Explicit Type Conversion in Python
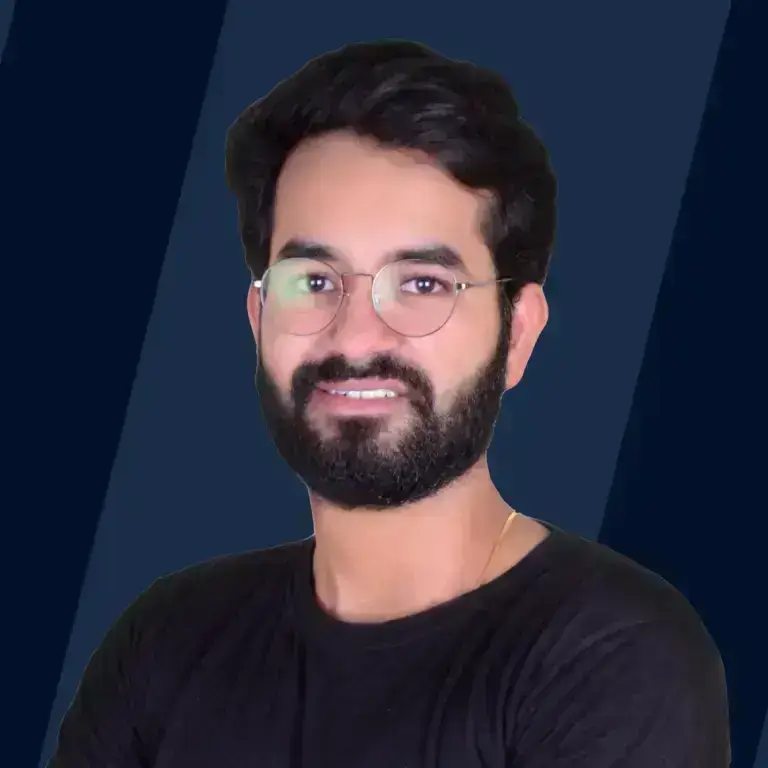
Overview
We transform an object's data type to the desired data type using explicit type conversion. To achieve explicit type conversion, we use predefined functions like int(), float(), str(), etc. Due to the user's casting (changing) of the objects' data types, this form of conversion is also known as typecasting.
What is Type Conversion in Python?
Suppose a situation where a person is traveling to America from India and that person wants to buy something from a store in America. But that person only has cash in INR(Indian Rupees). So the person goes to get their money converted from INR to USD (American Dollar) from a local bank in America.
The bank converts the INR into USD, and then the person can use the USD currency easily.
In the above scenario, there are two types of currencies, INR and USD, which you can consider as data types the bank has converted INR to USD, and this process is similar to Type Conversion in Python!
Now let’s see the definition of type conversion in Python-
Type conversion is a process by which you can convert one data type into another. Python provides type conversion functions by which you can directly do so.
There are two types of type conversion in Python:
- Implicit Type Conversion
- Explicit Type Conversion
In this article, we will learn in-depth about Explicit Type Conversion.
What is Explicit Type Conversion in Python ?
The conversion of one data type into another, done via user intervention or manually as per the requirement, is known as explicit type conversion. It can be achieved with the help of Python’s built-in type conversion functions such as int(), float(), hex(), etc.
Explicit type conversion is also known as Type Casting in Python. So now let’s see the different functions we can use to perform type casting in Python or explicit type Conversion
Functions
int()
float()
ord()
float()
hex()
oct()
tuple()
set()
list()
dict()
str()
Come along and look at these built-in functions one by one to understand them better so you can use them while programming in Python!
Int
Syntax:
This function can convert a floating-point number or string into an integer data type. It consists of two parameters. They are as follows-
- The first one “x” is the data type, which we want to convert into an integer data type. For example, if we want to write the decimal number 25 with its base, it will be written like this- , where 10 is the base for the value 25.
- The second one is base, which defines the number base. For example, a binary number has a base of 2. For an octal number, the base will be 8. The default value of the base is 10. If you want to convert the string data type into a decimal number, you need the base value to be 10.
Check out the example below to see how it’s used, Code:
Output:
From the above output, we can clearly see that the int() function has successfully converted the string into integer values with base 2 and base 10, respectively.
If you pass some other value, like the following code snippet-
You will see the following error if you execute it-
Float
Syntax:
Using float() function, you can convert an integer or string into a floating-point number. It only accepts one parameter that is the value, which needs to be converted into a floating-point.
Example:
Output:
In this code snippet, we have converted a string and an integer into a floating-point literal using the float() built-in function of Python.
Ord
Syntax:
This ord() function converts characters into their integer ASCII value. ASCII stands for American Standard Code For Information Interchange.
It is an integer value that is assigned to each character and symbol. So this ord() function helps to get the corresponding ASCII value of the given character.
Let’s understand this with an example.
Code:
Output:
Here, we have managed to find the ASCII values of the characters “Y” and “5” using the ord() function.
Hex
Syntax:
Using the hex() function, the user can convert an integer into a hexadecimal string. This function only allows integers to be changed into hexadecimal.
Take a look at the following example,
Code:
Output:
The output “0x2d” is the hexadecimal equivalent of the integer “45”.
Oct
Syntax:
Just like the hex function, this function only converts an integer into an octal string.
Check out the following example to see how its used,
Code:
Output:
The oct() function successfully converted the numerical value into an octal value having the base as 8.
Tuple
Syntax:
This function is used to convert data types into a tuple. A tuple is a collection in Python, which is used to store multiple objects. Tuples are ordered and immutable. Ordered refers to the order of the elements and how they are arranged sequentially (for example-(1,2) != (2, 1)) whereas immutable indicates that the value at a particular index cannot be changed. In simple words, these objects have a defined order which cannot be modified, and we cannot add, remove or edit the objects.
Example:
Output:
The given string was converted into a tuple using the tuple function with ease.
Set
Syntax:
The set() function is used to convert any iterable into sets. A set is a mutable collection that can only store distinct values. By mutable, it means that the set can be changed or modified.
Let's look at an example to understand this better,
Code:
Output:
The set function helps us to convert a string into a set successfully.
List
Syntax:
We use the list() function to convert an iterable into a list. A list in Python is a collection-like array that stores multiple different or same types of items.
Example:
Output:
By using the list() function, we have managed to convert the string “Scaler” into a list that contains characters as list elements.
Dict
Syntax:
Using this function, the user can convert data type into a dictionary but the data type should be in the sequence of (key,value) tuples. Some examples of key-value pairs are- ((‘1’, Rahul), (‘a’, ‘Alphabet’)).
The corresponding dictionary for the above example will be-
One thing to remember is that the key should be unique in nature; otherwise, the existing value will be replaced and overwritten.
Let’s see an example to understand this better,
Code:
Output:
Through the above code snippet, we converted the sets in pairs into a dictionary.
Str
The string function looks like this-
The str() function is used to convert any data type into the string data type. It comes in handy when we want to make use of the wide range of string functions of Python like count(), length(), upper(), lower() etc.
Check out the following example to see it in use,
Code:
Output:
More Examples
Example 1: Print the addition of string to an integer.
Code:
Output:
In the above code snippet, we have considered one string and one integer value. Then, we have type-casted the string into an int and then added the two variables.
Example 2: To show the basic data type conversions using Explicit Type Conversion.
Code:
Output:
Through the above code snippet, we have converted different values into different data types like int, float, string etc.
Conclusion
Now that we have reached the end of the article, you have learned the following points which you should keep in mind:
- Explicit Type Conversion is also known as Type Casting in Python.
- Explicit type conversion is done by the user whereas Implicit Type Conversion is done by a Python interpreter.
- We saw the different functions used to perform explicit type conversion and their examples.
You are now all set to use these functions in your Python programs. Happy Coding!