Python Type Conversion
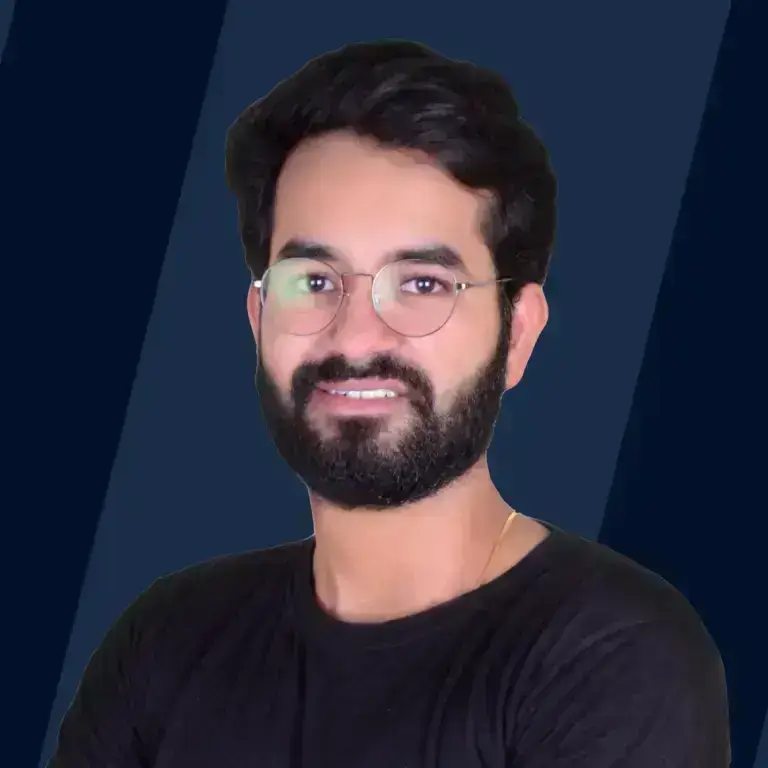
Overview
Type conversion refers to changing a given object from one data type to another data type.
Type conversion in Python is of two types:
Implicit where the conversion takes place automatically by the Python interpreter during runtime, and Explicit where the programmer manually converts the data type using some in-built Python functions.
What is Type Conversion in Python?
You would be aware that we have different data types like int, float, and string in Python.
Suppose we need to change a value from decimal to integer, to display its rounded-off data. Or from string to integer when we take user input of their age from the console and need to do an equality check with another number.
As you might have guessed, we will not be able to do operations like addition or equality checks on two values with different data types.
Type conversion in Python is used to convert values from one data type to another, either automatically by the Python interpreter or manually by the programmer using in-built functions. Note that not all data types can be converted into each other. For example, there's no way a string consisting of English letter alphabets can be converted into an integer.
The below image covers some type conversion examples between values of int, float, and string type.
Note:
An interpreter converts a high-level language into a language that can be understood by the computer. The interpreter reads each line of code one by one and then executes it directly.
Types of Type Conversion in Python
There are two types of type conversion in Python:
- Implicit Type Conversion: Done automatically by the Python interpreter.
- Explicit Type Conversion: Needs to be done manually by the programmer.
Let us learn about both these methods in detail along with some examples.
Implicit Type Conversion in Python
Implicit type conversion is when the Python interpreter converts an object from one data type to another on its own without the need for the programmer to do it manually. The smaller data type is covered into higher data type to prevent any loss of data during the runtime.
Since the conversion is automatic, we do not require to use any function explicitly in the code.
EXAMPLE:
Output:
Explanation:
We calculate the total marks and store them in a variable total. Since it is the sum of all integer values, the sum is also an integer and hence the data type of total is int. But let us see what happens in line 5. We divide an integer (total) by another integer (5), which may result in an output (percentage) having decimal points.
If the data type of variable percentage is an integer, we will lose the decimal part of the output. Hence to avoid this data loss, Python automatically converts the data type of percentage to float type, which is capable of storing decimal values.
Note:
type() method is used to return the class type of the input parameter. Hence, type(5) returns <class 'int'> whereas type("5") will return <class 'string'>.
Explicit Type Conversion in Python
Suppose we need to convert a value from a higher to a lower data type. As we saw in the previous section, implicit type conversion cannot be used here. Now explicit type conversion, also known as type castin, comes to the rescue. Explicit type conversion means that the programmer manually changes the data type of an object to another with the help of in-built Python functions like str() for converting to string type and int() for converting to the integer type.
Note:
In explicit type conversion, loss of data may occur as we enforce a given value to a lower data type. For example, converting a float value to int will round off the decimal places in the output.
SYNTAX:
We will take an example where we need to print the total marks and percentage of a student. We create a variable called message1, concatenate the message string with the total marks, and print it. Let's see if that works!
EXAMPLE: (without type casting)
Output:
TypeError:
can only concatenate str (not "int") to str
Explanation:
We cannot concatenate a string with an integer in Python since they both are of different data types. Due to this data type mismatch, the above code triggers a TypeError.
How to resolve this? Let us typecast the total marks and percentage to string data type using the in-built function str() and run the code again.
EXAMPLE: (with type casting)
Output:
Explanation:
There we go! We used the str() function to convert total of int data type and percentage of float data type to string type. Once both the message and the value are of string type, the concatenation of strings takes place and the output is printed.
Just like str() function, we have many more in-built functions in Python to explicitly convert the data type of values. Let us cover each of them in detail below:
- int(x, base): The int() function converts value x of any data type to an integer. If x is of string type, base refers to the base of the input string. For example, base of 2 means the input is a binary string.
EXAMPLE:
Output:
Note:
If no base is specified with a string input, a default base of 10 is taken. In other words, the input is treated as a decimal base string.
- float(x): The float() function converts value x of any data type to a floating point number.
EXAMPLE:
Output:
- hex(x): The hex() function converts an integer x of base 10 to a hexadecimal (base 16) string.
EXAMPLE:
Output:
- oct(x): The oct() function converts an integer x of base 10 to an octal (base 8) string.
EXAMPLE:
Output:
- complex(real, imaginary): The complex() function converts a real number to a complex number having a real and an imaginary component.
EXAMPLE:
Output:
Note:
If the imaginary part is not specified, a default value of 0 is taken.
- str(x): The str() function converts a number x to string type. The number could be integer, floating point or complex.
EXAMPLE:
Output:
- chr(x): The chr() function converts a number x to its corresponding ASCII character value.
EXAMPLE:
Output:
Note:
The value returned by chr() function is of string type.
- ord(x): The ord() function does the exact opposite of chr() function. It converts an ASCII character x to its corresponding integer type.
EXAMPLE:
Output:
- list(x): The list() function converts an iterable x to list type.
EXAMPLE:
Output:
- tuple(x): The tuple() function converts an iterable x to a tuple.
EXAMPLE:
Output:
- set(x): The set() function converts an iterable x to a set. Remember that a set contains only unique values and can be in any order.
EXAMPLE:
Output:
- dict(x): The dict() function converts a tuple x of the format (key, value) to a dictionary, where the value at the first tuple index becomes the key and the one at the second index becomes the value of the output dictionary.
EXAMPLE:
Output:
Benefits of Type Conversion in Python
- Type conversion is used to convert the data type of an object from one type to another.
- It helps us do operations on values having different data types. For example: concatenating an integer to a string.
- Implicit type conversion prevents the loss of data by taking care of data type hierarchy. For example: variable of type int is promoted to float to avoid loss of decimal places.
- It helps us convert a value to a lower data type depending on the use case. For example: explicitly convert float value to int to round off the decimal.
Conclusion
- Type conversion in Python refers to changing a given value from one data type to another.
- Implicit type conversion is done automatically by the Python interpreter during runtime.
- It converts lower data type into higher data type and thus prevents any loss of data.
- We get TypeError if we try to implicitly convert a higher data type to a lower data type.
- Explicit type conversion is done manually by the programmer to convert the higher data type to the lower data type.
- Explicit type conversion may result in loss of data.
- Explicit type conversion is also known as type casting.
- We have various predefined functions in Python for explicit type conversion like int(), float(), hex(), oct(), complex(), str(), chr(), ord(), list(), tuple(), set() and dict().
- type() method is used to return the class type of the passed input parameter.
- Type conversion benefits include operating on values with different data types, preventing data loss through data type hierarchy as well as helping in round-off operations by converting a value to a lower data type.