splitlines() in Python
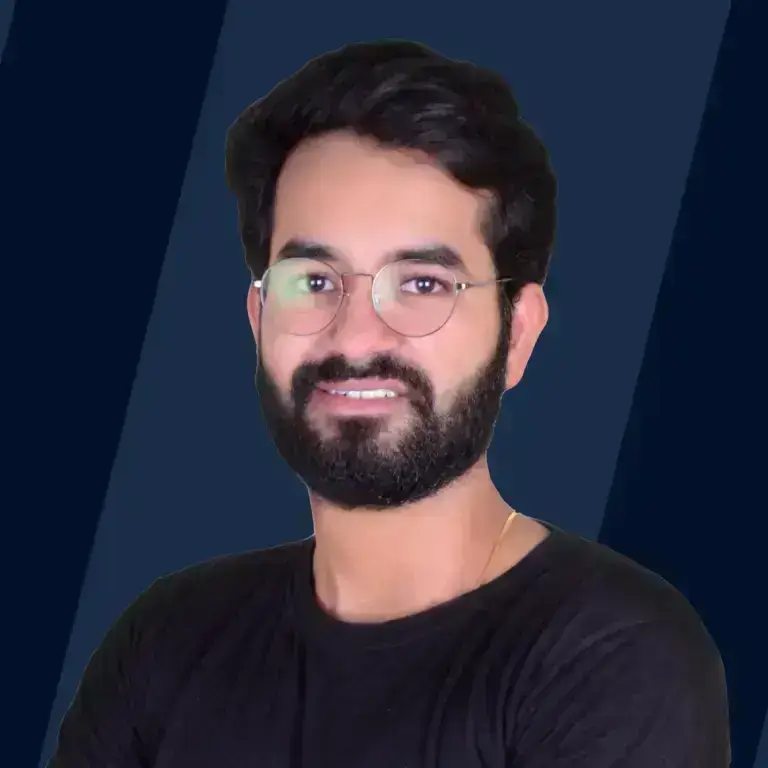
Overview
Splitlines() function in python generates a list of strings obtained by splitting the given string at specified line breaks.
Syntax of splitlines() in Python
Now, since the splitlines() function is a built-in function for the string data type in Python, its syntax is similar to other string methods, as shown below:
Here, we can observe that the splitlines() function accepts an optional parameter known as keepends. Let's understand this parameter and its use in the splitlines() method.
Parameters of splitlines() in Python
As discussed earlier, the splitlines() function accepts only one optional parameter, which is described below:
Keepends
- Type of the parameter - Boolean
- Default value - False
- Function - When the Keepends parameter is set to True, the line break statements are also included in the resulting list.
Now, let's look at the return value of the splitlines() function. :::
Return Value of splitlines() in Python
The splitlines() function returns a comma-separated list of lines extracted from a given string having line breaks. But what would happen if we give the splitlines() function an empty string or a string having no line breaks as the input?
Let's try to understand and explore this by learning how exactly the splitlines() function is working under the hood.
Example of splitlines() Function in Python
Let's look at an example to understand the functionality of the splitlines() function:
Code:
Output:
Here, we can notice that the splitlines() function returned a Python List that contains the sentences or lines present in the multi-line string input (para). :::
What is splitlines() in Python?
Consider a scenario where you are given a task to find the sentence having the largest number of words from a given paragraph. For accomplishing this task, the first and foremost step is to extract all the sentences from the paragraph, i.e., we need a function to split the paragraph or multi-line string into a collection of sentences.
For this task, Python provides a special built-in function known as splitlines() that can split the multi-line string into a list of sentences, i.e., it returns a list of splitted lines.
To understand the working of the splitlines() function in Python, we need to understand the concept of line boundaries.
Line Boundaries are special statements that format text, perform line wrapping, or indicate potential line breaks. In python, these lines boundaries include:
Representation | Description |
---|---|
\n | Line Feed |
\r | Carriage Return |
\r\n | Carriage Return + Line Feed |
\v or \x0b | Line Tabulation |
\f or \x0c | Form Feed |
\x1c | File Separator |
\x1d | Group Separator |
\x1e | Record Separator |
\x85 | Next Line (C1 Control Code) |
\u2028 | Line Separator |
\u2029 | Paragraph Separator |
The splitlines() function uses these line boundaries to locate the breakpoints and then splits the string into different parts that are stored into a Python List.
More Examples
Let's look at some examples to understand how we can use the splitlines() function.
Example 1 : Use of Keepends Parameter
In this example, we will notice the effect on the result obtained from splitlines() function when the Keepends parameter is set to True.
Code:
Output:
Here, we can observe that the newline character (\n) is also present in the result of the splitlines() function.
Example 2 : Empty String Input
In this example, we will notice the effect on the result obtained from the splitlines() function when we provide an empty string or a string with no line breaks as the input.
Code:
Output:
Here, we can observe that if the input string is empty, the splitlines() function returns an empty list. Whereas, If there are no line-break characters (or line boundaries) present in the string, it returns a single-line list, i.e., it returns a list having the input string as its only element.
Example 3: String Input With Different Line Breaks
In this example, let's understand the splitting process when the input string contains different line breaks.
Code:
Output:
Here, we can observe that the splitlines() function splits the string on each specified line break.
Example 4 : Practical Application of Splitlines Function
Now, let's look at the practical application of the splitlines() function that we already discussed at the very beginning of this article, i.e., finding the sentence having the maximum number of words.
Code:
Output:
In the above example, firstly, we extract the sentences from the input para using the splitlines() function. We further split the sentences using another built-in string function called split(). The split() function returns a list of words by splitting the string at whitespace characters. Then we can calculate the number of words in the string by simply using the len() function.
Here, we can observe that sentence four has the largest number of words (equals 10), which was the expected result.
Conclusion
- Splitlines() is a built-in function for strings in Python.
- It generates a list of lines from a given string.
- It splits a string according to the occurrence of different Line Boundaries.
- In the case of an empty string, it returns an empty list.
- In the absence of line breaks, it returns a unit-length list.
- It can be used to extract sentences from a paragraph.
See Also
Some similar built-in Python functions are: