What is Membership Operator in Python?
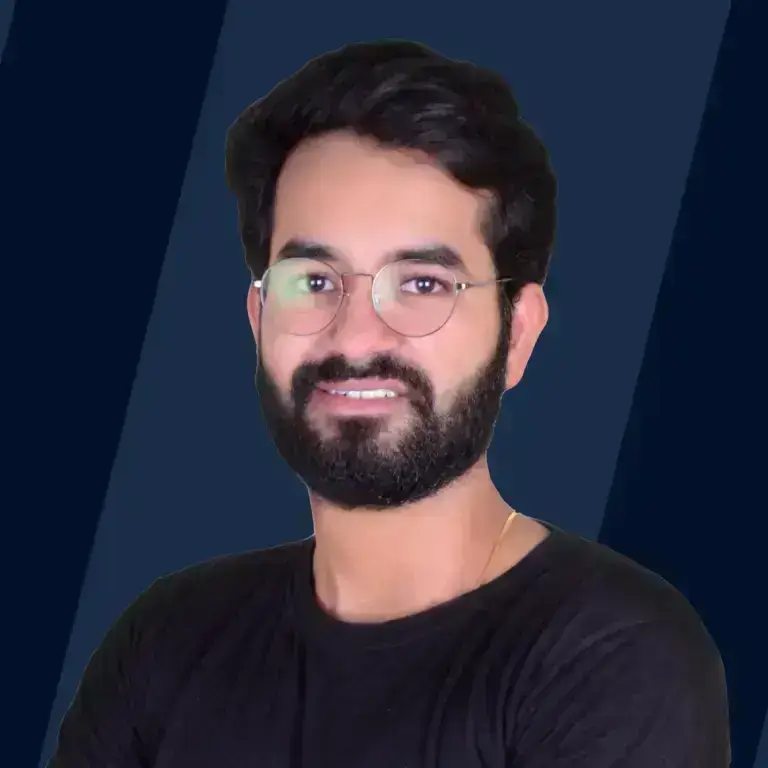
Membership operator in Python test a sequence, such as a string, a list, or a tuple, for membership. They check whether a sequence appears in an object or not. There are two membership operators in Python. To check if any sequence is present in any object, we use in, and to check if a sequence is not present in any object, we use not in. We get the output in the form of a boolean value that is True or False.
- in
- not in
Let's see what they do.
List of Membership Operators in Python
The list of membership operators that are present in Python is :
Operator | Description |
---|---|
in | By using the in operator, one can determine if a value is present in a sequence or not. If the specified variable/literal is found, it will return true, otherwise, it will return false. |
not in | By using the, not in operator, one can determine if a value is not present in a sequence or not. If the specified variable/literal is found, it will return false, otherwise it will return true. |
Now, Let's take a look at some python programs to understand them in a better way.
Examples of Membership Operators in Python
1. in Operator
A collection such as a list, set, string, or tuple can be checked for membership by using the in operator.
Example 1: Check if the number is present in the list or not
Code:
Output:
Explanation:
In the above code, the in operator on the third line checks the occurrences of number 29 in the list named list_1, and since it is present in the list, it gives output as True.
Example 2: Checking if the string is present in the list or not
Code:
Output:
Explanation:
In the above code, the in operator inside the print statement checks the occurrence of string ram in the list named list_1, and since it is present in the list, it gives output as True.
Example 3: Checking a character in String
Code:
Output:
Explanation:
In the above code, in the first line, the in operator checks the occurrence of character l in the string, and since it is present in the string, it gives output as True. But for character b in the second line, it does not encounter the occurrence, and hence, we get False as output.
Not only this, but a collection such as a set or tuple can also be checked for membership by using the "in" operator. Let's have a look at the in operator in set.
Example 4 : Using "in" operator with sets
Code:
Output:
Explanation:
In the above program, the in operator checks the occurrence of a number in the first line, and since it finds it, it gives True as an output, but for string on the second line inside a set, it does not encounter string Python inside the set hence, the output we get is False.
2. not in Operator
The not-in operator in python checks whether an object is not in a collection.
Let's take a look at the same examples that we took for the in operator but by using the not in operator.
Example 1: Checking if the number is present in the list or not, using the not in operator
Code:
Output:
Explanation:
In the above code, the not in operator in the third line checks the occurrences of number 45 in the list named list_1, and since it is not present in the list, it gives output as True.
Example 2: Checking if the string is present in the list or not, using the not in operator
Code:
Output:
Explanation:
In the above code, the not in operator inside the print statement checks the occurrence of string ravana in the list named list_1, and since it is not present in the list, it gives output as True.
Example 3: Checking if a character is present inside a string or not, using the not in operator
Code:
Output:
Explanation:
In the above code, the not in operator checks the occurrence of character z in the string, and since it is not present in the string, it gives output as True. But in the second line, it encounters S in the string therefore, it gives output as False.
Not only this, but a collection such as a set or tuple can also be checked for membership by using the "not in" operator. Let's have a look at the not in operator in the set.
Example 4: Using the "not in" operator with sets
Code:
Output:
Explanation:
In the above program, the not in operator checks the occurrence of number 4 in the first line therefore, it gives True as an output, but for the string the on the second line inside a set, it encounters the, and since it finds it, it gives False as an output.
Identity Operators In Python
Apart from the membership operator in Python, Python also contains a type of operator called Identity Operators. By using identity operators, Python checks if a particular value is of a particular class or type. An identity operator is used most commonly to determine the type of data a variable contains. Python offers two different identity operators.
- is operator
- is not operator
Let's have a look at how these two identity operators are used, along with their brief explanation.
1. is Operator
Python's is operator returns True when the type on both sides is the same. If not, it returns False.
Let's look at a python program to understand this better.
Example: To check if a variable is of type str or not.
Code:
Output:
Explanation:
In the above Python program, we are checking the data type of o, if it is a string or not. Since it is true, so it returns True.
2. is not Operator
The is not operator does the opposite of the is operator. If the data type of a variable does match the type written on the other side, it returns True. Otherwise, it returns False.
Let's look at a python program to understand this better.
Example: To check if a variable is not int type.
Code:
Output:
Conclusion
So, let's summarize what we have learned in this article, the takeaways from the article are given below :
- We use membership operators in Python to check whether a sequence is present in the object or not.
- To check the membership, we have two types of membership operators in Python, in and not in.
- The in operator returns True if it encounters the sequence in any object, whereas not in is the total opposite.
- Apart from the membership operators in Python, Python also has identity operators, which are somewhat similar, but they check whether a particular variable is of a particular class or type.
- To check identity, we have two types of identity operators in Python, is and is not.
- The is operator returns True if the type on both sides is the same, whereas is not is opposite to it.