frozenset() in Python
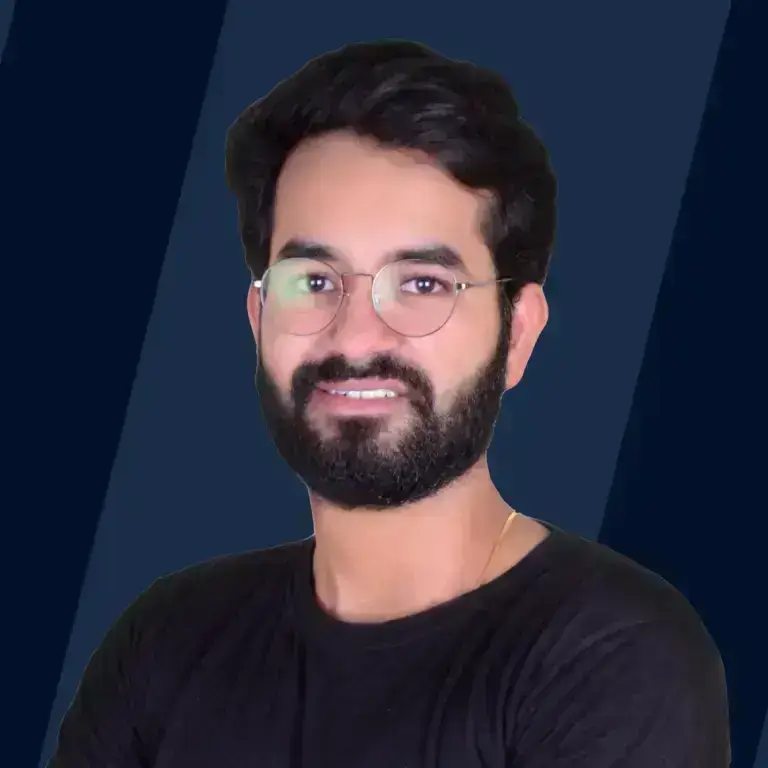
Overview
In Python, frozenset() is a function that takes an iterable and returns its frozenset object counterpart. A frozenset object is an immutable, unordered data type. A frozenset contains unique values, which makes it very similar to set data type. It is immutable like tuples.
Syntax of frozenset in Python
frozenset() has a single parameter, and that can be any iterable.
Parameters of frozenset in Python
The frozenset() function accepts only one parameter, i.e., an iterable (list, tuple, string, dictionary, or a set).
Return value of frozenset in Python
The return value of the frozenset() function is a frozenset object (an immutable, set like data type).
Example
This example is to give an idea of the implementation of frozenset in Python:
Output
The above example converts a list into a frozenset using the frozenset() function. This example was to give a basic idea of how the frozenset works.
What is frozenset in Python?
The frozenset() is an inbuilt function in Python, which takes an iterable object and returns its frozenset object initialized with elements from the given iterable. Frozenset objects and sets in Python are very similar. Both are unordered and unindexed. Frozenset objects are immutable (they can't be changed). The order of elements is not guaranteed to be preserved.
Since the frozenset is an immutable data type, it can be used in cases where we have to create a group of keys (or some other data) that we don't want the user to change, as frozenset doesn't allow changes.
Now we will look at some examples for frozenset to understand its implementation.
Examples of frozenset in Python
When no parameters are passed to the frozenset
Output
In the above example, we are initializing a frozenset with no iterable passed. As we can see in the output, the result is an empty frozenset object.
Using frozenset with dictionary in Python
Output
In the above example, we initialize a frozenset object using a dictionary. As we can see in the output, the result is a frozenset object with the keys of the dictionary as its elements.
Error warning: item assignment in frozenset object
Error:
In the above example, we initialize a frozenset object using a dictionary. We are assigning the frozenset a value "work" at index 1. As we know, a frozenset is unordered, so its index 1 doesn't make any sense, and it is immutable (we can't modify its elements), which is why it throws the error "frozenset object does not support item assignment".
Frozenset operations in Python
Now we will discuss some operations which can be performed on frozensets. Like sets, frozensets can be also perform operations like copy, difference, union, intersection, and symmetric_difference.
Let's have a look at the implementation of each operation:
Copy operation in frozenset
The copy operation in frozenset is used to copy the whole frozenset passed to it and return that to another variable.
Syntax: The frozenset to be copied is written before .copy(); frozensetObjectName.copy()
Output
In the above example, we are copying the frozenset object "frozenDict" to the variable "A", and then we are printing it.
Difference operation in frozenset
The difference operation in frozenset is used to return a frozenset object after removing the common values with another frozenset.
Syntax: The frozenset to be subtracted from (frozen1) is written before .difference() and the frozenset to be subtracted (frozen2) is passed to the difference method; frozen1.difference(frozen2)
Output
In the above example, we find the difference between frozen1 and frozen2 using the .difference() method. The resultant frozenset contains the values present in frozen1 except for common values of frozen1 and frozen2.
Union operation in frozenset
The union operation in frozenset is used to find the union of two frozensets (elements present in either of the frozensets or both frozensets). Using this method, we can find the union of two frozensets (frozen1 and frozen2).
Syntax: One frozenset is passed to the union method and the other is written before .union(); frozen1.union(frozen2)
Output
In the above example, we find the union of frozen1 and frozen2 using the .union() method in python. The resultant frozenset contains all the elements present in either of the sets and both of the sets.
Intersection operation in frozenset
The intersection operation in frozenset is used to find the intersection of two frozensets (only the elements present both frozensets). Using this method, we can find the intersection of two frozensets (frozen1 and frozen2).
Syntax: One frozenset is passed to the intersection method and the other is written before .intersection(); frozen1.intersection(frozen2)
Output
In the above example, we find the intersection of frozen1 and frozen2 using the .intersection() python method. The resultant frozenset contains only the elements present in both of the sets.
Symmetric difference operation in frozenset
The symmetric difference operation in frozenset is used to find the symmetric difference between two frozensets (elements present in either of the frozensets except for their common elements). It is the opposite of the difference operation. Using this method, we can find the symmetric difference between two frozensets (frozen1 and frozen2).
Syntax: One frozenset is passed to the symmetric_difference method and the other is written before .symmetric_difference(); frozen1.symmetric_difference(frozen2)
Output
In the above example, we find the symmetric difference between frozen1 and frozen2 using the .symmetric_difference() python method. The resultant frozenset contains all the elements present in the sets except their common elements.
Conclusion
- A frozenset() function returns a frozenset object of the iterable passed to it.
- The frozenset object is an immutable, unordered, set-like data type.
- The frozenset is used to bar the user from changing a data set.
- We can't assign items to a frozenset as it is an immutable data type (which can't be modified).
- We can perform different operations like copy, difference, union, intersection, and symmetric_difference on frozenset objects.
See Also:
If you need some help with this article or want to move forward, refer to these topics: