Java Float
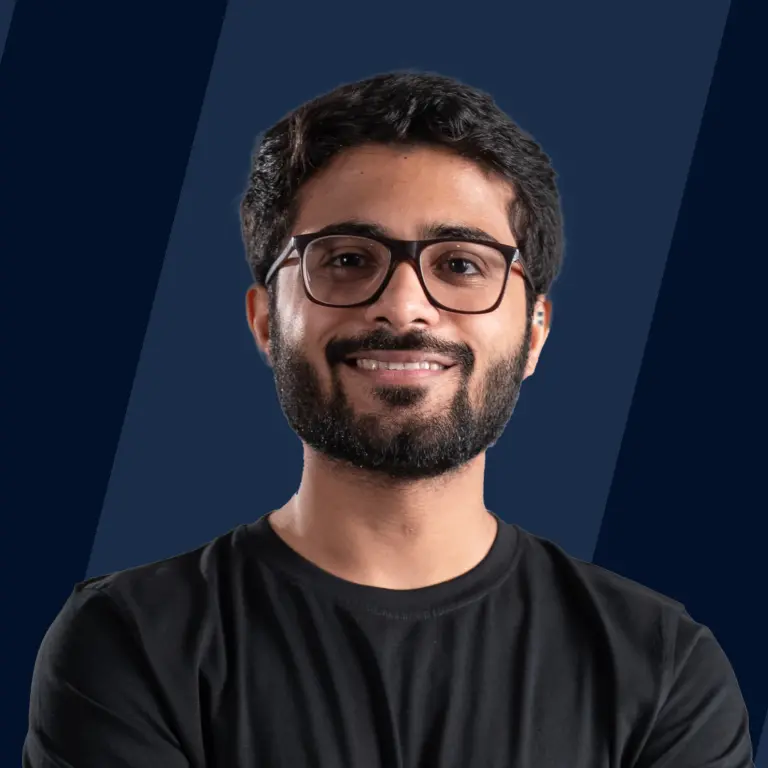
Overview
Every budding programmer who is starting to learn Java learns about some primitive data types that are used to store values that might be useful to their program. You must have used data types such as int, boolean, or char in your code along with float and double data types that are used to store fractional or decimal values that are very useful if you want to calculate let's say for example the area of a polygon or square root of a particular number or in any other case where the precision of the value is super important. If you wanna learn more about different primitive data types in java and how they work, you can start learning here.
But we are not here to focus on the float primitive data type, we are here to learn something even more exciting called the java Float class or shall I say the wrapper class for the primitive float data type. It is a way by which java provides you with a lot more methods, functionalities, and control over your data type which you can't have with the primitives.
I Know after hearing some of these terms few of you might be very confused and searching your head but don't worry, in this article we are going to break down the java float class for you in simple and easy steps, and that too in a very fun and exciting way. So without wasting any more time, let's get started.
Introduction to Java Float
In the overview section we used something called a wrapper class, but what do we mean by that? what is a wrapper class? well, a wrapper class is exactly what it sounds like, its a class wrapped or in better words surrounding your primitive data type so that it can provide you with various fields and methods that might be useful for you and also so that your primitive data type can be passed in your collections such as an ArrayList or a hashmap as an object.
every collection in Java takes in an object and not a primitive value. But now you must be wondering "Hey! that's wrong, I have made ArrayLists of ints so many times". well if you recall, you initialize your ArrayLists something like this.
Here, Integer is not the same as an int, Integer is a wrapper class for the primitive data type int, similarly, if you want to declare an ArrayList of floats, you will do it like this.
But now another question might be coming to your mind. "Okay, so if every collection takes in an object, how come I can add a primitive float to my ArrayList of Float objects??". That's a very good question and the answer is our old friend Java. So when you enter a primitive value inside of a collection, java automatically converts it to an object of the wrapper class of the same type as that of the primitive one with something called Autoboxing so that you don't have to worry about it, isn't it amazing?
now that we know what wrapper classes are and how they are useful, let's go deep inside the java float class and look at all the different fields and methods that it provides us.
Declaration
we have been talking about the java float class for so long, so let's now look at how it has been declared.
to break this declaration down for you, public means that the Float class is available to all the other classes in your program. final means that the float class cannot be extended or inherited. The Float class also extends the Number class and implements the comparable interface to perform basic comparative calculat/ions.
Constructor Summary of Java Float
Before we look at the various fields and methods of the java float class, let's understand how to create one because the java float class has some overloaded constructors.
Sr. No. | constructor | description |
---|---|---|
1 | Float(double value) | This constructor creates a new Float object with the double value that has been passed to it as an argument. |
2 | Float(float value) | This constructor creates a new Float object with the float value that has been passed to it as an argument. |
3 | Float(String s) | This constructor creates a new Float object with the String value that has been passed to it as an argument. |
Field Summary of Java Float
Java float class has some defined fields that we will look at now
Sr. No. | Field name | Description |
---|---|---|
1 | static int MAX_EXPONENT | This field gives the Maximum exponent a finite float variable may have. |
2 | static float MAX_VALUE | This field gives a constant holding the largest positive finite value of type float, (2-2-23)·2127. |
3 | static int MIN_EXPONENT | This field gives the minimum exponent a normalized float variable may have. |
4 | static float MIN_NORMAL | this field gives a constant holding the smallest positive normal value of type float, 2-126. |
5 | static float MIN_VALUE | this field gives a constant holding the smallest positive nonzero value of type float, 2-149. |
6 | static float NaN | this field gives a constant holding a Not-a-Number (NaN) value of type float. |
7 | static float NEGATIVE_INFINITY | this field gives a constant holding the negative infinity of type float. |
8 | static float POSITIVE_INFINITY | this field gives a constant holding the positive infinity of type float. |
9 | static int SIZE | this field gives the number of bits used to represent a float value. |
10 | static Class | this field gives the Class instance representing the primitive type float. |
You may have noticed that all of these fields are static, which means that they belong to the class rather than any instance of the float class. this also means that want to access any of the above-mentioned fields, you don't necessarily have to make an object of the Float class, you can use the class name directly and use the fields, something like this
if you want to learn more about the static keyword and how static fields and methods work, you can refer to this article by the scaler topics.
Method Summary of Java Float
now we will look at all the different methods that the java float class provides us and try to understand what each one of them does.
Sr. No. | Method name | Description |
---|---|---|
1 | toString() | this method returns the string representation of the specified float object that it was called upon on |
2 | valueOf() | This method returns a Float instance representing the specified float value. This method should generally be used in preference to the constructor Float(float). |
3 | parseFloat() | this method takes in a string value and returns a float value from that. can be used to get a float value from a string type value. |
4 | byteValue() | Using this technique, a primitive type float value can be extracted from a Float object. It gives back the float-typed numeric value that this object represents. |
5 | shortValue() | This procedure widens the primitive conversion and then returns the value of this Float as a short. |
6 | intValue() | After widening primitive conversion, the intValue() method returns the value of this Float as a primitive int type. |
7 | longValue() | After widening primitive conversion, the longValue() method returns the value of this Float type as a long type. |
8 | doubleValue() | After widening primitive conversion, the value of this Float type is returned as a double type. |
9 | floatValue() | After a widening primitive conversion, this method is used to return the value of this Float type as a float type. |
10 | hashCode() | This method is used to find a float value's hash code. It returns an int value of the float object. |
11 | isNaN() | A boolean value of either true or false is returned by this method. If the value of this Float is a Not-a-Number (NaN), it returns true; otherwise, it returns false. |
12 | isInfinite() | This method is used to determine whether a float value has an infinitely large magnitude. It returns either true or false as a boolean value. |
13 | toHexString() | This method is used to obtain the float argument's hexadecimal string representation. It accepts a float argument that is converted to a hexadecimal value. |
14 | floatToIntBits() | The IEEE 754 floating-point "single format" bit layout is used to obtain a representation of the specified floating-point value. A floating-point argument is required. |
15 | floatToRawIntBits() | This method returns a representation of the specified floating-point value in the IEEE 754 floating-point "single format" bit layout, with Not-a-Number (NaN) values preserved. |
16 | IntBitsToFloat() | This method is used to obtain the float floating-point value using the same bit pattern as before. According to the IEEE 754 floating-point "single format" bit layout, the argument is a representation of a floating-point value. |
17 | equals() | The equals() method compares one object to another. If the objects are the same, it returns true; otherwise, it returns false. |
18 | compareTo() | This method is used to numerically compare two float objects. If both float objects are equal, it returns 0. If one float object is less than the argument object, it returns less than 0. If one float object is numerically greater than the argument float object, it returns greater than 0. |
19 | compare() | It is used to numerically compare two float values. The value returned is the same as the value returned by. |
As you can see, the java float class provides you with so many methods that you can use in your program according to your needs.
Java Float Examples
Till now in this article, we have looked at various fields and methods that the java float class provides us, but enough with the jargon, let's look at some examples to understand the concepts a little better.
Example 1:
Let's first look at a program using a simple float variable.
Output:
Let's now look at some programs that use java float methods and fields.
Example 2:
In this example, we are going to see a java program that uses the toString() method of the java Float class and then prints the string.
Output:
Example 3:
In this example, we are going to see a java program that creates a float variable and a string variable and then uses the valueOf() method of the java Float class to convert them into a Float value and then prints the results.
Please note that the "float" is used for the Primitive float value and "Float" is used for the java Float class.
Output:
Example 4:
In this example, we are going to look at a java program that declares a string variable and converts it into a Float value by using the parseFloat() method of the java Float class.
Output:
Example 5:
In this example, we will look at a java program that showcases a lot of methods of the java float class that converts the java float variable from one type to another.
Output:
Example 6:
This java program creates a float variable, then creates a Float object using the float variable, and then uses the hashcode() method of the java float class to print the hashcode of the newly created object.
Output:
Example 7:
Float.POSITIVE_INFINITY is a static field in the java float class and it gives us a constant that holds the positive infinity value for the Java float object. Here is a Java program that will show you the field and this program also uses the isInfinite() method of the java float class.
Output:
Example 8:
In this example we will look at a java program that uses the equals() method of the java float class and used to compare two Float objects and returns true or false.
Output:
Example 9:
In this example, we will look at a java program that uses the compareTo() method of the java Float class which is also used to compare two Float objects just like the equals() method but return 1 if the first object is greater than the second object, returns 0 if both the objects are equal and returns -1 if the first object is smaller than the second object.
Output:
Conclusion
- java float class is a wrapper class around the primitive data type float which is used to store decimal or fractional values
- java float class has some overloaded constructors that can be used to create an instance of the class
- java float class also has several static fields and methods that provide the java float class with a wide range of functionalities