Opening and Closing Files in Python
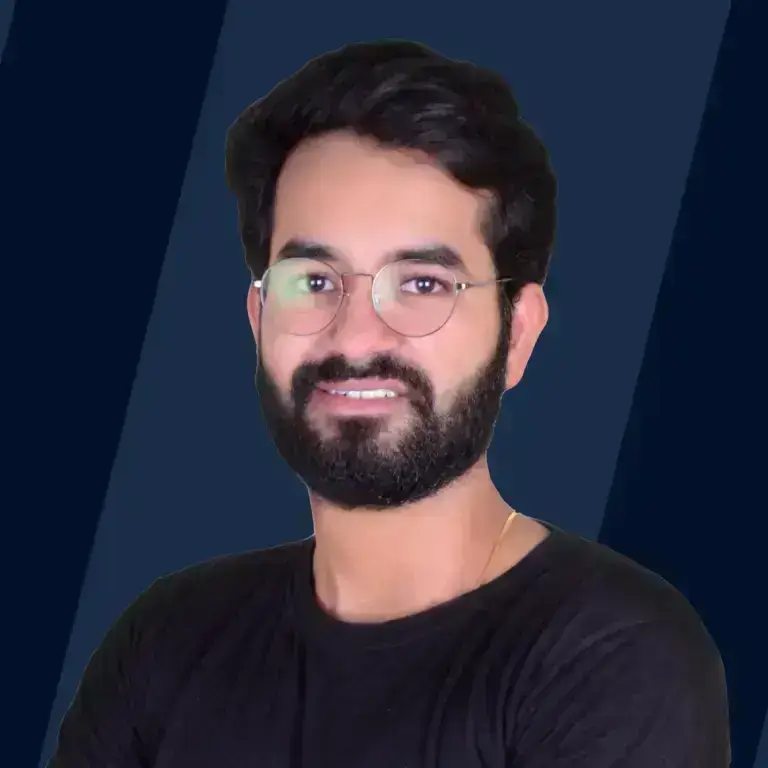
Overview
There may be times when using Python programming language one needs to interface with external files. File creation, writing, and reading functions are built into Python, along with methods to open and close those files in Python.
What is File Handling in Python?
Suppose you are working on a file saved on your personal computer. If you want to perform any operation on that file like opening it, updating it or any other operation on that, all that comes under File handling. So, File handling in computer science means working with files stored on the disk. This includes creating, deleting, opening or closing files and writing, reading or modifying data stored in those files.
What is the need for File Handling?
In computer science, we have programs for performing all sorts of tasks. A program may require to read data from the disk and store results on the disk for future usage. This is why we need file handling. For instance, if you need to analyse, process and store data from a website, then you first need to scrap(this means getting all the data shown on a web page like text and images) the data and store it on your disk and then load this data and do the analysis and then store the processed data on the disk in a file. This is done because scraping data from a website each time you need it is not desirable as it will take a lot of time.
Opening and Closing a File in Python
In file handling, we have two types of files one is text files, and other is binary files. We can open files in python using the open function
open() Function:
This function takes two arguments. First is the filename along with its complete path, and the other is access mode. This function returns a file object.
Syntax:
Important points:
- The file and python script should be in the same directory. Else, you need to provide the full path of the file.
- By default, the access mode is read mode if you don't specify any mode. All the file-opening access modes are described below.
Access mode tells the type of operations possible in the opened file. There are various modes in which we can open a file. Let's see them:
Serial No. | Modes | Description |
---|---|---|
1. | r | Opens a file in read-only mode. The pointer of the file is at the beginning of the file. This is also the default mode. |
2. | rb | Same as r mode, except this opens the file in binary mode. |
3. | r+ | Opens the file for reading and writing. The pointer is at the beginning of the file. |
4. | rb+ | Same as r+ mode, except this, opens the file in binary mode. |
5. | w | Opens the file for writing. Overwrites the existing file and if the file is not present, then creates a new one. |
6. | wb | Same as w mode, except this opens the file in binary format. |
7. | w+ | Opens the file for both reading and writing, rest is the same as w mode. |
8. | wb+ | Same as w+ except this opens the file in binary format. |
9. | a | Opens the file for appending. If the file is present, then the pointer is at the end of the file, else it creates a new file for writing. |
10. | ab | Same as a mode, except this opens the file in binary format. |
11. | a+ | Opens the file for appending and reading. The file pointer is at the end of the file if the file exists, else it creates a new file for reading and writing. |
12. | ab+ | Same as a+ mode, except this, opens the file in binary format. |
Example of Opening and Closing Files in Python
Code:
It is general practice to close an opened file as a closed file reduces the risk of being unwarrantedly updated or read. We can close files in python using the close function. Let's discuss it.
close() function:
This function doesn't take any argument, and you can directly call the close() function using the file object. It can be called multiple times, but if any operation is performed on the closed file, a "ValueError" exception is raised.
Syntax:
You can use the 'with' statement with open also, as it provides better exception handling and simplifies it by providing some cleanup tasks. Also, it will automatically close the file, and you don't have to do it manually.
Example using with statement
Code:
The method shown in the above section is not entirely safe. If some exception occurs while opening the file, then the code will exit without closing the file. A safer way is to use a try-finally block while opening files.
Code:
Now, this guarantees that the file will close even if an exception occurs while opening the file. So, you can use the ‘with’ statement method instead. Any of the two methods is good.
Now we will see examples of how to open and close files in python in various modes. Below is an example of a few important modes for rest you can try yourself.
Now we will perform some operations on the file and print the content of the file after each operation to get a better understanding of how this works. The example is given below. Both the file and the python script should be in the same folder.
Code:
Explanation:
In the above example, at first test.txt file was opened in read(r) mode to read its content, and that file data will be printed and after that file was opened with write(w) mode so that it will overwrite all the content of that file and new data will be written to that file. After that file was opened in append(a) mode, so new data will be appended to the existing data of the file and will not be overwritten.
Conclusion
- Creating, reading, opening, writing and closing files using Python comes under File Handling
- There are various methods of file handling that have been discussed above thoroughly.
- If we try to write on an already closed file, the ValueError exception is raised.