scenarios where we want to do certain work numerous times. So instead of writing the same code multiple times, we use a loop.
There are mainly two kinds of loops in Python namely the for loop and the while loop.
Refer to the image below to understand the working of the loop using a flow chart.
Now, coming back to the topic, an infinite loop in Python is a continuous repetition of the conditional loop until some external factors like insufficient CPU memory, error in the code, etc. occur.
There are various types of infinite loops in Python such as:
- while statement.
- if statement.
- break statement.
- continue statement.
An infinite loop does not have an explicit end (no loop termination condition is specified by the programmer).
Example:
Output:
In the above code, the Scaler Topics is printed an infinite number of times. Now, we can terminate the execution using CTRL + C so that the memory of the CPU does not get exhausted.
The output that will be shown when we terminate the execution is:
When are Infinite Loops Necessary?
In Python, an infinite loop can be useful in various scenarios. One of the prime use of an infinite loop can be seen in a client-server architecture model in networking.
As we know a server is central storage that is always awake and ready to handle client requests. So, a server runs in an infinite loop which makes it always awake and ready to handle various situations like new requests from the client, new connections from the client, etc.
Another example of an infinite loop in Python can be visualized in game development. For example, an infinite while loop is used for the main game frame which continues to get executed until the user or the game selects some other event. Since the game developer does not know the exact iterations or the time at which the user will do something, the game developer uses the infinite loop in Python.
There are several other scenarios in which we explicitly use the infinite loop in Python, refer to the following sections for examples and code implementation.
How Would an Infinite Loop be Run by Mistake?
Suppose we are given a program to print a certain thing 10 times. We can use a while loop to print the output.
The idea of the program is very simple, we will use a counter (initialized with 0) that will increment in each iteration and print the result. We can insert a termination condition that will terminate the loop once the counter reaches 10.
Suppose we have written the code correctly but missed out on the increment statement. So, the code results in an infinite loop as the counter break condition will never reach.
Let us see the implementation.
Output:
To avoid this issue, we need to increment the counter so that the loop will get terminated at a certain specified condition.
Output:
Kinds of Infinite Loop in Python
We have mainly three types of infinite loops in Python. They are:
- The fake infinite loops
- The intended infinite loops
- The unintended infinite loops
Let us discuss them one by one briefly.
1. The Fake Infinite Loops
A fake infinite loop is a kind of loop that looks like an infinite loop (like while True: or while 1:) but has some certain condition that will terminate the loop.
For example, we are given a program in which we need to print all the even multiples of 3 that is less than and equal to a given number n. Now, at the very start of the code, we cannot know the number of times we need to execute the loop.
So, we will make it an infinite loop but inside the loop, we will insert a condition that will terminate the loop when the number n is encountered.
Let us code the program for better understanding.
Output:
As we can see in the code above, we have run an infinite loop and inside the infinite loop, we have used an if statement that will work as the terminating condition of the loop.
2. The Intended Infinite Loops
In some scenarios, we need to use an infinite loop to correctly execute the program. Suppose, we need to make a counter then we must use an intended infinite loop in Python.
Let us implement the above program for better understanding.
Output:
In the above program, we ran an infinite loop and in each iteration, the timer will keep on increasing.
3. The Unintended Infinite Loops
Another variant of the infinite loop in Python can be the unintended infinite loop. As the name suggests, if we have made a silly mistake and have not added any loop terminating condition then the loop becomes an unintended infinite loop in Python.
Let us take an example program to understand the content better.
Output:
In the above program, we made a mistake by not adding any termination condition as well as we have not incremented the counter than can break the loop. So, it resulted in an unintended infinite loop.
Types of Statements in Python Infinite Loop
A statement in Python is used for creating variables or for displaying values. A statement is not evaluated for some results. Example: x = 3.
We can use the various states with an infinite loop in Python. Let us understand the various statement used with the infinite loops in Python with example(s).
1. While Statement in Python Infinite Loop
We can use the while loop in Python to create an infinite loop in Python as we have seen in the above examples. Now, we generally use two types of statements to create an infinite loop in Python. Let us see both of these statements using code examples:
a. Using while 1:
b. Using while True:
2. Using IF Statement with While Loop
Now, as we have seen above, we used the while loop to run an infinite loop in Python. Now to terminate the infinite loop, we can use an if statement that will check some conditions. Now, if the condition is satisfied, then we can perform something like terminating the loop.
Let us code an example for better understanding.
Output:
3. Using Break Statement
As the name suggests, the break statement is used to break or terminate the currently executing loop.
Let us take an example to understand the working of the break statement better.
Output:
4. Using Continue Statement
The continue statement is used to shift to the next iteration of the loop. So, whenever we want to skip a certain condition and we want to execute the rest of the execution, we can use a continue statement.
Let us take an example to understand the working of the continue statement better.
Output:
How to Create an Infinite Loop in Python?
We can create an infinite loop in Python (or other programming languages) using the while loop. In general, the for loop is not used for creating infinite loops as the for loop statement contains the termination condition.
Suppose we are given a program to print the square of a number provided by the user. Now, we can write a program something like this:
Now, the above code is completely correct but we need to run the code every time a new input has to be provided. So, to avoid such a problem, we can write an infinite loop that will print the square of a number every time the user inputs a new number.
Let us see how we can convert the above code into an infinite loop using the while loop.
Output:
Heads or Tails Game
A real-life use case of the infinite loop can be the head and tails game. Various games need a toss (random selection of head or tail) that decides which team will start the game first (for example in a cricket game, the toss decides which team will be allowed to select the batting or bowling).
So, to make a head and tail game, we can use an infinite loop that will randomly choose between head or tail every time user demands a selection.
We can use the Python random module for the same. Let us see the implementation for a better understanding.
Output:
In the above code, we are using the random module's choice() function that will randomly select among the list of choices. Now, if the user enters N or n then the if condition is satisfied and the loop is terminated.
To learn more about the random module and other modules in Python, refer to here.
Finite vs. Infinite Loop in Python
Let us now discuss the basic differences between the finite loop and infinite loop in Python.
Infinite Loop | Finite Loop |
---|---|
An infinite loop in Python iterates an infinite number of times. | The finite loop in Python iterates a finite number of times. |
Some external factors like insufficient CPU memory, error in the code, etc. can terminate the infinite loop in Python. | Finite loop in Python has the predefined terminating condition. |
An infinite loop in Python is used to run a certain program or block of code an unspecified number of times. | A finite loop in Python is used to run a certain program or block of code a certain number of times. |
Example: while True: | Example: for i in range(5): |
Learn more
To learn more about the Python programming language and other Python-related topics, please refer to the links specified below:
Conclusion
- A loop is a sequence of continuously repeated instructions until a certain condition meets. Loops are used in scenarios where we want to do a certain work numerous time
- There are mainly two kinds of loops in Python namely the for loop and the while loop.
- An infinite loop in Python is a continuous repetition of the conditional loop until some external factors like insufficient CPU memory, error in the code, etc. occur.
- An infinite loop does not have an explicit end (no loop termination condition is specified by the programmer).
- An infinite loop in Python can be useful in various scenarios. One of the prime use of an infinite loop can be seen in a client-server architecture model in networking.
- We have mainly three types of infinite loops in Python:
- The fake infinite loops
- The intended infinite loops
- The unintended infinite loops
- The continue statement is used to shift to the next iteration of the loop.
- The break statement is used to break or terminate the current executing the loop.