Switch Case in Python
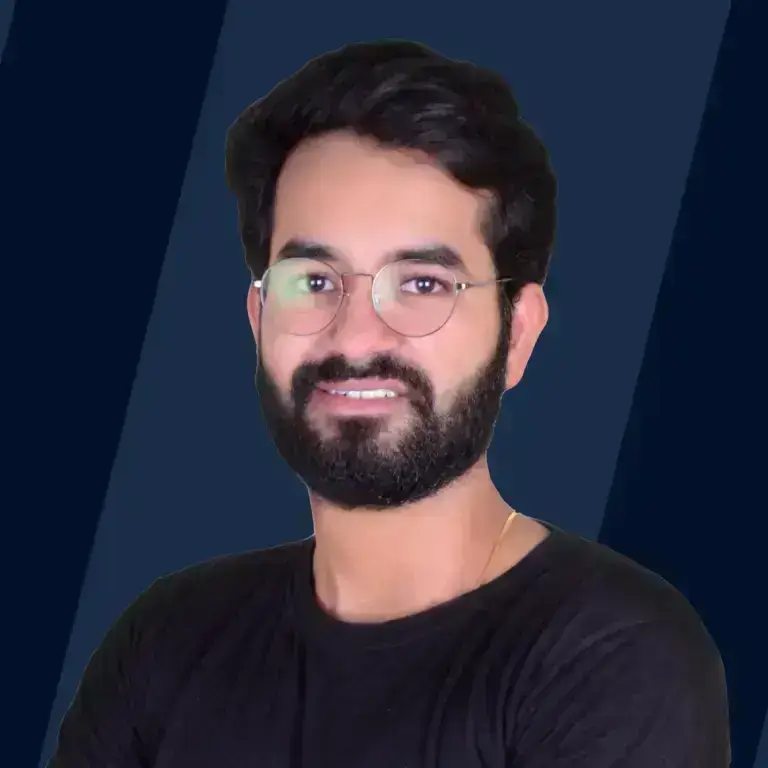
Overview
Python Switch Case is a selection control statement. The switch expression is evaluated once. The value of the expression is compared with the values of each case; if there is a match, the associated block of code is executed. Unlike other languages, Python doesn't have built-in switch case statements, so we have to implement them using different methods. All of these methods are explained in the article. Read along to know more.
Introduction to Python Switch Case
Have you ever wondered how a menu-driven program (A program that obtains input from a user by displaying a list of options) works? As these programs take input from the users, they have to check the condition matches the input. For this purpose, we have to use Python Switch Case statements. Read along to know more.
What is Switch Case in Python?
The if makes a decision based on whether the condition is true or not. If the condition is true, it evaluates the indented expression; however, if the condition is false, the indented expression under else will be evaluated. When we need to run several conditions, you can place as many elif conditions as necessary between the if condition and the else condition. Switch case statements are a substitute for long if statements that compare a variable to several integral values
- The switch statement is a multiway branch statement. It provides an easy way to dispatch execution to different parts of code based on the value of the expression.
- Switch is a control statement that allows a value to change control of execution.
But unline different languages; python doesn't have its inbuilt switch case statements. Read along to learn more about how we can use switch case statements in python.
This flow chart clearly shows the working of the switch case. This shows that, first of all, the conditions are checked one by one, and after that, if not a single statement gets true, then a default statement will get implemented.
How to implement Python Switch Case?
Unlike C++/Java, python doesn't have its inbuilt Switch Case statement. There are several alternative methods by which we can implement Switch Case statements in python. These methods are:
1. With the help of dictionaries
- By using functions
- By using Lambdas
2. With the help of classes
3. With the help of if-elif-else statements
Let's see each of the methods to implement the python switch case statement one by one with the help of examples. Read along to know more.
Python Switch Case using Dictionary
If you are familiar with Dictionary and its pattern of storing a group of objects in memory using key-value pairs. So, when we use the Dictionary to replace the Switch case statement, the key value of the dictionary data type works as cases in a switch statement. Moreover, there are two different ways to implement Switch Case using Dictionary. These are:
Switch Case in Python using Functions
When we use the Dictionary to replace the Switch case statement, the key of the dictionary data type works as cases in a switch statement. We need to define some functions which are considered as values in dictionaries. When we call these values with the help of keys, then the particular function gets invoked, and we get the desired output.
We will implement a switch case in python by dictionaries with the help of functions.
Example
In this example, we are converting a number to its number name.
Code:
Output:
Explanation
- We create different functions which return the number names of the number.
- Then, we create a dictionary in which we have stored different key-value pairs.
- After creating Dictionary, we created the main function, which takes user input to find the number name.
- At the end, we have called the function to print the desired output.
Python Switch Case Using Lambdas
We are going to implement a switch case in python by dictionaries with the help of lambda functions. Lambda functions are small anonymous functions that can take n numbers of parameters but only have a single expression.
When we use the Dictionary to replace the Switch case statement, the key of the dictionary data type works as cases in a switch statement. We need to define anonymous lambda functions, which are considered values in dictionaries. When we call these values with the help of keys, the particular function gets invoked, and we get the desired output.
Let's understand the basics of lambda functions.
Syntax of Lambda Function
We have to use a lambda keyword to create the lambda function; then, we have to pass parameters and expressions to it with : separating them.
Code:
Output:
Explanation
- We have created a lambda function that accepts one parameter and returns a single expression, which returns the number by multiplying it by 10.
- Then, we print the variable by passing a number to it.
- In the end, we got the desired output.
Let's move to the example to understand how we can use dictionaries with the help of lambda functions for implementing switch-case statements in python.
Example
In this example, we are converting a number to its number name.
Code:
Output:
Explanation
- We create different functions which return the number names of the number.
- Then, we created the main function in which we created a dictionary in which we stored different key-value pairs.
- Inside the Dictionary, we have used the lambda function.
- This main function returns the number name of the number if it is available in Dictionary. If not available, then it will return the default value.
- At the end, we have called the function to print the desired output.
Switch Case using Python Classes
We can use Python Classes to implement Switch Case in python. Class in python is the combination of properties and methods. Let's see, with the help of the example, how we can implement switch cases using classes.
We have to use the getattr function, which is used for implementing switch cases by using classes. Let's understand in brief about this function.
Syntax
This is the syntax of getattr, which accepts three parameters object, name, and default. All three parameters are explained below. Read along to know more.
Parameters
The getattr accepts three parameters used for implementing conditional statements (switch case) using classes. These parameters are:
- object(MANDATORY PARAMETER): object name whose attribute is to be returned.
- name(MANDATORY PARAMETER): string that contains the attribute name of the object.
- default(OPTIONAL PARAMETER): if the attribute is not present in the object, then the default statement is returned.
Return Value
This function returns the name of the attributes present in the object, which finally gives us the output. If the attribute is not present, we will get a default value.
Example
We are going to create an example in which we are checking whether the entered digit is binary or not. If it is binary_0, our function will return zero as an output. On the other hand, if it is binary_1, our function will return one as an output, but if the digit entered is other than 0 & 1, then our code will return not a binary digit. Let's code this example for better understanding.
Code:
Output:
Explanation
- Firstly, we have created a class that will return to us whether the number input is binary or not.
- Then, we create various functions inside the class to check whether a number is binary or not.
- We have used the method getattr(), which is used to check the conditions.
- After that, we called the class and used its function to implement the switch case in python.
Don't just learn Python; master it! Enroll in our Python course and gain the expertise to tackle any coding challenge.
Python Switch Case using if-elif-else
The if…elif…else statement is used in Python for decision-making, and it can be replaced with a switch case in python with all the switch conditions in the if & elif sections and the default condition in the else section.
The if makes a decision based on whether the condition is true or not. If the condition is true, it evaluates the indented expression; however, if the condition is false, the indented expression under else will be evaluated. When we need to run several conditions, you can place as many elif conditions as necessary between the if condition and the else condition.
Syntax
This is the if-elif-else chain where we have to pass the condition into the if & elif, and else is the default statement that gets executed if no conditions above it are false.
Example
Suppose you have a number stored in the variable, and you have to check whether a number is negative, in between 1-10, or greater than 10. Let's code this problem.
Code:
Output:
Explanation
- Firstly, we assigned a number to a variable.
- Then, we use conditional statements to the if and elif blocks
- After assigning the conditions to if-elif, we return the statement from these blocks/.
- At the end, we use the else statement, which is used when no other conditions above it are not satisfied.
Application of Switch Case in Python
As we saw in the introduction section that MENU DRIVEN PROGRAMS requires a switch case to get implemented. We will write code here to create a menu-driven program with the help method if-elif-else statements. Let's code it for better understanding.
Code:
Output:
Conclusion
- First of all, we understand what a switch case is in python.
- Then we know that python doesn't have its built-in switch case statements.
- After that, we learn three different ways to implement switch cases in python.
- Last but not least, we have implemented a practical application with the help of a switch case.