C++ Program to Find the Length of a String
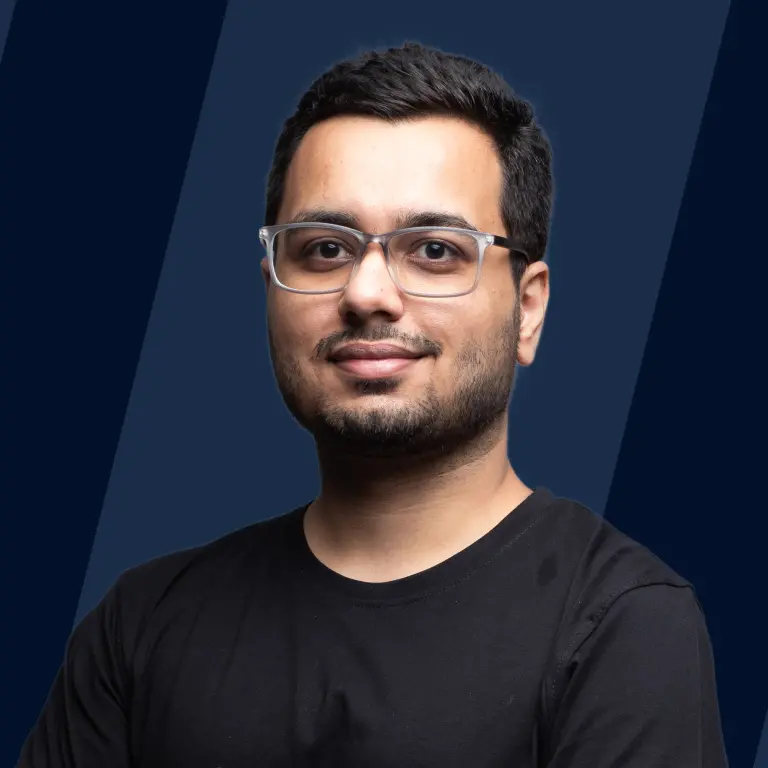
Overview
String length in C++ can be calculated using 5 different methods. We can make use of the string::size, string::length ( here, :: is called a Scope Resolution Operator. It indicates to which namespace or class a symbol belongs), strlen() method (C library function), for loop or while loop.
Introduction
Strings are a group of characters enclosed between double quotes (""). Strings can be represented as an array of characters with a null character at the end. Example, HELLO is represented as
Arrays are zero-indexed. This means we start counting from 0; therefore, the first character, i.e., H, has the index of 0. Further, the null character, i.e., \0, will not be included in the length of the string. With these pre-requests, let us see how to find string length in c++.
We can find the string length in c++ using the following methods :
- Using std::string methods
- string::length method
- string::size method
- Using the C library function
- strlen() function
- Using loops
- for loop
- while loop
Important points
- The string class constructor will set it to a C-style string, which ends with a null character(\0).
- The size() and the length() function both return the same value i.e, both size() and length() functions are synonyms. Different names are given so that they can be used consistently. The size() is consistently used with STL containers (Standard library templates) like map, vector, etc., and length is consistently used with character strings, words, sentences, paragraphs, etc.
Methods to find the length of the string
Let us look at different methods to find the length of the string, along with an example.
Using string::size
The length of the string can be calculated using the length() function. The length() function returns the number of characters in the given string.
The syntax is simple. We have to add .length() as a suffix to the name of the variable containing the string whose length has to be calculated.
Syntax:
Here is a code to find string length c++ using .size() :
Code:
Output:
Using string::length
The size() function returns the same value as the length() function. As discussed earlier, both are synonyms.
Syntax:
Here is a code to find string length c++ using .length() : Code:
Output:
Using C library function strlen() method
The C library function strlen() returns the number of characters in the string, which is passed as a parameter.
Syntax:
Here is a code to find string length c++ using strlen() :
Code:
Output:
Using for loop
For Loops can be used to find the length of the string. An iterating variable that will traverse along the length of the string. The variable of the counter variable is incremented by one in each iteration. The loop breaks once the iterating variable reaches the null character.
Here is a code to find string length c++ using the for loop :
Code:
Output:
Using while loop
This method is similar to finding the string length using the for loop, but the only change is that the while loop is used instead of for loop. Click here, to learn more about While Loop in C++.
Here is a code to find string length c++ using the while loop :
Code:
Output:
Stand out in the coding world with a C++ certification. Join Scaler Topics Free course and unlock the door to becoming a proficient software developer.
Conclusion
- The string length in C++ can be found out using the length() and size() method.
- strlen(), a C library function, can also be used to find the length of the string.
- for loop and while loop can also be used to find the length of the string by iterating through the string until a null character is encountered.