JavaScript Getter and Setter
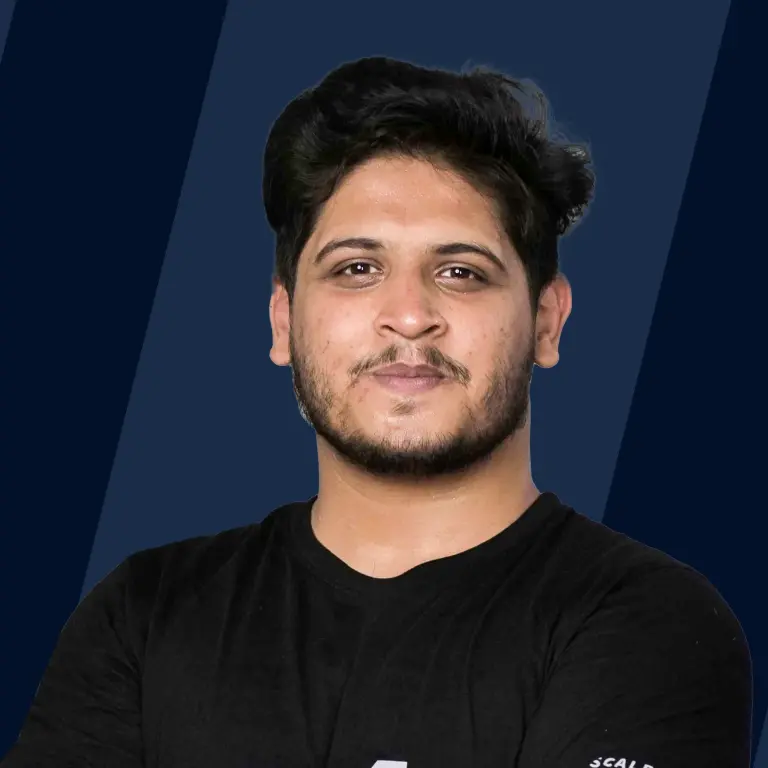
The JavaScript Object Accessors are used to access and manipulate object properties i.e. the values associated with the javascript objects. The getter and setter methods in Javascript are primarily used as object accessors. The getter and setter in JavaScript are used to alter the property values.
JavaScript Getter (The get Keyword)
The Javascript get syntax binds an object property to a function that will be called when that property is looked up.
In layman's terms, the Javascript getter provides us a way to define an Object’s property. The Javascript getter methods are mainly used to access the properties of an object.
Note: The object's property's value does not get calculated until it is accessed.
Syntax
- prop: It is the name of the property that is used to bind to the provided function.
- expression: We can also use expressions for a computed property name to bind to the given function. It is an ES6 addition.
Example
JavaScript Setter (The set Keyword)
The set syntax binds an object property to a function to be called when there is an attempt to set that property.
The Javascript set executes whenever a particular property is altered. Generally, the Setters in javaScript are used in correspondence with getters to create a type of pseudo-property. The Javascript setter methods are used to change the values of an object.
Syntax
- prop: The name of the property to bind to the given function.
- val: An alias for the variable that holds the value attempted to be assigned to prop.
- expression: From ECMAScript 2015 onwards, we can also use expressions for a computed property name to bind to the given function.
Example
JavaScript Function vs Getters
The prime difference between the function and getters is that in the function call we are accessing the function whereas, in the getter call, we are accessing the property. Thus the getter syntax turns out simpler and more readable than the function.
Example
Accessing object attribute using function:
Output:
Accessing object attribute using getter:
Output:
Explanation of the example:
In the above example, we have created an object Student with properties like firstName, lastName, rollNo. Now the 'displayName method' and 'displayName getter' are respectively used to access the name of the student. We can observe that the 'displayName method' is returning player.myFunc() which is a function whereas 'displayName getter' is returning player.myFunc which can be used as a property.
Data Quality While Using Getters and Setters
The Getters and Setters are used to secure better data quality. The getter and setter methods in JavaScript provide centralized control of how a certain field is initialized and provided to the user, thus making it easier to verify and debug.
Example
Output
Why Use Getters and Setters?
- Accessor and Mutator methods encapsulate our classes, keeping them safe by hiding unnecessary implementation details and only modifying certain values.
- Get and Set methods to have a slightly simpler syntax and also allow us to recognize their purpose at a glance.
- The syntax for getters and setters is similar to that of the javascript method thus it is easy to learn.
Smarter Getters/Setters
Getter and setter in Javascript can be used as wrappers over “real” property values. This is done to gain more control over operations with them using which we could add constraints etc.
For example, we can restrict users from keeping too short names for the name property in the above-discussed example.
Example
Output:
Explanation of the example:
In the above example, we have wrapped the setter with a constraint that if the length of the value passed to it is less than 4 then it prompts an alert. Thus when the user.name is assigned 'p' it alerts the message. user.name = "Peter" works fine as its length is greater than 4.
Note:
Technically, external code is able to access the name directly by using user.name. But there is a widely known convention that properties starting with an underscore "" are internal and should not be touched from outside the object.
Using for Compatibility
The Getter and Setter in Javascript can be used to allow to take control over a “regular” data property at any moment. It is done by replacing the regular data property with a getter and a setter and altering its behavior.
Example
Let's suppose that we are creating User objects with name and age properties.
But for some reason, we have decided to use birthday instead of age.
Now, what do we do of the age property that would have been used in the earlier code chunks? The naive method would be to replace all occurrences of age with birthday. But this would take a lot of time.
Adding a getter solves this issue. The age is now calculated from the current date.
Output:
Object.defineProperty() in JavaScript
The Object.defineProperty() method in JavaScript is used to define a new property directly on an object. The Object.defineProperty() returns an object. It is generally used to change the flags of the attributes.
Syntax
- Obj: It is the Object on which the property is defined.
- Prop: It is the name of a property to be defined or modified.
- Descriptor: It is the descriptor for the property being defined or modified.
Example
Output:
Explanation of the example
In the above example, we are defining a new property ' city' using Object.defineProperty whose value is set to be ' California. Thus upon assigning the property using ' student.city' the object will be assigned the property.
Browser Support
Browser | Chrome | Firefox | Opera | Safari | Edge |
---|---|---|---|---|---|
Version | yes | yes | yes | yes | 9.0 |
- The first column contains names of web browsers and the rows contain the versions that support getters and setters.
- The version mentioned in the row is the minimum version required to run the program.
- If the row has yes then the getter and setter are supported by all versions of the browser.
Conclusion
- The Javascript Object Accessors are used to access the properties of objects.
- The getter and setter in Javascript are used to access and manipulate the object properties.
- Accessor and Mutator methods encapsulate our classes, keeping them safe by hiding unnecessary implementation details and only modifying certain values.
- The Enumerable Attribute in Javascript is used to determine if a property is accessible when the object’s properties are enumerated by the for...in loop.
- The Javascript object's properties can be deleted or modified only if the configurable attribute for the object is true, otherwise, we cannot alter the attributes of the object.
- The Object.defineProperty() method in Javascript is used to define a new property directly on an object.
- The Getter and Setter in javascript can be used to allow to take control over a regular data property