Implicit Type Conversion in Python
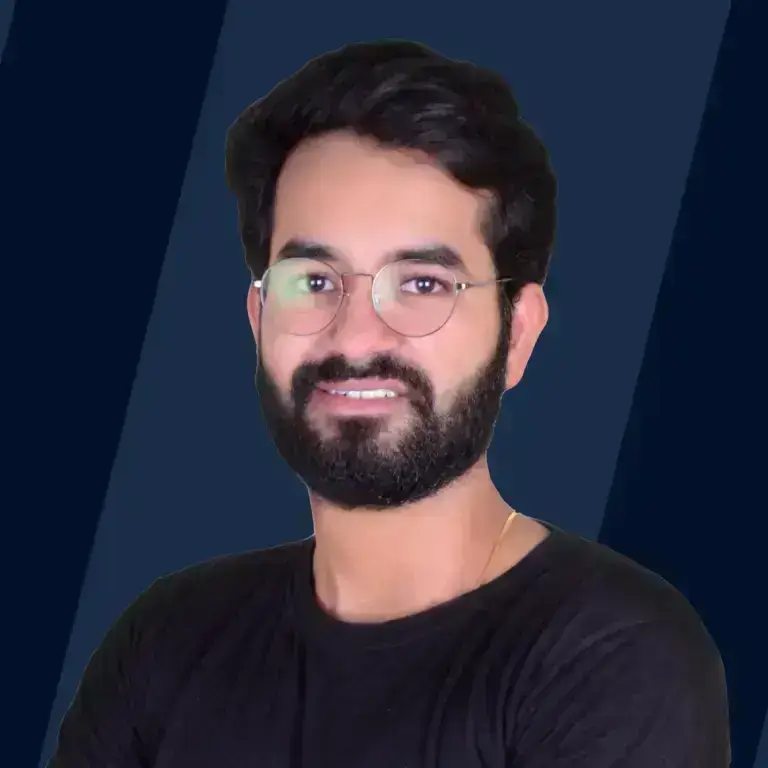
Overview
Type conversion in Python refers to converting the value of one data type into another data type. In Python, there are two ways to convert data types. In Implicit type conversion, Python automatically converts one data type to another data type without any user's need.
Note: Before going on to read about type conversion, give a quick read to Python Data Types.
Introduction
Data types of variables are the kind of data a variable holds. Python has four basic data types, among others :
-
Integer
-
Float
-
String
-
Boolean
To know the type of data a variable holds, you can use the Python type() function.
When working with different data types in Python, it sometimes becomes useful to convert one data type into another because the data type of a variable determines what operations it supports.
For example, an int data type does not directly support iteration, while the str type does. Or, when dividing coprime integers, you'd get a fractional result that does not come under the ints category. If your result variable is an int, you might lose information! Converting a data type to another is what we call data type conversion, and it is handy in such cases.
So essentially, there are two types of data type conversion :
- Implicit
- Explicit
Explicit data type conversion is where you force a Python compiler to convert a data type into another. When python itself does data type conversion, it's called Implicit Data Type Conversion.
In this article, we will delve deep into the implicit type conversion.
What is Implicit Type Conversion?
First things first, it is important to note that all data types in Python do not have the same level. They have a certain ordering in which Python handles them. Some of the data types are higher, and some are lower. Which one's which? We'll see in the example.
While performing any operations on variables that have different data types in Python, one of the variable's data types will be changed to the higher data type among the two variables, and then the operation is completed. This automatic conversion of data types done by the interpreter is called implicit type conversion. It does not have any involvement of the user.
Python will convert smaller data types to higher data types to prevent data loss. Seems complicated! Don't worry; all this jargon will make sense when we see a few examples of how it is done and what this statement means. When we do, keep these few statements in mind.
A very common example of adding an integer and float value is given below; let's find out the resultant data type.
Example - Implicit Type Conversion of int to float:
Output
In this small piece of code, we have declared two variables, num1 and num2. num1 is of the type - int, while num2 is of the type float. We have then declared a third variable, res, which stores the value of their sum. We then print out the data types of each variable, including the resultant variable, res and see that when we add an integer and float data type, our result is a float.
But Why is our Result a float?
As we read earlier, the data type conversion is done to prevent the loss of data. Had our result been converted to an int, the fractional portion of the result would have been lost. Hence, the Python online compiler converted the smaller data type (int) into a larger one (float) to avoid any data loss.
Now do you see why conversion to a "higher" data type is necessary and how it prevents loss of data?
The same way we added two numbers, we can even see the conversion of an int to float when dividing two numbers.
Output
Just like our previous example, here even though both num1 and num2 were integers, python converted our result to a float to prevent any of the fractional portion of the result to be lost.
Let us now take a look at another example to better our understanding.
Example - Adding String and Integer:
Now in the above example, we tried adding int and float, which were both numeric data types and were hence compatible. Let's take a look at what happens if we add data types, such as a text data type and a numeric data type.
Output
As you can see, we have tried adding a string (higher data type) and an int (lower data type). One might expect implicit type conversion, but this doesn't happen here as the data types are incompatible.
The solution to this problem is explicit type conversion.
Conclusion
-
There are two ways of converting data types:
- Implicit
- Explicit
-
Implicit data type conversion is done automatically by the python compiler without any involvement of the user.
-
The compiler converts smaller data types into larger data types to prevent any loss of data, for example, conversion of int to float.
-
Implicit type conversion will occur only if the data types used in the statement are compatible. If the data types are incompatible, then a type error will be raised.