Python @property
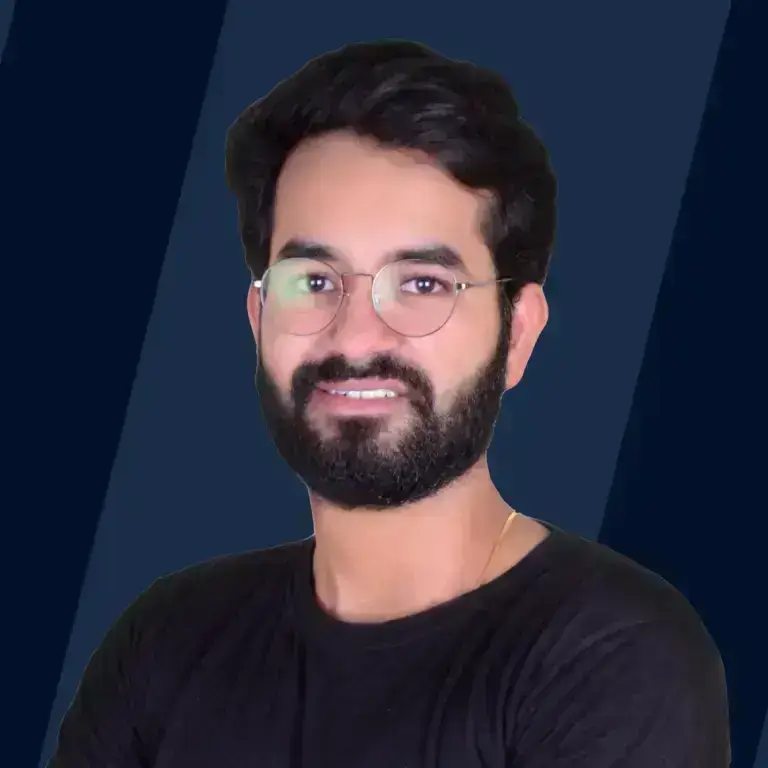
Overview
If we want to use a function like a property or an attribute, we can use @property decorator in python programming. @property in python is a decorator. The @property decorator is a built-in decorator that comes under the property() function.
We can also use the property() function to define the properties of a python class.
What is @property in Python?
To learn about @property in python, we should first know the term - decorator. Decorator is a very powerful and special kind of function in python. A decorator takes another function as its argument, and it returns yet another function with modified behaviour.
@property is also a decorator. If we want to use a function like a property or an attribute, we can use @property decorator. The @property decorator is a built-in decorator that comes under property() function. The property() function is used to define the properties of a python class.
So, the @property decorator makes our work easier, as we do not need to manually call the property() function to get the properties of the class.
We can use the @property decorator on any method of a class and use the method as a property.
The @property decorator also makes the usage of getters and setters much easier, we will be learning more about the getters and setters, later in this article.
Declare a Property
We can also declare a function as a property using the property() function, the property function itself returns the object of the property class.
The basic syntax of property() method is: property(fget, fset, fdel, doc)
- fget: fget is an optional argument that is used to get the value of an attribute. It's default value is set to None. (a basic getter)
- fset: fset is an optional argument that is used to set the value of an attribute. It's default value is set to None. (a basic setter)
- fdel: fdel is an optional argument that is used to delete the value of an attribute. It's default value is set to None. (a basic deleter)
- doc: doc stands for docstring, it contains the basic documentation for the attributes of the class. It's default value is set to None.
What is Returned by the Property?
There are mainly three methods associated with a property in python:
- getter - it is used to access the value of the attribute.
- setter - it is used to set the value of the attribute.
- deleter - it is used to delete the instance attribute.
The property() method returns the function as property class using these getters, setters, and deleters.
Let's take an example to get a better understanding of the property() method.
Output:
Property Class
Let us take the example of a Person class and we will declare a function namely person_name(). We will learn how to declare the function as a property using the property() method. The property method will convert the function into a property.
The @property decorator is recommended to use in the case of property() method.
Syntax of Using the property() Method:
Output:
If we do not declare the function as a property, we must call the function using parenthesis or () after the function name.
Example: Jack.person_name()
Otherwise, we will get the address of the person_name() function.
Using @property Decorator
Earier we learnt about property() method. Now, let us take the same example of a Person class. We will declare the same function named person_name(). But this time, we will learn how to declare the function as a property using the @property decorator in python. @property decorator in python is more practical and frequently used technique than the property() function.
Syntax of using the @property decorator:
Output:
We can also use the property class.
Why Should We Use @property Decorator?
- Using the @property decorator, you may encapsulate data by making it seem like a class's public attribute while in fact getter and setter methods are used to access and modify it. By doing so, you may manage how the data is accessed and edited as well as apply logic for validation and other purposes.
- The @property decorator enables you to access and alter data in a more Pythonic manner, offering a more Pythonic interface. For instance, you don't need to invoke a getter method to retrieve a property's value; you can just use it like any other attribute.
- By isolating the logic for data access and change from the rest of your code, you may improve the readability and maintainability of your code by using the @property decorator. By doing this, it may be simpler to comprehend how your code operates and to make modifications to it without damaging anything.
Property Setter
In the example above, we have seen the syntax and use of the @property decorator. The main problem with the @property decorator used in the Person class is that we cannot use the property to modify the attributes or values of the class. The @property decorator can be only used to access the value returned by the person_name() function.We cannot modify the value by just using the @property decorator in python.
Python has a special decorator method called setter method that helps in modifying the value of the function. The syntax of declaring the setter method decorator is: @property-name.setter.
Let's take the same Person class example, and learn how to use the setter decorator method to modify the value of a function.
Output:
Property Deleter
Just like the setter method, python has deleter method which is used to delete the property of a class. The syntax of declaring the deleter method decorator is: @property-name.deleter.
Once the property is deleted, it cannot be accessed using the same instance.
When we use the del keyword with the class name, the deleter method is invoked which will delete the property of the class.
Let's take the same Person class example, and learn how to use the deleter decorator method to delete the name property of the Person class.
Output:
As we can see in the example demonstrated above, after we have invoked the deleter method, the name attribute got deleted. So, when we tried to access the name after the deletion, an error occurred saying the 'Person' object has no attribute 'name'.
Deleter method does not set a value to None, deleter method removes the value from the class.
Class Without Getters and Setters
Let us take the example of a Student class that will store the name and roll number of students. We will make objects for the Student class and will use the Student class objects to get and set the values of the class.
But, using Objects for setting and getting the values is not recommended because of data security issues. Later in this article, we will learn about some methods called getters and setters that will help us to set and get the values of the class.
Let's see the implementation to get better understanding.
Output:
Using Getters and Setters
Previously we have learned about setting and getting the values of class using objects. Let's now learn about the two most commonly used methods namely - getter method and setter method.
The getter and setter methods help us to secure our class data. Without the getters and setters, any object can access and modify the values of a class and.
The getter and setters help us to encapsulate our data in a single object using methods so that the data cannot be modified by just using an object of the class.
Let's see the implementation of the getters and setters to get a better understanding.
Output:
Conclusion
- @property is a decorator. If we want to use a function like a property or an attribute, we can use @property decorator in python.
- The @property decorator in python is a built-in decorator that comes under property() function.
- The property() method is used to define the properties of a python class. The basic syntax of property() method is: property(fget, fset, fdel, doc)
- The @property decorator in python can be used to only access the value. We cannot modify or delete the values.
- The setter method helps in modifying the value of the function in the class. The syntax of declaring the setter method decorator is: @property-name.setter, which is invoked as a method.
- Python also has deleter method which is used to delete a property of the class. The syntax of declaring the deleter method decorator is: @property-name.deleter, which is invoked as a method.