Convert List to String in Python
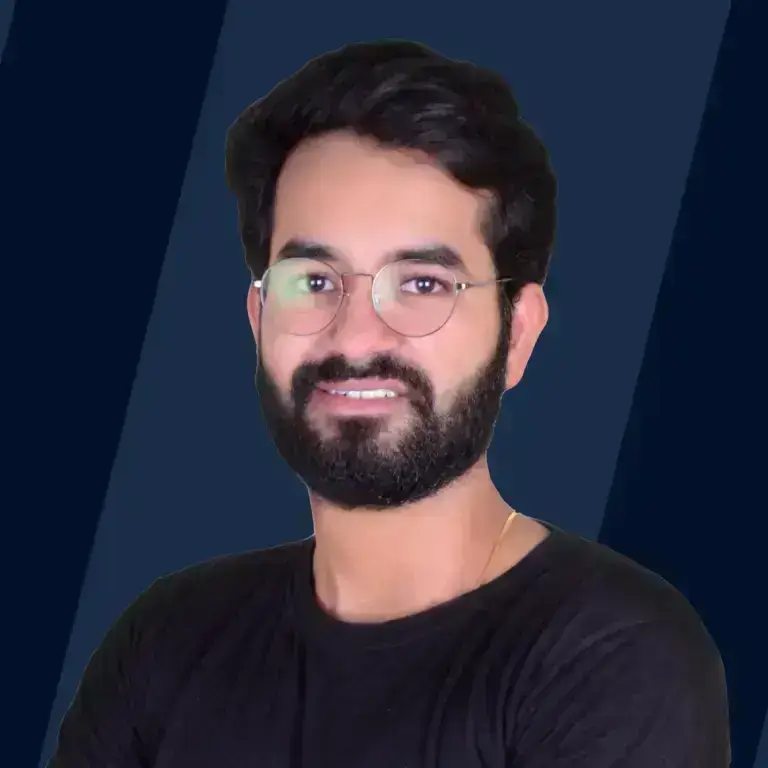
Overview
To convert a list to string in Python Programming, you can make use of the join() function, but with a separator, or by traversing the list, the map() function, or with one of the fastest operations in Python - list comprehension.
Introduction to Python List to String
Have you worked with URLs? The ones you see on the top of your browser (links, as one might call them)? They are made of different sections, like the scheme, the domain etc.
Now suppose you want to make some very minor changes to that URL. You know for a fact that the URL is a string, how will you access the exact elements you want to change? Keeping track of it's indices, or the words would be so cumbersome!
What's easier, is to break it into it's sections and then put them in a list. Now you can freely modify the complete URL anyway you want. You figured out how to break the URL into a list, or maybe while you were working with the URLs, you received the input as a list. But now how will you convert it back to the string format it was in earlier?!
It is very common in python, that you would want to convert lists to strings or vice versa. You might want to display the contents of the list, or you might want to perform string manipulations on elements of the list. Or maybe like in the scenario above you have some components of a URL fetched, but in the form of a list. And now you want the URL to of course, be a string, you would have to join all the elements of the list to make it a string. Either way, it would be convenient for you to convert your list into a string.
Before we go deep into discussing the numerous ways that we could do so, let's learn about lists and strings first.
What is a String in Python?
A string by definition is an immutable data type which can't be changed once declared in a program (explained below). Any set of characters enclosed in single or double quotes denote a string.
For example,
Now every element of the string is indexed. Let's take an example string, simply "word". It is enclosed in double quotes, so we know that it is a string. The first element of this string will have index = 0, i.e. index of w is 0. Index of the second element will be 1, and so on. It looks like this:
0 | 1 | 2 | 3 |
---|---|---|---|
w | o | r | d |
To initialize a string, you must enclose the characters in either single, or double quotes.
Have you wondered how you would access say the 3rd element of the string? Suppose you wanted to change it, you would have to access it first, and then change it! So, to access any element of the string, you first write the variable name, and with it, you enclose the index you want to fetch in square brackets.
In the example below, we're going to declare two strings in single or double quotations, and then access an element from each of the strings.
Output:
So in line 1, we simply declared a string by enclosing it in double quotations. In line 2 we then declared another string, this time in single quotations. You can see that it makes absolutely no difference when we print it!
As we read about the indexing above, when we try to access the element at index 2, in the first string, we get the output as r, and that's the exact element at index 2. The same way, in the second string, we access the element at index 4 and the output is e.
Remember when we said that strings are immutable?
Try and run the following code:
What was the output that you got? You got a type error. Strings in python are immutable, i.e. they cannot be updated like this. However, you can use string methods such as replace() if you wish to update the string.
What is a List in Python?
You must have heard of the term arrays, in other programming languages like C, Java, C++. They are collections of a data type stored in contiguous parts of memory, as in continuous memory.
Lists in Python are the same as the definition of arrays above, except, python lists, unlike arrays, need not be homogenous (homogenous means of same data type). Lists can contain variables of different data types and even of same data types. They could be strings, integers, float, objects or even other lists or a mix of all these as shown in the examples below.
Python lists are mutable, which means that even after they have been created, they can be altered. They are used to store multiple data at the same time.
To create an empty list in python, here's the syntax you can follow:
Here's what a list looks like in python:
A list in python can be created by simply separating the elements by commas, and putting them in square brackets.
In the above examples, the_list is a list that contains only strings while in the second list, another list we have strings as well as a number, which shows that lists in python can be homogenous as well as heterogenous i.e. containing values of different data types. Take a look at list 3, third_list where we have the first element which is a string, the second is a float, the third is an integer, and the last 4th element is another list.
Now, every element in this list is indexed. For example in the first list, the_list the first element of the list has index 0, the second element has index 1 and so on. The last element has index size - 1, where size is the size of the list, in our example - 4.
0 | 1 | 2 | 3 |
---|---|---|---|
This | is | a | list |
So, to access any element from this list, we use the indices of the elements. Here's how we do it, we put the name of the list, and then in square brackets, the index that we want to get.
For example, let's say we want to access the element at index 1 in the_list, then we do it this way:
Output:
Check out this article to learn more about What is list in Python?
How to Convert a List to String in Python?
Now that we know our basics, let's learn about the different ways that you could convert a list to a string in python. We will discuss 4 methods here - join, loop, map, list comprehension.
Python List to String Conversion Using Join Function
What is the join() function?
It is a function defined in the python standard library that does exactly what it's name suggests, it joins elements and returns the combined string.
Syntax of the join() function in python:
So you essentially need a string, and an iterable. An iterable is a data structure over which you can iterate / loop over. For example, a string itself is an iterable, and so is a list, but an integer is not an iterable. So you cannot use the join function to join a string and an integer directly.
How does the join function work in our case?
Say you have two variables, holding your first name and last name respectively. Now of course you would not want your name to be printed out separately, you'd like to join the two variable contents and then print it. So as per syntax, the string here will be first_name and the iterable on which we want to join would be the last_name.
Output:
Wait, what? That's not what we expected! Well, the join() function joins a separator to a sequence of words. As in, the syntax requires a string, and that string is a supposed to be a separator. The join function will join the two(or more) strings but will leave the separator character between each of the strings.
In the example above, when we wrote first_name.join(last_name) it was the same as Bugs.join("Bunny"). So, the join function considered the first string, i.e. "Bugs" to be the separator. And since only one string was provided in the parameters of the join() function, every element / character of the string "Bunny" was separated with the word "Bugs" in the middle.
So the correct way of joining the first and last names is to first create a sequence of the words, and then join it with a space separator i.e. the separator is still a string, but it's a string with simply a single space. So when the join function will join the two strings, it will place the string that has a space, between all the words it has to join / the iterables.
So here, we're creating a sequence of the words. The words simply put with commas in the join() function are not iterables, so we first need to create a structure that can be iterated over so that the join() function can work correctly.
Output:
The sequence that we created here, can be iterated over, and along with the separator, the join() function has joined the first name and last name properly.
Hence, we can use the join function to convert our list elements to a string. We're going to create a function for the same, which will take a list of strings as input and give a string as output. The separator given in the example below is again a space character enclosed in double quotes.
Output:
The time complexity of using the join() function is O(n) where n is size of the list as the join function will iterate over every element given in it's input as we read above.
Python List to String Conversion Using Traversal of a List Function
We have seen how to convert a list to a string using the join function, let's now traverse over the list. Traversal of the list means that we would be going over each and every element of the list one by one.
Here's the algorithm, we will declare an empty string, and as we go over (traverse) every element in the list, we will keep adding that to our existing string. The + here is used for concatenating the string. Let's look at the code.
Output:
If we want spaces, or separators between the elements of the list, we can simply add those as well along with every element to the string.
The extra line string += " " adds the spaces between each element of the list.
Again, the time complexity for this method is linear as well since we are traversing over every element in the list.
Python List to String conversion Using map() Function
To convert our list into a string, we can even make use of the map() function. How does a map() function in python work?
The map() function in python loops over every element in an input iterable and performs an input function on those elements. The input function could be anything, like a function you wrote, or like using str, to convert the input to a string (as we will use below). An iterable is an object that can be iterated over / looped over, eg. lists. So, the map() function in python takes 2 parameters, the function to be applied to on the elements, and second, the iterable over which we would want the function to be applied.
Syntax:
The key thing to note is that the first argument of the map() function is a function object which means that the function will be passed without being called. You need to write the function, without parantheses.
So, what do you think will be the transformation function that we will call over our list? Of course, we will call str.
Here's how it's done. We're going to create a function that converts our list to string in python and of course it's input would be a list. Next, we're simply going to return our string which has been converted from a list using the map() function. The function that will be given to map() as it's first parameter will be str, and the iterable will of course be our list.
Output:
We just received a map object (it's an iterator)! How do we convert this to string? We just read about the join function above, can that be used?
Output:
Did you see that we also need to make use of the join function so that we could convert the map object to a string? Why should we use map, if the join function can already make conversion from list to string possible?
Take a look at this with some code examples.
Output:
Did you see how print statement 1 gave an error? That is because the list was not homogenous, i.e. did not contain all elements of the same data type. We had two data types in the list, str and int. The join function treated every element in the list as str and when it encountered an int, it could not join it to the rest of the string and hence gave an error.
Now in the second print statement, where we used the map function, we fist transformed every element of the list into str using the map function, and then applied the join function on the strings. This way, we can even convert all non str elements of the list to string in Python.
PS. don't be shocked when you see the 4 in the string. Yes it is an integer, but as we read above, anything enclosed in double or single quotations in python is a string, so even "4" is a string, but 4 isn't since it is not enclosed in quotes.
Python List to String Conversion Using List Comprehension
What is List Comprehension? List comprehension is one of the most distinctive aspects of the python programming language. One single line of code can be used to construct powerful functionality. List comprehensions can be used for the creation of new lists, from other iterable objects in python like tuples, strings, lists, etc.
To write a list comprehension, we write our expression which runs on every element of the iterable using a for loop in square brackets.
Syntax:
So, what we want to is essentially convert every element in the list to string, and join them all together.
Here's how we will do it, the function that we need to apply on every element of the list is a string, so in the do_something above, we replace it with str. Next, once we have converted every element in the list from it's data type to a string, we simply need to make use of the join() function yet again to convert the list to a string using a space separator.
Output:
Every element in the list, was converted to a string first, using the str() function, even the integer 4. Since we are traversing the list, so we start from the first element in the list "Method", which is already a string, but the str function was still applied to it. Next, we move to element at index 1 which is 4 and convert this integer using the str function again and so on till the end of the list. At the end, the join() function joined the elements of the list using a space separator and we have our output.
The advantage of list comprehension in python is that it is much more time and space efficient than loops. It also required less lines of code, and even transforms iterative statements into formulae.
Check out this article to know more about List Comprehension in Python .
Conversion of List of Characters to a String
This time we are given a list of characters, not strings , just characters, and we want to convert that to a single string.
So essentially we want to convert this:
to this:
It is the same as every method we read above. We can apply any of those 4 methods to convert the list of characters to a string. Except, since here the list of characters forms a single word, we would not want any spaces between each character. So when we use the join function, instead of adding the space as separator, we simply leave the quotes blank.
Here's how each of them will look:
Using join
Output:
Traversing the list
Output:
Using map() function
List comprehension
The output for every method will still be the same - "hello".
How to Write a List of Items into a Text or CSV File?
Using the methods we read about above, we can even write data (lists) to text or CSV files. You can read the scaler article on Python Writing Files.
As mentioned in the article linked above as well, python offers functions called - write() and writelines() to write into files. Remember that the file must be in w, w+, a, or a+ modes.
To write into a file, you must first open it, and then write into it, and close it. To write into the file, we're going to select the fastest among the 4 methods we discussed above - list comprehension.
Now, when you check the contents of the file, this is what it contains:
In the code above we opened a txt file to write into, you can change the extension and open any file you desire, whether csv, txt or any other.
Instead of writing into the file in a single line, you can also use "n" which tells the program to break after each item in your list.
So the file.write statement looks like this:
And the contents of your file will look like this:
Now you know everything there is to converting a list to a string in Python!
Conclusion
- A list is a mutable data type in python which is used to store items. The items stored in the lists can be of different data types, unlike in other programming languages.
- Strings are an immutable data type. They are indexed just like lists, starting from 0.
- Any element of a string or a list can be accessed like this : list[0] for the first item in the list, and like this : string[3] for the fourth element in the list.
- To convert a list to a string, you can use four methods :
- join() function
- Traversing the list
- Using the map() function
- List comprehension
- Of these 4, list comprehension is the fastest method.
- You can use any of the above mentioned methods to convert a list of characters to a string, by removing the space separator from the join function.
- To write the contents of a list into a file, python's file_obj.write() function is used. Inside the write() function, you can use any of the methods listed.