translate() in Python
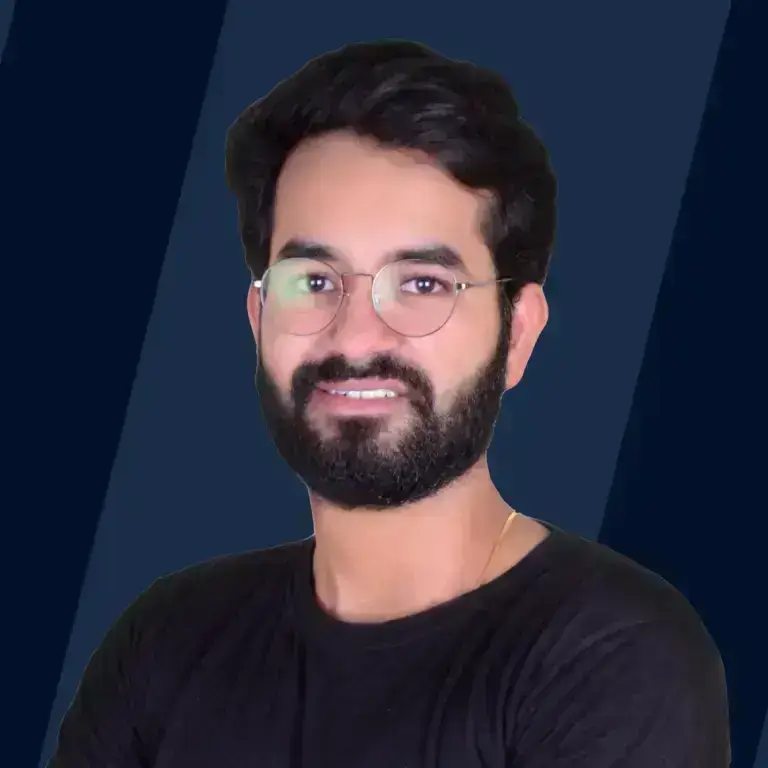
Overview
The translate() function in Python is used to replace the characters of a string according to the character mapping provided in the translation table input.
Syntax for translate() in Python
The syntax of translate() function in Python is:
Where str is the input string and the translation_table is the required parameter for the function.
Parameters for translate() in Python
The translate() function acccepts only one parameter which is described below:
Translation_table
- This parameter can accept a Python Dictionary or a Mapping Table object usually generated using the in-built str.maketrans() function.
- This parameter represents the Translation Table, i.e., a dictionary-like object that specifies the set of characters that are needed to be replaced or deleted from a string.
- Since the characters are represented using Unicode Standard in Python, the translation table takes the Unicode code points (i.e., the Unicode for the specified character) as the keys for the Map object. It should substitute them with other Unicode code points, strings, or None values.
:::
Return Values for translate() in Python
Return Type : str Description: The translate() function returns a new string generated from the input string by replacing or deleting some specified characters from the input string according to the Unicode mapping provided in the translation_table parameter.
Example for translate() in Python
Output:
Note:
- Since the translate() function accepts only Unicode mappings as the translation_table parameter, we used the ord() function to access the Unicode code for the specified characters.
What is translate() in Python?
Python provides several in-built functions to perform certain standard string operations efficiently. One such function is the translate() function, which replaces several characters in a given string with a different set of characters. This process is also known as string translation.
The translate() function uses a Unicode mapping object describing the substitutions for the specified characters. This Unicode mapping object is passed in the translation_table parameter in the translate() function.
You can notice the use of Unicode mapping in the earlier discussed example where the table variable consists of a Python Dictionary object representing the Unicode mapping. The translate() function used this mapping to convert the input string "This is a normal message" to "Th40 40 6 no2m6l m1006g1" by replacing the characters 'a', 't', 's', 'i', 'e', and 'r' to '6', '9', '0', '4', '1', and '2' respectively.
How to use translate() Function in Python?
The string translation is a process of replacing the characters present in the target string with some other characters. Since the mapping table or the translation table specifies the substitutions that will take place in the target string, the string translation process depends on the translation table object or the Unicode Mapping of characters.
Hence, based on the type of mapping table object, there are mainly two ways in which we can use the translate() function in Python:
- Manual Translation - In this method, we provide a simple Python Dictionary object as an argument to the translate function. This Dictionary object describes the Unicode Mapping of characters for the string translation process.
- Helper Function Translation - In this method, we will use the in-built string function known as maketrans() to generate a translation table provided as an argument to the translate() function. The maketrans() function is a static method. It takes at most three arguments (two optional arguments) and generates a one-to-one mapping of a character to its translation.
More Examples
Example 1: Manual Translation
Output:
Explanation: Here, we use a Python Dictionary to replace the specified characters with other characters or the None value.
Now, we know that the mapping table object defines the replacement for the specified characters in the target string. Hence, when we map a specific character present in the target string with None, it gets replaced with a None value, i.e., deleted from the target string.
Example 2 : Maketrans() using Single Argument
Output:
Explanation: When using a single argument in the maketrans() function, the argument must be a Python Dictionary object containing a Unicode mapping of characters. The maketrans() function uses this Python Dictionary object of Unicode mapping to generate a translation table for the translate() function.
Example 3 : Maketrans() using Two Arguments
Output:
Explanation: When two arguments are passed while using the maketrans() function, the arguments must be two strings of the same length as each character in the first string argument is mapped with the character present in the corresponding index of the second string. Hence, in the above example, 'a' is mapped with 'D', 'b' with 'E', and 'c' with 'F'.
Example 4 : Maketrans() using Three Arguments
Output:
Explanation: When all the three string arguments are passed while using the maketrans() function, then all the characters of the third parameter are mapped to None, i.e., they are deleted from the target string, while the characters of the second parameter replace the characters of the first parameter.
Conclusion
- The translate() method of the str class is used to replace a set of characters in the given string according to a Unicode Mapping object.
- It returns a new string after performing string translation.
- String translation can be performed using Manual translation or by using the helper function maketrans().
- The python dictionary acts as the translation table in the manual translation approach.
- maketrans() function is used to generate translation table for the translate() function.