What are Mutable Data Types in Python?
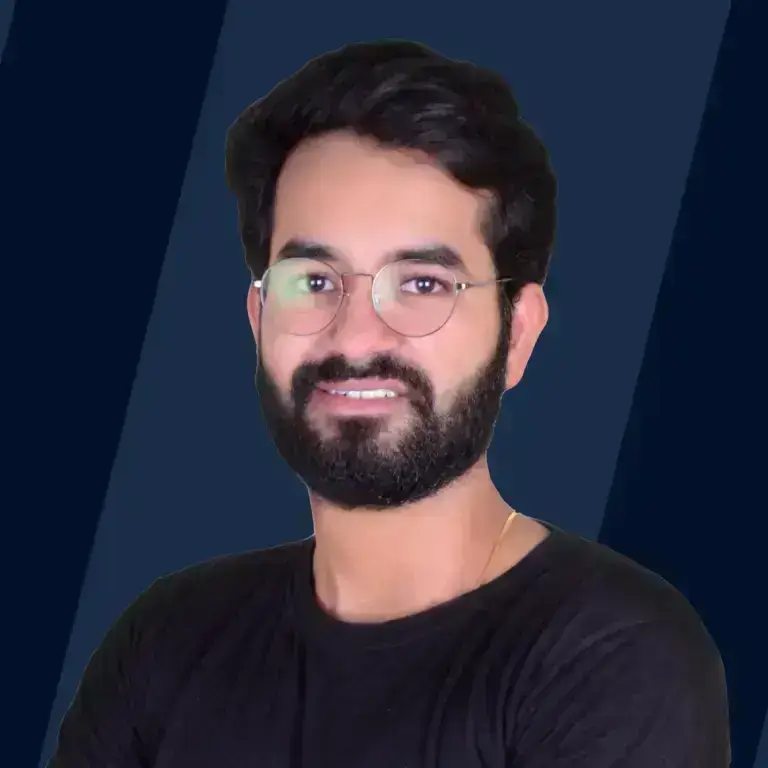
Programmers often love coding in Python and beginners love learning Python because of its simplicity. Also, we must be aware that everything in Python is an object. However, there is an important and notable difference between different types of objects in Python. And that is, some objects in Python are mutable, whereas, some are immutable. And this difference causes a lot of confusion among new Python developers. Hence, in this article, we are going to clear this confusion. In this article, we are going to learn about what are mutable data types in python.
Basically, mutable data types in Python are those whose value can be changed in place after they have been created.
This statement might have added two more questions to your mind - What is "in-place"? And, what kind of "values can be changed" ??
Let us discuss each of them.
1. What is "in-place" ?
By in-place, we mean that, any operation that changes the content of anything without making a separate copy of it. For example, we can directly add, remove, or update the values of a list in Python, without creating a new list.
For example, in the above image, we are able to append new values to our list lst without having to create another list. Hence we can overwrite the original list. Hence, it is an in-place operation.
2. What kind of values can be changed in mutable data types?
In mutable data types, we can modify the already existing values of the data types (such as lists, dictionaries, etc.). Or, we may add new values or remove the existing values from our data types. Basically, we may perform any operation with our data without having to create a new copy of our data type. Hence, the value assigned to any variable can be changed.
Examples of Mutable Data Types in Python
There are basically 3 mutable data types in Python:
- List
- Dictionary
- Set
Let us discuss each of them in brief.
List in Python
A list data structure is a ordered sequence of elements in Python, that is mutable, or changeable. This is because we can change the value of a list in Python by assigning a new value to our already existing list. We need not create any separate (or new) copy of the list to perform these operations. A few examples for the same are given below.
Example 1:
Output:
Explanation: In the above list, we have performed multiple operations to demonstrate that we can change the value of an already existing list
- Initially we created a dummy list
- Then appended a value to our list, and printed the updated list where our added value was reflected.
- We also performed operations like extending values and removing values from our original list.
- As an output, all the performed changes were reflected.
- Hence, we may conclude that lists in Python are mutable.
We can also change the value of the list by using assignment operator in python. For example, consider the below code:
Example 2:
Output:
Explanation : In the above example, we have modified the value of our list using the indexing operator.
Set in Python
A set is an unordered collection of items in Python. All the elements of the set are unique in nature, that is, there are no duplicates in a set. Also, the elements of the set are immutable in nature, that is, they cannot be changed.
However, a set itself is mutable in Python. That means, we can add or remove elements from a set in Python. Hence, we can perform operations in a set that can modify the overall set.
A few notable points regarding the mutability of sets in Python are:
- Since sets are unordered in nature, we cannot apply indexing to them.
- We cannot use indexing or slicing to fetch (access) or change the elements of a set. In fact, the set data type itself does not support these operations.
- However, we may add, update or remove any element from the set. The addition of elements can be performed using the add() method in Python. And, updating operations can be performed using the update() method. We can use the remove() in Python to remove elements from a set.
Let us look into a few examples to clear our above-discussed points.
Example:
Output:
Explanation : In the above example, we have added, updated, and removed values from our set. Please note, that the result each time is in an unordered fashion. That is the major reason we cannot use indexing to change any set element, because the position of any element in the set is not fixed, or not in an ordered fashion.
Please Note: We can also remove the elements from a set using the pop() method and the clear() method.
Dictionary in Python
Dictionary in Python is an unordered collection of items. Each item of a dictionary has a key/value pair, using which we can access a particular key or value of a dictionary. Keys in dictionaries are unique in nature.
Dictionary is a mutable data type in Python. That means, we can modify, remove or add values of existing items in a dictionary using the assignment operator.
The addition of items can be performed by using the keys of the dictionary. If the item is already present, then simply the value gets updated. Removing elements in a dictionary can be done simply using pop(), popitem(), clear() or del() in Python.
Let us look into some examples to understand what we discussed above.
Example:
Output:
Explanation: In the above example, we performed operations like adding, updating, and removing elements from our dictionary. This proves that dictionary is a mutable data type in Python.
Mutable vs. Immutable Objects
We saw earlier in this article, that we can change the contents of a mutable data type in Python, by assigning new values or by simply adding new values. On contrary to that, we cannot change the contents of an immutable data type in Python.
Before lining the differences between them, let us first get a short idea about immutable objects in Python --
In simple words, the value assigned to a variable cannot be changed for the immutable data types. For example, String is an immutable data type in Python. We cannot change its content, otherwise, we may fall into a TypeError. Even if we assign any new content to immutable objects, then a new object is created (instead of the original being modified).
Python handles mutable and immutable objects quite differently. Let us look into the difference between both of these types of objects:
Mutable Objects | Immutable Objects |
---|---|
A mutable object can be changed after it is created | An immutable object cannot be changed after it is created |
Examples : List, Set, Dictionary | Exg: tuples, int, float, bool, frozenset. |
Mutable objects are not considered as thread-safe in nature | Immutable objects are regarded as thread-safe in nature |
Mutable Objects are slower to access, as compared to immutable objects | Immutable objects are faster to access when compared to mutable objects |
Mutable objects are useful when we need to change the size or contents of our object. | Immutable objects are best suitable when we are sure that we don't need to change them at any point in time. |
Changing mutable objects is a cheaper operation in terms of space and time | Changing immutable objects is an expensive operation since it involves creating a new copy for any changes made. |
Learn More
I encourage you to go ahead and pick one of the scaler articles mentioned below to learn more about mutable and immutable data types in Python:
Conclusion
In this article, we learned about mutable data types in Python. Let's take a brief pause and reflect on what we have seen so far!
- Objects in Python can be either mutable or immutable.
- Mutable data types are those whose values can be changed or new values can be assigned to them
- List, Sets, and Dictionary in Python are examples of some mutable data types in Python.
- Immutable data types are those, whose values cannot be modified once they are created. Examples of immutable data types are int, str, bool, float, tuple, etc.
- We should consider mutable objects whenever we are certain that the size of our data might change. Whereas, immutable types are preferred whenever we have data of some constant size. This is because making changes in an immutable data type is a costly operation.