Object in Java
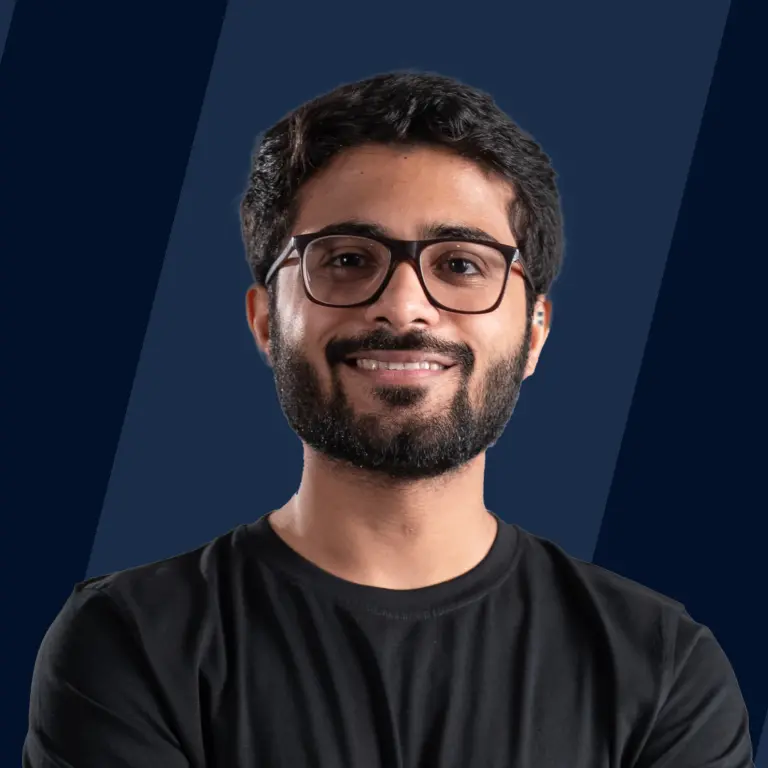
Overview
An object (also known as an instance), is a member of a Java class, with its own identity, behavior, and state. Basically, the state of an object is stored in a variable, whereas the behavior of an object is displayed using functions (methods). Objects are created during runtime, and those objects are also known as classes. They also allocate memory and space addresses. A new object in Java is created using the new keyword.
So let us now begin with the main agenda of our article, object in java.
What is an Object in Java
As we know java is an object-oriented programming language and classes and objects are the building blocks of an object-oriented programming language. So, in this topic let us learn about what is an object in Java.
An object is a member of a Java class, which has its own identity, behavior, and state. An object is also known as an instance of a class because a class is a blueprint from which an object is created during runtime. Basically, an object is created using the new keyword and the state of an object is stored in a variable, whereas the behavior of an object is displayed using functions (methods).
How to Create Objects in Java?
There are several ways of creating an object in Java, they are :
- Using a new keyword.
- Using the newInstance() method of the Class.
- Using the newInstance() method of the Constructor Class.
- Using object serialization and deserialization.
- Using the clone() method
For Details Read, How to Create Object in Java?
Using a new keyword
In Java using a new keyword is the most common and basic way of creating an object. Using this new keyword we need to call the constructor of the class with a parameter or with no parameter. The new keyword allows us to create a new object.
Syntax:
Let us now look at the syntax of how we can create an object using the new keyword.
Explanation:
In the above syntax, we are using the new keyword to create the object of the class class_name, we can also observe, how the constructor of the class is been called using the new keyword.
Example
Let us now see an example code of how we can create an object using the new keyword.
Code:
Output:
Explanation:
In the above code, we are creating an object (instance) of the class MyClass using the new keyword. We are printing the instance variable of the class by calling it through the object with a dot (.) notation.
Using the newInstance() Method of the Class
In this method, we need a no-argument constructor which is been called by the newInstance() function to create an object. Since this newInstance() method belongs to the java.lang.reflect.Constructor class, this method is also considered a reflective way to use it for creating an object.
Syntax:
Let us now look at the syntax of how we can create an object using the newInstance() method of the class.
Explanation:
In the above syntax, we are using the newInstance() method to create the object. To load the class dynamically in the memory, Class.forName is called. We can also see there are some exception errors thrown, Let us understand, why this is so.
- If the class or method is not accessible, it will throw the IllegalAccessException error.
- If the Class represents a primitive data type, an interface, an abstract, an array class, or if the class does not have a no-arg constructor, it will throw an InstantiationException error message.
Example
Let us now see an example code of how we can create an object using the newInstance() method.
Code:
Output:
Explanation:
In the above code, Firstly, we are storing the class in memory dynamically by calling the Class.forName method and giving the class name inside it as a parameter. We are creating an object (instance) of the class MyClass using the newInstance() method. We are printing the instance variable of the class by calling it through the object with a dot (.) notation. There is also some exception handling block catch block.
Using the newInstance() Method of the Constructor Class
This method also uses the newInstance() method, in which we need a constructor, but this time with a parameterized constructor to create an object.
Syntax:
Let us now look at the syntax of how we can create an object using the newInstance() method of the Constructor Class.
Explanation:
In the above syntax, we have a newInstance() method to create an object with a parameterized constructor. The following exceptions are returned by the newInstance() method:
- the method will throw an IllegalAccessException if the constructor cannot be accessed.
- The method will throw an IllegalArgumentException if the number in the formal and actual parameters are different.
- the method will throw an InvocationTargetException if the constructor throws an exception.
- the method will throw an ExceptionInInitializerError if the provoked initialization fails.
Example
Let us now see an example code of how we can create an object using the newInstance() method of the Constructor Class.
Code:
Output:
Explanation:
In the above code, we are creating objects using the newInstance() method of the Constructor Class. There is also some exception handling block catch block.
Using Object Serialization and Deserialization
When we serialize and then deserialize an object a new separate object is created. In this method to create an object, we do not need any constructor. For serialization and deserialization, we must use the Serializable interface in Java.
Object serialization:
Serialization is basically used for converting an object state into a byte stream. To serialize an Object we use the writeObject() method.
Syntax when serializing an object
Explanation:
In the above syntax, we are using the writeObject() method to serialize the object. This method will also throw an IOException error if the input object is not correct.
Object deserialization:
Deserialization is basically used for recreating an object by using the byte stream in Java. To deserialize an Object we use the readObject() method.
Syntax when deserializing an object
Explanation:
In the above syntax, we are using the readObject() method to deserialize the object. This method will also throw an IOException error if the input object is not correct.
Example
Let us now see an example code of how we can create an object using the serialization and deserialization method.
Serialization: Serialization is used to convert the object format to a byte of streams to save the state of the object.
Deserialization: Deserialization means the opposite of it. Here we use the byte of streams, that is, files to retrieve the saved state of the object.
Code:
Output:
Explanation:
In the above code, we are creating objects by serializing and deserializing the object. In the main class we have implemented the Serializable interface. Inside the public class at first, we are creating an object of the main class, and then we are creating a filename that should have .ser. Then we start the serialization process, inside the try block, at first, we save the object in the file, then the object is been serialized with the writeObject() method, and our serialization process is done. It also contains the catch block to handle the IOException error. Now after serialization, we are starting the deserialization process for the object. Here, inside the try block, at first, we are reading the object from the file, then we are deserializing the object using the readObject() method, and then the file is closed. It also contains the catch block to handle the IOException and ClassNotFoundException errors.
Using the clone() Method
Whenever the clone() method is called, it creates a new object and then gets all the content in the old object copied to it. We do not need a constructor to call the clone() method. We usually implement the Cloneable class to use the clone() method.
Syntax:
Let us now look at the syntax of how we can create an object using the clone() method.
Example
Let us now see an example code of how we can create an object using the clone() method.
Code:
Output:
Explanation:
In the above code, we are creating an object by cloning another object's content inside it with the help of the clone() method.
Characteristics of Objects in Java
Just like in the real world, there are different objects like cats, dogs, mobile phones, pens, cars, etc. They have their own identity, behavior, and state, the object in Java is also very similar to these real-life objects, and it also has these characteristics. So, let us understand these characteristics in detail, and how objects are defined with them.
Identity :
Basically, to make the object uniquely identifiable and also enables one object to interact with other objects, these identity characteristic are used - such as an address in memory or a random ID number. Suppose, in the real world different cars can have the same state (color, brand, mileage) and behavior (transportation) but they must have some unique feature that makes them different (that item’s manufacturing ID).
State :
The state of an object is represented by the data members of the object, and it is stored in a field (variable). Basically, it defines the property or individual characteristics of an object. For example the color, brand, and mileage of a car. Here, we are supposing the car as an object and its features like color, brand, and mileage as the state of the object.
Behaviour
The behavior of an object is represented by the methods of the object, which operate its internal state. Basically, it reflects the response of an object to other objects. For example, the car runs on petrol or diesel, or a car is used as a mode of transport. Here, we are supposing the car is an object and it runs on petrol, and its transportation is the behavior of the object which is defined inside the method of the object.
Example of a car object:
Identity | State | Behaviour |
---|---|---|
Manufacture ID | Color | Runs in petrol |
Number Plate | Brand | mode of transportation |
Model name | Speed and mileage | Drifting |
Example for the Object in Java
Let us now look at some examples of objects in Java.
Code:
Output:
Explanation :
In the above code, we are creating an object of the Dog class inside the main() method. Which consist of breed, age, size, and color as the state of object, and some methods like getInfo, eat, and sleep. Here, we can see the use of different Characteristics of objects. We have also created 2 different objects of the same Dog class.
Conclusion
In this article, we learned about the object in java. Let us recap the points we discussed throughout the article:
- An object is a member of a Java class, which has its own identity, behavior, and state.
- An object is also known as an **instance of class**because a class is a blueprint from which an object is created during runtime
- An object is created using the new keyword. Using this new keyword we need to call the constructor of the class with a parameter or with no parameter.
- We can also create an object using the newInstance() method of the Class. Using this method we need a non-argument constructor to create an object.
- We can also create an object using the serialization and deserialization method, where at first we need to serialize the object, then deserialize it.
- We can also use the clone() method to clone the content of an object to another object.
- Few characteristics of an object in java are its identity, behavior, and state. We usually store the state of the object in a variable, the behavior of the object is defined inside a method, and the identity is the unique feature of the object like its name.
- We have also seen an example of an object in Java, to make it more clearly understandable.