Convert String to Datetime in Python
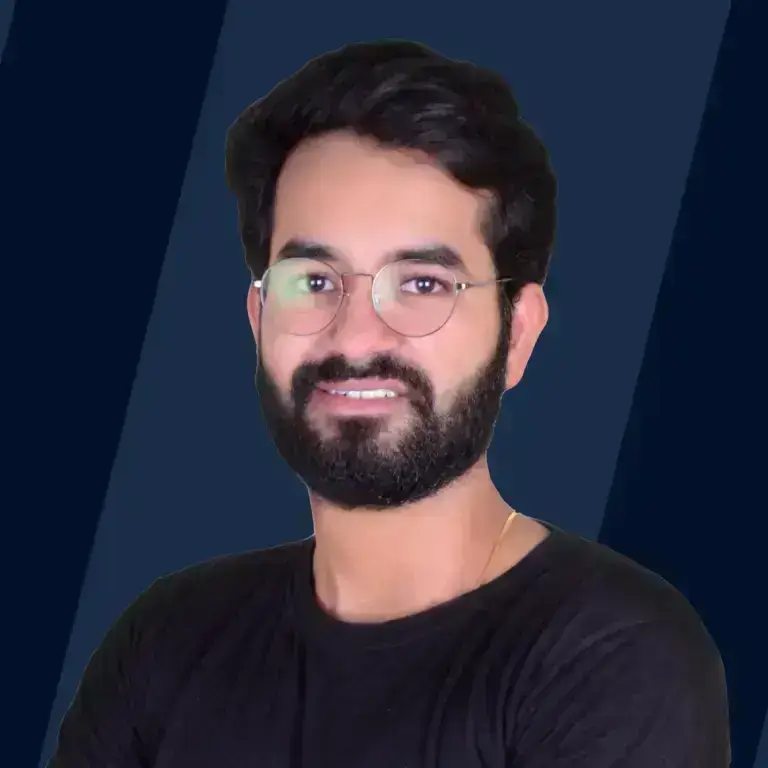
While exploring various sections in python we might have come across situations where we need to represent the date which is given in the string into a date object or vice versa (converting the date, time and datetime objects to its equivalent string).
Python has got you covered with its strptime() and strftime() functions, which can help you with exactly the same. Also, in order to switch from the standard date-time format to the string type, we have the strftime() function in python.
Converting a String to a datetime object using datetime.strptime()
In order to write a Python program where our focus is to convert a given string to datetime or vice-versa, we have in Python what we call the strptime() or the strftime() function.
One point to note here is, that when we convert the string to a datetime object, we need to have the string in a certain format. The string cannot be converted into the datetime object randomly.
The strptime() function in Python is available in the datetime and the time modules. It is mainly used for the Date-Time Conversion. The major function of this method is to change the given string of datetime into the desired format.
In Python, we have the strptime() method, which is mostly in its datetime class, and in order to convert any string representation for the date/time into a date object, we use it.
Syntax
The syntax to convert string to datetime python is done by the strptime() function as follows:
Here, the arguments that are, date_string and format must be of string type. The format can be represented as %d, %B, %Y representing the day of the month, the month's name in full format and the Year in four digits respectively.
For example:
We have the following strings representing the date-time and we want the below-mentioned output.
Input_1 :
Output_1 :
Input_2 :
Output_2 :
As shown above, we are converting the date, time, and datetime objects into string format which we can achieve via the strptime() function.
Convert string to datetime in Python Using Datetime Module
Code:
Output:
Explanation:
Here as the string had a correct format for the date the string was converted into datetime format and we got the output. It must be noted that the strptime() method does not work when the string argument is not consistent with the format parameter. Once we checked that the string was in the format we continued by applying the strptime() function to change it into date_time_obj.
Convert string to datetime in Python Using Dateutil Module
Code:
Output:
Explanation:
Here we are converting the datetime object into a string by using various formats. Through each line of code, that is we are using the dateutil module from Python to import the parse function.
Once done we move to write the strings of date in different formats as shown above. Then we make use of the for loop in Python to call the date, time, and time zone information for the specified date. This way we can convert the strings of data stored in an array and convert it to the datetime as and when required.
Convert string to datetime in Python Using strftime() Function in Python
Code:
Output:
Explanation:
Here we are converting the datetime object into a string by using various formats. Through each line of code, that is how we fetch date.today() method in the now variable representing today's day. Then move to extract the day from the new object with the help of using the %d string directive. Similarly, we fetched the month from the new date object with the help of the %m string directive, and with the help of %Y string directive we get the year of the date object.
Convert the datetime format into the string format with the strftime() function
We can also convert the datetime format into the string format with the strftime() function
Syntax:
where the format_str is usually represented as %m, %d, %Y, %H, %M,%S that is the month, day of the month, Year in four digits, the hour of the day, the minutes of the day and the seconds of the day respectively.
The above strftime() function that is available in the time module allows converting a tuple or a struct_time object into a string as defined by the format argument shown above.
For example:
We have the following datetime representing the date-time and we want the below-mentioned string type output.
Input_3 :
Output_3 :
Input_4 :
Output_4 :
For changing the string type format into date time, we have the strftime() function in python which can do exactly the same for us. The strftime() function in python is available in the datetime and time modules in order to create a string representation based on the decided format string.
Conclusion
- In python, in order to convert the string to datetime format or datetime to string format we have the pre-defined functions to help us achieve the same.
- The strptime() function in python is available in the datetime and the time modules. It is majorly used for the Date-Time Conversion.
- The strftime() function in python is available in the datetime and time modules in order to create a string representation based on the decided format string.
- To convert the date, time, and datetime object into the string using strftime() function
- To convert the string into a datetime object using strptime() function