Packages in Java
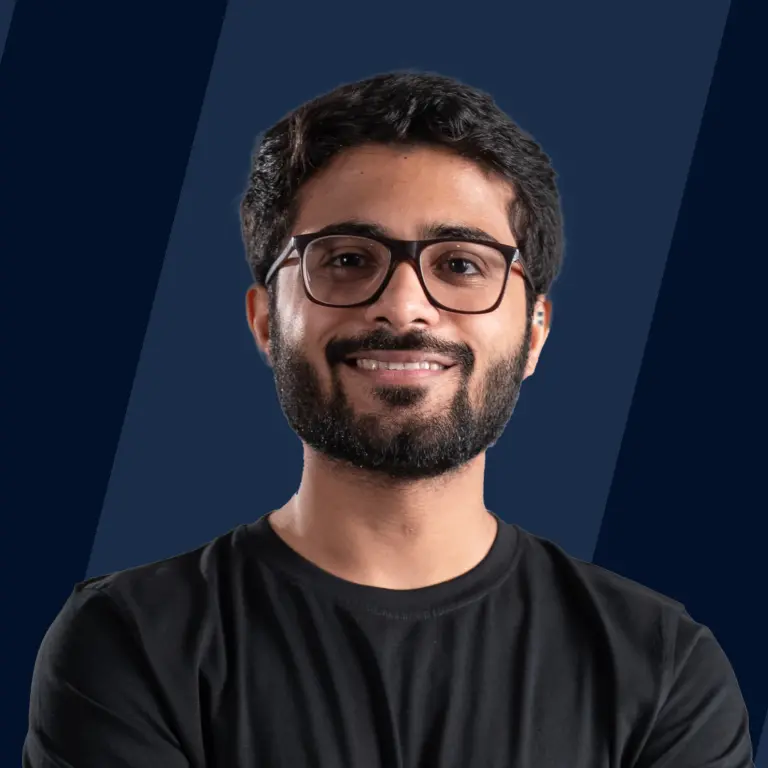
Packages in Java serve as a container for organizing classes, interfaces, and sub-packages with similar functionalities. These packages fall into two categories: built-in packages and user-defined packages. Built-in packages encompass a wide range of functionalities such as java, lang, awt, javax, swing, net, io, util, SQL, etc. User-defined packages in Java offer the advantage of structured organization and enhanced maintenance.
Advantage of Java Package:
- Structured Organization: Packages categorize classes and interfaces, facilitating easy maintenance.
- Access Protection: Packages in Java provide access control mechanisms, ensuring encapsulation and security.
- Name Collision Prevention: Packages in Java help prevent naming conflicts, ensuring clarity and avoiding ambiguity in code.
How Do Packages Work?
- Packages in java organize classes, interfaces, and sub-packages, mirroring directory structures.
- For instance, package "college.staff.cse" translates to directories "college", "staff", and "cse".
- Access to directories is facilitated via the CLASSPATH variable, ensuring easy class location.
- Package naming convention follows reverse domain notation, e.g., "org.geeksforgeeks.practice".
- To add classes, specify the package name at the file's beginning and save it in the corresponding directory.
- Sub-packages, like "java.util", require explicit import and lack default access privileges.
- Sub-package members are treated separately, necessitating explicit import for access.
Example:
Simple Example of Java Package
To compile and run this program, ensure that the directory structure reflects the package hierarchy. The "Rectangle.java" file should be saved in the "geometry" directory, and the "Main.java" file should be saved in the parent directory. Then, compile and execute "Main.java" using appropriate commands.
Output:
Accessing Classes inside a Package
Example:
Types of Packages in Java
Packages in Java can be categorised into 2 categories.
- Built-in / predefined packages
- User-defined packages.
Let’s understand them in a little more detail.
Built-in packages
When we install Java on a personal computer or laptop, many packages are automatically installed. Each of these packages is unique and capable of handling various tasks. This eliminates the need to build everything from scratch. Here are some examples of built-in packages in Java:
- java.lang
- java.io
- java.util
- java.applet
- java.awt
- java.net
Let’s see how you can use an inbuilt package in your Java file.
Importing java.lang
Output:
Explanation:
- In this example, we import java.lang.* to have access to some of the fundamental classes provided by Java, such as Math, String, and Integer.
- The code demonstrates the usage of these classes by calculating the area of a circle, determining the length of a string, and converting an integer to its binary representation.
User-defined packages
User-defined packages in Java are those that developers create to incorporate different needs of applications. In simple terms, User-defined packages are those that the users define. Inside a package, you can have Java files like classes, interfaces, and a package as well (called a sub-package).
Using Static Import
Static import is a feature in Java (introduced in versions 5 and above) that enables the usage of static members (fields and methods) from a class without explicitly specifying the class name. Here's an example demonstrating static import:
In this example, static members of the System class, specifically the out field, are imported using static import. This allows us to use out directly without prefixing it with System.
Output:
Handling Name Conflicts
Having two classes with the same name in a single file produces a name collision error, but having them on different packages can prevent it. So, packages in Java also help in preventing naming collision errors. See example.
We’ve made two packages, package1 and package2. Both packages contain a file of the same name that is sameFileName.java. Having them inside a single package causes a name collision error, and we use two packages to thwart it.
In this example, we demonstrate how to handle name conflicts in packages using the List class from both java.util and java.awt packages. By using specific import statements, we eliminate ambiguity and can use the classes without any compile-time errors.
Directory Structure
In Java, the directory structure of packages closely aligns with their names. Each package corresponds to a directory in the file system, and subpackages are represented as subdirectories. Here's how the directory structure in packages works:
- Base Directory: The base directory for packages is typically the root directory of your source code.
- Package Directory: Each package name corresponds to a directory relative to the base directory. For example, if you have a package named com.example.myapp, it will correspond to the directory structure com/example/myapp/.
- Class Files: Within each package directory, you'll find the class files (.class) corresponding to the classes in that package.
Here's an example to illustrate this:
In this example:
- src is the base directory.
- com/example/myapp represents the package com.example.myapp.
- MyClass1.java and MyClass2.java are Java source files belonging to the com.example.myapp package.
When compiling Java source files, the compiler will create a corresponding directory structure in the output directory (e.g., bin or target). The directory structure reflects the organization of packages, ensuring that compiled class files are stored in their corresponding directories.
Subpackage in Java
A package defined inside a package is called a sub-package. It’s used to make the structure of the package more generic. It lets users arrange their Java files into their corresponding packages. For example, say, you have a package named cars. You’ve defined supporting Java files inside it.
But now you want to define another package called superCars. Should we define it inside the car's package or outside as another package? At this point, you must say that it should go inside cars because superCars are also related to cars.
But to be more generic, we’re defining it as a separate package, but having it inside cars makes them part of cars.
Example
We’ve defined four packages, and some of them even have sub-packages defined inside them. We distributed Java files to each package. By this example, you can calculate the importance of giving a good name to files and placing them inside a designated package. The Sooner the application grows, the more the importance of packages and this structured format becomes prominent.
Conclusion
- Java Packages: Serve as containers for organizing classes, interfaces, and sub-packages with similar functionalities.
- Built-in and User-defined Packages: Java offers built-in packages for common functionalities, while developers can create custom packages for application-specific needs.
- Package Structure: Packages in Java align with directory structures, providing a systematic organization of code files.
- Access and Import: Packages in Java facilitate access control mechanisms and import statements, ensuring encapsulation and ease of use.
- Name Conflict Resolution: Packages help prevent naming conflicts, ensuring code clarity and avoiding ambiguity.
- Subpackages: Packages can contain subpackages, enabling further organization and abstraction within an application.